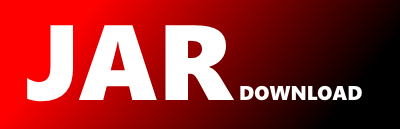
fr.lteconsulting.hexa.client.tools.HierarchySet Maven / Gradle / Ivy
The newest version!
package fr.lteconsulting.hexa.client.tools;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map.Entry;
import java.util.Set;
import fr.lteconsulting.hexa.client.ui.treetable.Row;
import fr.lteconsulting.hexa.client.ui.treetable.TreeTable;
public class HierarchySet
{
public interface IHierarchyLevel
{
public String getName();
public String getIdentifier( T record );
public void fillRow( Row row, T record );
public IHierarchyAccumulator getNewAccumulator();
}
ArrayList> hierarchy = new ArrayList>();
public interface IHierarchyAccumulator
{
public void add( T element );
public void fillRow( Row row );
}
HashMap items = new HashMap();
HashMap> accumulators = new HashMap>();
public void resetDisplay()
{
items.clear();
accumulators.clear();
}
public void clearHierarchy()
{
hierarchy.clear();
}
public void add( IHierarchyLevel item )
{
hierarchy.add( item );
}
public Row getParentItem( TreeTable table, T element )
{
Row itemParent = null;
String itemAddress = "";
for( IHierarchyLevel h : hierarchy )
{
itemAddress += "-" + h.getIdentifier( element );
Row item = items.get( itemAddress );
if( item == null )
{
item = table.addRow( itemParent );
h.fillRow( item, element );
// TODO : FIx the bug that prevents to do that...
// table.setExpanded( item, false );
items.put( itemAddress, item );
accumulators.put( item, h.getNewAccumulator() );
}
IHierarchyAccumulator accu = accumulators.get( item );
if( accu != null )
accu.add( element );
itemParent = item;
}
return itemParent;
}
public Row createItem( TreeTable table, T element )
{
Row itemParent = getParentItem( table, element );
return table.addRow( itemParent );
}
public void displayAccumulators( TreeTable table )
{
Set>> set = accumulators.entrySet();
for( Iterator>> i = set.iterator(); i.hasNext(); )
{
Entry> entry = i.next();
if( entry.getValue() != null )
entry.getValue().fillRow( entry.getKey() );
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy