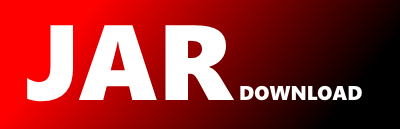
fr.lirmm.boreal.util.externalHaltingConditions.ExternalHaltingCondition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of integraal-util Show documentation
Show all versions of integraal-util Show documentation
Util objects for integraal
package fr.lirmm.boreal.util.externalHaltingConditions;
import java.time.Duration;
import java.util.Objects;
import fr.lirmm.boreal.util.keywords.InteGraalKeywords.Algorithms.ExternalHaltingConditions;
/**
* Represents a pair of halting condition: a keyword and its associated value.
*
* @param keyword the keyword representing the condition.
* @param value the value of the condition.
*/
public record ExternalHaltingCondition(ExternalHaltingConditions keyword, Object value) {
/**
* Custom constructor that enforces type constraints based on the keyword.
*
* @param keyword the halting condition keyword.
* @param value the value associated with the keyword.
*
* @throws IllegalArgumentException if the value cannot be cast to Long or
* Duration, depending on the keyword.
*/
public ExternalHaltingCondition {
Objects.requireNonNull(keyword);
Objects.requireNonNull(value);
switch (keyword) {
case RANK -> {
if (!isValidNonNegativeLong(value)) {
throw new IllegalArgumentException("Value for " + keyword + " must be convertible to Long and be non-negative");
}
}
case TIMEOUT -> {
if (!isValidDuration(value)) {
throw new IllegalArgumentException("Value for " + keyword + " must be convertible to Duration");
}
}
case null -> throw new IllegalArgumentException("Keyword cannot be null");
default -> throw new IllegalArgumentException("Unsupported keyword: " + keyword);
}
}
/**
* Returns the value cast to the correct type based on the keyword.
*
* @param The expected type of the value.
* @return The value cast to the specified type.
*
* @throws ClassCastException if the value cannot be cast to the expected type.
*/
@SuppressWarnings("unchecked")
public T getValue() {
switch (keyword) {
case RANK -> {
return (T) Long.valueOf(value.toString());
}
case TIMEOUT -> {
return (T) Duration.parse(value.toString());
}
default -> throw new IllegalArgumentException("Unsupported keyword: " + keyword);
}
}
/**
* Helper method to check if the value can be converted to a Long using a
* try-catch. It also checks if the value is positive.
*
* @param value the value to validate.
* @return true if the value can be cast to Long, false otherwise.
*/
private static boolean isValidNonNegativeLong(Object value) {
try {
return (Long.valueOf(value.toString()) >= 0 ? true : false);
} catch (NumberFormatException e) {
return false;
}
}
/**
* Helper method to check if the value can be converted to Duration using a
* try-catch.
*
* @param value the value to validate.
* @return true if the value can be cast to Duration, false otherwise.
*/
private static boolean isValidDuration(Object value) {
try {
Duration.parse(value.toString());
return true;
} catch (Exception e) {
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy