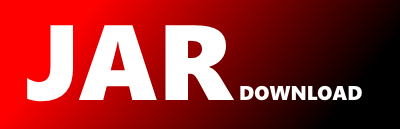
fr.lteconsulting.hexa.server.qpath.DatabaseDescription Maven / Gradle / Ivy
The newest version!
package fr.lteconsulting.hexa.server.qpath;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
public class DatabaseDescription
{
public class TableDescription
{
public String name;
HashMap fields = new HashMap();
HashMap> unicityConstraints = new HashMap>();
public TableDescription( String name )
{
this.name = name;
}
public FieldDescription addField( String name, String type, String canNull, String defaultValue, String extra, String key )
{
FieldDescription fieldDesc = new FieldDescription( name, type, canNull, defaultValue, extra, key );
fields.put( fieldDesc.name, fieldDesc );
return fieldDesc;
}
public List addUnicityConstraint( String name )
{
List columnNames = new ArrayList();
unicityConstraints.put( name, columnNames );
return columnNames;
}
}
public class FieldDescription
{
public String name;
public String type;
public String canNull;
public String defaultValue;
public String extra;
public String key;
public boolean primaryKey;
public boolean uniqueKey;
public boolean multipleIndex;
public String comment;
ArrayList fieldReferences = new ArrayList();
public FieldDescription( String name, String type, String canNull, String defaultValue, String extra, String key )
{
this.name = name;
this.type = type;
this.canNull = canNull;
this.defaultValue = defaultValue;
this.extra = extra;
this.key = key;
if( key.equalsIgnoreCase( "PRI" ) )
primaryKey = true;
else if( key.equalsIgnoreCase( "UNI" ) )
uniqueKey = true;
else if( key.equalsIgnoreCase( "MUL" ) )
multipleIndex = true;
}
public void addReferenceField( String table, String field, String constraintName )
{
FieldReference referenceToAdd = new FieldReference( table, field );
// skip if already declared
for( FieldReference ref : fieldReferences )
{
if( ref.equals( referenceToAdd ) )
{
ref.addConstraintName( constraintName );
return;
}
}
referenceToAdd.addConstraintName( constraintName );
fieldReferences.add( referenceToAdd );
}
public boolean hasReference( String table, String field )
{
for( FieldReference ref : fieldReferences )
{
if( ref.table.equalsIgnoreCase( table ) && ref.field.equalsIgnoreCase( field ) )
return true;
}
return false;
}
}
public class FieldReference
{
public String table;
public String field;
HashSet constraintNames = new HashSet();
public FieldReference( String table, String field )
{
this.table = table;
this.field = field;
}
public void addConstraintName( String constraintName )
{
constraintNames.add( constraintName );
}
public boolean equals( FieldReference other )
{
return table.equalsIgnoreCase( other.table ) && field.equalsIgnoreCase( other.field );
}
}
public HashMap tables = new HashMap();
public String name;
public DatabaseDescription( String name )
{
this.name = name;
}
public TableDescription addTable( String tableName )
{
TableDescription tableDesc = new TableDescription( tableName );
tables.put( tableDesc.name, tableDesc );
return tableDesc;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy