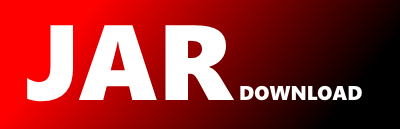
fr.lteconsulting.hexa.client.datatable.DataTableTools Maven / Gradle / Ivy
The newest version!
package fr.lteconsulting.hexa.client.datatable;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import com.google.gwt.core.shared.GWT;
import fr.lteconsulting.hexa.client.interfaces.IHasIntegerId;
import fr.lteconsulting.hexa.client.tools.Func1;
/**
* Tools for DataTable
*
*/
public class DataTableTools
{
/**
* Inserts a flat list as a tree in a TableCollectionManager. It does
* insert data in the right order for the creation of the tree (parents
* must be inserted first)
*
* @param list
* @param mng
* @param parentIdGetter
*/
public static void insertFlatList( List list, TableCollectionManager mng, Func1 parentIdGetter )
{
HashSet inserted = new HashSet<>();
List toInsert = new ArrayList<>( list );
List postPoned = new ArrayList<>();
List inOrder = new ArrayList<>();
int nbInserted;
while( ! toInsert.isEmpty() )
{
nbInserted = 0;
while( ! toInsert.isEmpty() )
{
T a = toInsert.remove( 0 );
Integer parentId = parentIdGetter.exec( a );
if( parentId==null || parentId<=0 || inserted.contains( parentId ) || mng.getRowForRecordId( parentId )!=null )
{
inOrder.add( a );
inserted.add( a.getId() );
nbInserted++;
}
else
{
postPoned.add( a );
}
}
toInsert = postPoned;
postPoned = new ArrayList<>();
if( nbInserted == 0 && ! toInsert.isEmpty() )
{
GWT.log("Cannot construct full tree !");
throw new RuntimeException( "Cannot construct full tree !" );
}
}
for( T t : inOrder )
mng.getDataPlug().updated( t );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy