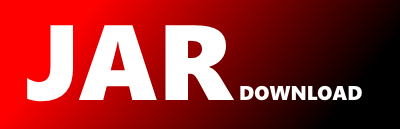
fr.vergne.optimization.TSP.TSP2 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of optimization-samples Show documentation
Show all versions of optimization-samples Show documentation
Optimization algorithms' examples.
The newest version!
package fr.vergne.optimization.TSP;
import java.io.IOException;
import java.util.Collection;
import java.util.LinkedList;
import java.util.List;
import java.util.Random;
import fr.vergne.optimization.TSP.path.Location;
import fr.vergne.optimization.TSP.path.Path2;
import fr.vergne.optimization.generator.Explorator;
import fr.vergne.optimization.generator.Mutator;
public class TSP2 extends AbstractTSP {
private final static Random rand = new Random();
private final List references;
public static void main(String[] args) throws IOException {
new TSP2().run();
}
public TSP2() {
super();
this.references = new LinkedList(getLocations());
}
@Override
protected PathIncubator getIncubator(Collection locations) {
PathIncubator incubator = new PathIncubator();
for (Explorator explorator : getExplorators(locations)) {
incubator.addExplorator(explorator);
}
for (Mutator mutator : getMutators(locations)) {
incubator.addMutator(mutator);
}
return incubator;
}
protected Collection> getMutators(
Collection locations) {
Collection> mutators = new LinkedList>();
mutators.add(new Mutator() {
@Override
public String toString() {
return "rebranch";
}
@Override
public boolean isApplicableOn(Path2 original) {
return true;
}
@Override
public Path2 generates(Path2 original) {
List genes = original.getIndexes();
int index = rand.nextInt(genes.size() - 1);
int newValue = rand.nextInt(genes.size() - index - 1);
List newGenes = new LinkedList(genes);
newGenes.set(index, newValue);
return new Path2(references, newGenes);
}
});
return mutators;
}
protected Collection> getExplorators(
final Collection locations) {
Collection> explorators = new LinkedList>();
explorators.add(new Explorator() {
@Override
public String toString() {
return "random";
}
@Override
public boolean isApplicableOn(Collection configuration) {
return true;
}
@Override
public Path2 generates(Collection population) {
List genes = new LinkedList();
for (int i = references.size() - 1; i > 0; i--) {
genes.add(rand.nextInt(i));
}
Path2 path = new Path2(references, genes);
System.out.println("Random: " + path);
return path;
}
});
explorators.add(new Explorator() {
@Override
public String toString() {
return "combinator";
}
@Override
public boolean isApplicableOn(Collection population) {
return population.size() > 1;
}
@Override
public Path2 generates(Collection population) {
List remaining = new LinkedList(population);
Path2 parent1 = remaining.remove(rand.nextInt(remaining.size()));
Path2 parent2 = remaining.remove(rand.nextInt(remaining.size()));
List genes1 = parent1.getIndexes();
List genes2 = parent2.getIndexes();
List newGenes = new LinkedList();
for (int i = 0; i < references.size() - 1; i++) {
newGenes.add(rand.nextBoolean() ? genes1.get(i) : genes2
.get(i));
}
Path2 child = new Path2(references, newGenes);
System.out.println("Combinator: " + child);
return child;
}
});
return explorators;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy