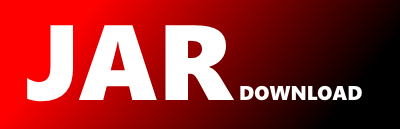
fr.vergne.downhill.impl.ReuseDownhillCollider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of downhill-core Show documentation
Show all versions of downhill-core Show documentation
Implementation of the downhill collider algorithm.
The newest version!
package fr.vergne.downhill.impl;
import java.util.Collection;
import java.util.HashSet;
import java.util.LinkedList;
import fr.vergne.downhill.DownhillCollider;
/**
* The {@link ReuseDownhillCollider} aims at considering all the possible
* combinations of {@link Ball}s to create new ones. Thus, each {@link Ball} is
* re-used at each iteration, and the process stops when no new {@link Ball} can
* be created. At the end of the colliding, the original {@link Ball}s are
* returned with the new ones (including the intermediaries) unless the opposite
* is asked.
*
* @author Matthieu Vergne
*
* @param
*/
public class ReuseDownhillCollider implements DownhillCollider {
private final boolean isConsumedKept;
/**
* Create an instance of {@link ReuseDownhillCollider}. The argument allows
* to tell which {@link Ball}s to return: if set to true
, all
* the {@link Ball}s should be returned, including the initial ones. If it
* is false
, only the ones which have not been used to create
* new {@link Ball}s should be returned.
*
* Because true
returns the full set of {@link Ball}s and
* false
returns only the final {@link Ball}s, you can get the
* intermediaries (the ones which have been actually used to generate new
* {@link Ball}s) by taking the full set (true
) and removing
* the final set (false
) as well as the initial {@link Ball}s
* (provided at the start).
*
* @param isConsumedKept
* true
if all the {@link Ball}s should be returned,
* false
if only the final ones should be returned
*/
public ReuseDownhillCollider(boolean isConsumedKept) {
this.isConsumedKept = isConsumedKept;
}
public ReuseDownhillCollider() {
this(true);
}
@Override
public Collection rolls(Collection balls,
Collider... colliders) {
Collection oldBalls = new HashSet(balls);
Collection newBalls = oldBalls;
Collection consumed = new HashSet();
do {
Collection merged = new LinkedList();
for (Ball b1 : oldBalls) {
for (Ball b2 : newBalls) {
for (Collider collider : colliders) {
if (collider.areColliding(b1, b2)) {
Ball collided = collider.collide(b1, b2);
if (collided.equals(b1) || collided.equals(b2)) {
// already made, do not consider it
} else {
consumed.add(b1);
consumed.add(b2);
merged.add(collided);
}
} else {
// not able to merge them
}
}
}
}
merged.removeAll(oldBalls);
oldBalls.addAll(newBalls);
newBalls = merged;
} while (!newBalls.isEmpty());
if (isConsumedKept) {
// keep all the balls
} else {
oldBalls.removeAll(consumed);
}
return oldBalls;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy