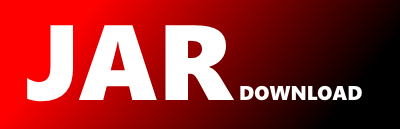
fr.lirmm.boreal.util.keywords.InteGraalKeywords Maven / Gradle / Ivy
Show all versions of integraal-util Show documentation
package fr.lirmm.boreal.util.keywords;
import java.time.Duration;
import java.time.format.DateTimeParseException;
import java.util.Optional;
import fr.lirmm.boreal.util.enumerations.EnumUtils;
/**
* The InteGraalKeywords enum provides a set of keywords and categorizations
* that defines the realm of possibilities with InteGraal. These include:
*
*
* - Algorithms: This section defines various algorithms for executing
* key operations within InteGraal, e.g., Knowledge Base Chase,
* Ontology-Mediated Query Rewriting, Rule Compilation, and Query
* Answering.
*
* - For each algorithm, it also includes the set of its supported
* Parameters.
*
* - Finally, it includes options for the algorithms' result options, such as
* Answer Types (e.g., list vs count-based results).
*
* - Internal Storage Configuration: This section outlines the
* parameters for setting the storage of a factbase in InteGraal.
*
* - Supported File Extensions: Defines the various file formats
* supported by the system, notably formats for factbases, query bases, rule
* bases, and mapping bases.
*
* - Monitoring Operations: Specifies key operations that can be
* monitored within the system, such as loading factbases, rulebases, and query
* workloads, or running a specific algorithm.
*
*
* This enumeration is meant to represent all features of InteGraal and is
* primarily (but not only) used by the component builder.
*
* @author Federico Ulliana, Michel Leclère
*/
public enum InteGraalKeywords {
/**
* Closed parameters
*/
ALGORITHMS(Algorithms.class),
ANSWERS(Algorithms.Answers.class),
IMAGES(Algorithms.Images.class),
COMPILATION(Algorithms.Parameters.Compilation.class),
SCHEDULER(Algorithms.Parameters.Chase.Scheduler.class),
EVALUATOR(Algorithms.Parameters.Chase.Evaluator.class),
APPLIER(Algorithms.Parameters.Chase.Applier.class),
TRANFORMER(Algorithms.Parameters.Chase.Transformer.class),
COMPUTER(Algorithms.Parameters.Chase.Computer.class),
CHECKER(Algorithms.Parameters.Chase.Checker.class),
APPLICATION(Algorithms.Parameters.Chase.Application.class),
SKOLEM(Algorithms.Parameters.Chase.Skolem.class),
HYBRIDTYPES(Algorithms.Parameters.HybridTypes.class),
DBTYPE(InternalStorageConfiguration.DBType.class),
DRIVERTYPE(InternalStorageConfiguration.DriverType.class),
DBMSDRIVERPARAMETERS(InternalStorageConfiguration.DBMSDriverParameters.class),
STORAGELAYOUT(InternalStorageConfiguration.StorageLayout.class),
FACTBASE(SupportedFileExtensions.Factbase.class),
QUERYBASE(SupportedFileExtensions.Querybase.class),
RULEBASE(SupportedFileExtensions.Rulebase.class),
MAPPINGBASE(SupportedFileExtensions.Mappingbase.class),
MONITORINGOPERATIONS(MonitoringOperations.class),
/**
* Open parameters
*/
MAX(Integer.class), // needs a positive integer, default 0 (means no limit)
RANK(Integer.class), // needs a positive integer, default 0 (means no limit)
TIMEOUT(Duration.class), // needs a duration, default 0 (means no TIMEOUT)
URL(String.class, DBMSDRIVERPARAMETERS),
PORT(String.class, DBMSDRIVERPARAMETERS),
DATABASE_NAME(String.class, DBMSDRIVERPARAMETERS),
USER_NAME(String.class, DBMSDRIVERPARAMETERS),
USER_PASSWORD(String.class, DBMSDRIVERPARAMETERS),
CLEAR_DB(String.class, DBMSDRIVERPARAMETERS);
// TODO Les paramètres peuvent-ils être partagés par les différents services ?
// Comment indiquer les valeurs autorisés d'un paramètre (cf. code ci-après) ?
// Les valeurs par défaut des paramètres sont elles les mêmes pour les différents services ?
// Comment connaitre les paramètres permis par un service ?
// Qui vérifie la cohérence d'un paramètre ?
// Qui vérifier la validité d'une séquence de paramètres pour un service ?
// Que fait-on si le même paramètre est donné deux fois pour un service alors que
// le service n'accepte qu'une valeur ? (notion de paramètre cumulatif ou non)
// La dernière valeur passée est celle prise en compte ?
// Ne devrions nous pas avoir une enum des services ?
private final Class> type;
private final Optional parentEnum;
private final boolean isEnumeration;
/**
* Constructor for InteGraalKeywords.
*
* @param type The class type associated with the keyword.
*/
private InteGraalKeywords(Class> type) {
this.type = type;
this.parentEnum = Optional.empty();
this.isEnumeration = Enum.class.isAssignableFrom(type);
}
/**
* Constructor for InteGraalKeywords which represent parameters with open values.
* Such parameters must be declared by an existing enumeration.
*
* @param type The class type associated with the keyword.
* @param parent The InteGraalKeyword defining this parameter.
*/
private InteGraalKeywords(Class> type, InteGraalKeywords parent) {
this.type = type;
this.parentEnum = Optional.of(parent);
this.isEnumeration = Enum.class.isAssignableFrom(type);
// sanity check
checkAndGetEnumerationValue(this.name(),parent);
}
/**
* Gets the class type associated with the keyword.
*
* @return The class type.
*/
public Class> getType() {
return this.type;
}
/**
* @return A possibly empty optional containing the parent enumeration.
*/
public Optional getParentEnum() {
return this.parentEnum;
}
/**
* True iff the keyword values are defined by an enumeration
*
* @return The class type.
*/
public boolean isEnumeration() {
return isEnumeration;
}
/**
* Finds the keyword associated with the given string.
*
* @param s The string representation of the keyword.
* @return The corresponding InteGraalKeywords enum.
* @throws IllegalArgumentException If the keyword is unknown.
*/
public static InteGraalKeywords findKeyword(String s) {
InteGraalKeywords ikw = (InteGraalKeywords) EnumUtils.findConstantInEnumeration(s, InteGraalKeywords.class);
if (ikw == null)
throw new IllegalArgumentException(ikw + " is an unknown InteGraalKeywords.");
return ikw;
}
/**
* Finds the value associated with the keyword from the given string.
*
* @param s The string representation of the value.
* @return The corresponding value.
* @throws IllegalArgumentException If the value is invalid for the keyword.
*/
public Object findValue(String s) {
if (this.isEnumeration()) {
return checkAndGetEnumerationValue(s, this);
} else {
return getValueType(s, this);
}
}
public static Object checkAndGetEnumerationValue(String s, InteGraalKeywords type) {
Enum> val =
EnumUtils.findConstantInEnumeration(s, (Class extends Enum>>) type.getType());
if (val == null) {
String[] expectedValues = EnumUtils.getAllConstantsAsString(type.getDeclaringClass());
throw new IllegalArgumentException(val + " is not a valid value for " + type.name() + " InteGraalKeywords. It must be one among:" + expectedValues);
} else
return val;
}
public static void checkValueType(Object s, InteGraalKeywords type) {
if (!type.getType().equals(s.getClass())) {
throw new RuntimeException("Wrong type : " + s.getClass() + " for type " + type);
}
}
public static Object getValueType(String s, InteGraalKeywords inteGraalKeyword) {
if (inteGraalKeyword.getType().equals(String.class)) {
return s;
}
if (inteGraalKeyword.getType().equals(Integer.class)) { // we assume here that Integer parameter type means positive integers.
try {
Integer i = Integer.parseUnsignedInt(s);
if (i >= 0) return i;
} catch (NumberFormatException e) {
throw new IllegalArgumentException(s + " is not a valid value for " + inteGraalKeyword.name() + " InteGraalKeywords. It must be a positive integer.");
}
}
if (inteGraalKeyword.getType().equals(Duration.class)) {
try {
return Duration.parse(s);
} catch (DateTimeParseException e) {
throw new IllegalArgumentException(s + " is not a valid value for " + inteGraalKeyword.name() + " InteGraalKeywords. It must be a positive integer.");
}
}
throw new RuntimeException("Unsupported type : " + s.getClass() + " for type");
}
/**
* Defines various algorithms for InteGraal's operations.
*/
public enum Algorithms {
/**
* Knowledge Base Chase
*/
KB_CHASE,
/**
* Ontology-Mediated Query Rewriting
*/
OMQ_REWRITING,
/**
* Rule Compilation
*/
RULE_COMPILATION,
/**
* Query Answering
*/
QUERY_ANSWERING,
/**
* Ontology-Mediated Query Answering via Chase
*/
OMQA_CHASE,
/**
* Ontology-Mediated Query Answering via Rewriting
*/
OMQA_REW,
/**
* Query Answering via Hybrid Strategy
*/
QUERY_ANSWERING_VIA_HYBRID_STRATEGY,
/**
* Query Explanation
*/
QUERY_EXPLANATION,
/**
* CSV Rules Encoding
*/
CSV_RLS_ENCODING;
/**
* Specifies types of answers in InteGraal algorithms.
*/
public enum Answers {
/**
* Provides a list of answers
*/
LIST,
/**
* Provides a set of answers
*/
SET,
/**
* Provides only the count of answers
*/
COUNT_ONLY
}
/**
* Specifies types of images for running an evaluation in InteGraal.
*/
public enum Images {
/**
* Only constant (and literal) terms in answers
*/
CONSTANT,
/**
* all terms (including variables) in answers
*/
ALL
}
/**
* Specifies the algorithm parameters.
*/
public enum Parameters {
/**
* Represents the algorithm parameter keyword
*/
ALGORITHM_PARAMETERS;
/**
* Defines compilation strategies.
*/
public enum Compilation {
/**
* No Compilation
*/
NO_COMPILATION,
/**
* Identifier Compilation
*/
ID_COMPILATION,
/**
* Hierarchical Compilation
*/
HIERARCHICAL_COMPILATION
}
/**
* Defines the different strategies for running a chase in InteGraal.
*/
public enum Chase {
/**
* Represents the chase keyword.
*/
CHASE;
/**
* Specifies different scheduler strategies for chase.
*/
public enum Scheduler {
/**
* Naive Scheduler
*/
NAIVE_SCHEDULER,
/**
* GRD Scheduler
*/
GRD
}
/**
* Specifies different evaluator strategies for chase.
*/
public enum Evaluator {
/**
* Generic Evaluator
*/
GENERIC,
/**
* Smart Evaluator
*/
SMART
}
/**
* Specifies different trigger applier strategies for chase.
*/
public enum Applier {
/**
* Breadth-First Trigger Applier
*/
BREADTH_FIRST_TRIGGER,
/**
* Parallel Trigger Applier
*/
PARALLEL_TRIGGER,
/**
* Source Delegated Datalog Applier
*/
SOURCE_DELEGATED_DATALOG
}
/**
* Specifies different transformation strategies for chase.
*/
public enum Transformer {
/**
* Apply all transformations
*/
ALL,
/**
* Apply frontier transformations
*/
FRONTIER
}
/**
* Specifies different computation strategies for chase.
*/
public enum Computer {
/**
* Naive Computer
*/
NAIVE_COMPUTER,
/**
* Semi-Naive Computer
*/
SEMI_NAIVE,
/**
* Two-Step Computer
*/
TWO_STEP
}
/**
* Specifies different rule-checking strategies for chase.
*/
public enum Checker {
/**
* True Checker
*/
TRUE,
/**
* Oblivious Checker
*/
OBLIVIOUS,
/**
* Semi-Oblivious Checker
*/
SEMI_OBLIVIOUS,
/**
* Restricted Checker
*/
RESTRICTED,
/**
* Equivalent Checker
*/
EQUIVALENT
}
/**
* Specifies different application methods for chase.
*/
public enum Application {
/**
* Direct Application
*/
DIRECT,
/**
* Parallel Application
*/
PARALLEL
}
/**
* Specifies different Skolem function strategies.
*/
public enum Skolem {
/**
* Fresh Skolem
*/
FRESH,
/**
* Body-based Skolem
*/
BODY,
/**
* Frontier-based Skolem
*/
FRONTIER,
/**
* Frontier Piece-based Skolem
*/
FRONTIER_PIECE
}
}
/**
* Specifies hybrid reasoning types.
*/
public enum HybridTypes {
/**
* Max FES Hybrid Type
*/
MAX_FES,
/**
* Max FUS Hybrid Type
*/
MAX_FUS
}
}
/**
* Specifies external halting conditions
*/
public enum ExternalHaltingConditions {
/**
* Max depth of reasoning (algorithm dependent)
*/
RANK,
/**
* Time to finish the task
*/
TIMEOUT;
}
}
/**
* Defines storage-related keywords for InteGraal.
*/
public enum InternalStorageConfiguration {
/**
* Represents the storage keyword.
*/
STORAGE;
/**
* Defines different database types supported.
*/
public enum DBType {
/**
* SQL database
*/
SQL,
/**
* SPARQL endpoint
*/
SPARQL,
/**
* Simple In-Memory Graph Store
*/
SimpleInMemoryGraphStore,
/**
* Default In-Memory Atom Set
*/
DefaultInMemoryAtomSet,
/**
* Simple FO Formula Store
*/
SimpleFOFormulaStore
}
/**
* Defines different driver types supported by InteGraal for internal storage.
*/
public enum DriverType {
/**
* HSQLDB driver
*/
HSQLDB,
/**
* MySQL driver
*/
MySQL,
/**
* PostgreSQL driver
*/
PostgreSQL,
/**
* SQLite driver
*/
SQLite
}
/**
* Parameters for database management system drivers.
*/
public enum DBMSDriverParameters {
/**
* Database URL
*/
URL,
/**
* Database Port
*/
PORT,
/**
* Name of the Database
*/
DATABASE_NAME,
/**
* User Name
*/
USER_NAME,
/**
* User Password
*/
USER_PASSWORD,
/**
* Flag to reset the database after opening the connection
*/
CLEAR_DB
}
/**
* Storage layouts for the database.
*/
public enum StorageLayout {
/**
* Ad-hoc SQL layout : property tables
*/
AdHocSQL,
/**
* Encoded Ad-hoc SQL layout : property tables with integer encoding
*/
EncodingAdHocSQL
}
}
/**
* Specifies different file extensions supported by InteGraal.
*/
public enum SupportedFileExtensions {
/**
* Represents DLGP file extension.
*/
DLGP;
/**
* Specifies supported file extensions for factbases.
*/
public enum Factbase {
/**
* DLGP format
*/
DLGP,
/**
* DLGPE format
*/
DLGPE,
/**
* RDF format
*/
RDF,
/**
* CSV format
*/
CSV,
/**
* RLS format
*/
RLS
}
/**
* Specifies supported file extensions for query bases.
*/
public enum Querybase {
/**
* DLGP format
*/
DLGP,
/**
* DLGPE format
*/
DLGPE;
}
/**
* Specifies supported file extensions for rule bases.
*/
public enum Rulebase {
/**
* DLGP format
*/
DLGP,
/**
* OWL format
*/
OWL,
/**
* DLGPE format
*/
DLGPE;
}
/**
* Specifies supported file extensions for mapping bases.
*/
public enum Mappingbase {
/**
* JSON format
*/
JSON,
/**
* VD is a JSON format for defining views
*/
VD
}
}
/**
* Defines various operations in InteGraal.
*/
public enum MonitoringOperations {
/**
* Load Factbase Operation
*/
LOAD_FACTBASE,
/**
* Load Rulebase Operation
*/
LOAD_RULEBASE,
/**
* Load Query Workload Operation
*/
LOAD_QUERY_WORKLOAD,
/**
* Execute a chase
*/
KBCHASE_EXECUTION,
/**
* Execute a rewriting
*/
COMPUTING_UFOQ_REWRITING_EXECUTION,
/**
* Evaluate a query
*/
QUERY_EVALUATION
}
}