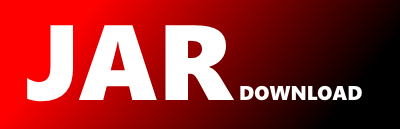
fr.sertelon.media.io.ByteArrayImageInputStream Maven / Gradle / Ivy
Show all versions of jpeg-cmyk Show documentation
/*
* @(#)ByteArrayImageInputStream.java
*
* Copyright (c) 2008-2011 Werner Randelshofer, Immensee, Switzerland.
* All rights reserved.
*
* You may not use, copy or modify this file, except in compliance with the
* license agreement you entered into with Werner Randelshofer.
* For details see accompanying license terms.
*/
package fr.sertelon.media.io;
import java.io.IOException;
import java.nio.ByteOrder;
import javax.imageio.stream.ImageInputStreamImpl;
/**
* A {@code ByteArrayImageInputStream} contains an internal buffer that contains
* bytes that may be read from the stream. An internal counter keeps track of
* the next byte to be supplied by the {@code read} method.
*
* Closing a {@code ByteArrayImageInputStream} has no effect. The methods in
* this class can be called after the stream has been closed without generating
* an {@code IOException}.
*
* @author Werner Randelshofer, Hausmatt 10, CH-6405 Immensee
* @version $Id: ByteArrayImageInputStream.java 134 2011-12-02 16:23:00Z werner
* $
*/
public class ByteArrayImageInputStream extends ImageInputStreamImpl {
/**
* An array of bytes that was provided by the creator of the stream.
* Elements buf[0]
through buf[count-1]
are the
* only bytes that can ever be read from the stream; element
* buf[streamPos]
is the next byte to be read.
*/
protected byte buf[];
/**
* The index one greater than the last valid character in the input stream
* buffer. This value should always be nonnegative and not larger than the
* length of buf
. It is one greater than the position of the
* last byte within buf
that can ever be read from the input
* stream buffer.
*/
protected int count;
/** The offset to the start of the array. */
private final int arrayOffset;
public ByteArrayImageInputStream(byte[] buf) {
this(buf, ByteOrder.BIG_ENDIAN);
}
public ByteArrayImageInputStream(byte[] buf, ByteOrder byteOrder) {
this(buf, 0, buf.length, byteOrder);
}
public ByteArrayImageInputStream(byte[] buf, int offset, int length,
ByteOrder byteOrder) {
this.buf = buf;
this.streamPos = offset;
this.count = Math.min(offset + length, buf.length);
this.arrayOffset = offset;
this.byteOrder = byteOrder;
}
/**
* Reads the next byte of data from this input stream. The value byte is
* returned as an int
in the range 0
to
* 255
. If no byte is available because the end of the stream
* has been reached, the value -1
is returned.
*
* This read
method cannot block.
*
* @return the next byte of data, or -1
if the end of the
* stream has been reached.
*/
@Override
public synchronized int read() {
flushBits();
return (streamPos < count) ? (buf[(int) (streamPos++)] & 0xff) : -1;
}
/**
* Reads up to len
bytes of data into an array of bytes from
* this input stream. If streamPos
equals count
,
* then -1
is returned to indicate end of file. Otherwise, the
* number k
of bytes read is equal to the smaller of
* len
and count-streamPos
. If k
is
* positive, then bytes buf[streamPos]
through
* buf[streamPos+k-1]
are copied into b[off]
* through b[off+k-1]
in the manner performed by
* System.arraycopy
. The value k
is added into
* streamPos
and k
is returned.
*
* This read
method cannot block.
*
* @param b
* the buffer into which the data is read.
* @param off
* the start offset in the destination array b
* @param len
* the maximum number of bytes read.
* @return the total number of bytes read into the buffer, or
* -1
if there is no more data because the end of the
* stream has been reached.
* @exception NullPointerException
* If b
is null
.
* @exception IndexOutOfBoundsException
* If off
is negative, len
is
* negative, or len
is greater than
* b.length - off
*/
@Override
public synchronized int read(byte b[], int off, int len) {
flushBits();
if (b == null) {
throw new NullPointerException();
} else if (off < 0 || len < 0 || len > b.length - off) {
throw new IndexOutOfBoundsException();
}
if (streamPos >= count) {
return -1;
}
if (streamPos + len > count) {
len = (int) (count - streamPos);
}
if (len <= 0) {
return 0;
}
System.arraycopy(buf, (int) streamPos, b, off, len);
streamPos += len;
return len;
}
/**
* Skips n
bytes of input from this input stream. Fewer bytes
* might be skipped if the end of the input stream is reached. The actual
* number k
of bytes to be skipped is equal to the smaller of
* n
and count-streamPos
. The value k
* is added into streamPos
and k
is returned.
*
* @param n
* the number of bytes to be skipped.
* @return the actual number of bytes skipped.
*/
public synchronized long skip(long n) {
if (streamPos + n > count) {
n = count - streamPos;
}
if (n < 0) {
return 0;
}
streamPos += n;
return n;
}
/**
* Returns the number of remaining bytes that can be read (or skipped over)
* from this input stream.
*
* The value returned is count - streamPos
, which is the
* number of bytes remaining to be read from the input buffer.
*
* @return the number of remaining bytes that can be read (or skipped over)
* from this input stream without blocking.
*/
public synchronized int available() {
return (int) (count - streamPos);
}
/**
* Closing a ByteArrayInputStream has no effect. The methods in
* this class can be called after the stream has been closed without
* generating an IOException.
*
*/
@Override
public void close() {
// does nothing!!
}
@Override
public long getStreamPosition() throws IOException {
checkClosed();
return streamPos - arrayOffset;
}
@Override
public void seek(long pos) throws IOException {
checkClosed();
flushBits();
// This test also covers pos < 0
if (pos < flushedPos) {
throw new IndexOutOfBoundsException("pos < flushedPos!");
}
this.streamPos = pos + arrayOffset;
}
private void flushBits() {
bitOffset = 0;
}
@Override
public long length() {
return count - arrayOffset;
}
}