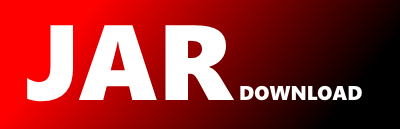
fr.sii.ogham.sms.builder.cloudhopper.DataCodingSchemeBuilder Maven / Gradle / Ivy
Show all versions of ogham-sms-cloudhopper Show documentation
package fr.sii.ogham.sms.builder.cloudhopper;
import java.util.function.Supplier;
import com.cloudhopper.commons.charset.Charset;
import com.cloudhopper.commons.charset.CharsetUtil;
import com.cloudhopper.commons.gsm.DataCoding;
import com.cloudhopper.smpp.SmppConstants;
import fr.sii.ogham.core.builder.Builder;
import fr.sii.ogham.core.builder.configuration.ConfigurationValueBuilder;
import fr.sii.ogham.core.builder.configuration.ConfigurationValueBuilderHelper;
import fr.sii.ogham.core.builder.configurer.Configurer;
import fr.sii.ogham.core.builder.context.BuildContext;
import fr.sii.ogham.core.builder.env.EnvironmentBuilder;
import fr.sii.ogham.core.fluent.AbstractParent;
import fr.sii.ogham.sms.sender.impl.cloudhopper.preparator.CharsetMapToCharacterEncodingGroupDataCodingProvider;
import fr.sii.ogham.sms.sender.impl.cloudhopper.preparator.CharsetMapToGeneralGroupDataCodingProvider;
import fr.sii.ogham.sms.sender.impl.cloudhopper.preparator.DataCodingProvider;
import fr.sii.ogham.sms.sender.impl.cloudhopper.preparator.FirstSupportingDataCodingProvider;
import fr.sii.ogham.sms.sender.impl.cloudhopper.preparator.FixedByteValueDataCodingProvider;
/**
* Data Coding Scheme is a one-octet field in Short Messages (SM) and Cell
* Broadcast Messages (CB) which carries a basic information how the recipient
* handset should process the received message. The information includes:
*
* - the character set or message coding which determines the encoding of the
* message user data
* - the message class which determines to which component of the Mobile
* Station (MS) or User Equipment (UE) should be the message delivered
* - the request to automatically delete the message after reading
* - the state of flags indicating presence of unread voicemail, fax, e-mail
* or other messages
* - the indication that the message content is compressed
* - the language of the cell broadcast message
*
* The field is described in 3GPP 23.040 and 3GPP 23.038 under the name TP-DCS
* (see SMS
* Data Coding Scheme).
*
*
* Configures how Cloudhopper determines the Data Coding Scheme to use:
*
* - Automatic mode:
*
* - If SMPP v3.3 is used then
* {@link CharsetMapToGeneralGroupDataCodingProvider} generates a
* {@link DataCoding} with
* {@link DataCoding#createGeneralGroup(byte, Byte, boolean)}
* - If SMPP v3.4+ is used then
* {@link CharsetMapToCharacterEncodingGroupDataCodingProvider} generates a
* {@link DataCoding} with
* {@link DataCoding#createCharacterEncodingGroup(byte)}
*
*
* - Allow registration of custom {@link DataCodingProvider}
*
*
* @author Aurélien Baudet
*/
public class DataCodingSchemeBuilder extends AbstractParent implements Builder {
private final BuildContext buildContext;
private final Supplier interfaceVersionProvider;
private final ConfigurationValueBuilderHelper autoValueBuilder;
private final ConfigurationValueBuilderHelper dcsValueBuilder;
private DataCodingProvider custom;
/**
* Initializes the builder with a parent builder. The parent builder is used
* when calling {@link #and()} method. The {@link EnvironmentBuilder} is
* used to evaluate properties when {@link #build()} method is called.
*
* @param parent
* the parent builder
* @param buildContext
* for registering instances and property evaluation
* @param interfaceVersionProvider
* A function used to retrieve the value of the interface
* version. This is needed when {@link #auto(Boolean)} mode is
* enabled.
*/
public DataCodingSchemeBuilder(CloudhopperBuilder parent, BuildContext buildContext, Supplier interfaceVersionProvider) {
super(parent);
this.buildContext = buildContext;
this.interfaceVersionProvider = interfaceVersionProvider;
this.autoValueBuilder = buildContext.newConfigurationValueBuilder(this, Boolean.class);
this.dcsValueBuilder = buildContext.newConfigurationValueBuilder(this, Byte.class);
}
/**
* * Enable/disable automatic mode based on SMPP interface version.
*
*
* {@link DataCodingProvider} implementation is selected based on SMPP
* interface version. SMPP v3.3 Data Coding Scheme values are defined in
* SMS
* Data Coding Scheme. SMPP 3.4 introduced a new list of data_coding
* values (PDU
* body).
*
*
* SMPP v3.3
*
* The text message is encoded using {@link Charset}. According to that
* charset, the Data Coding Scheme is determined using the General
* Data Coding group table. Therefore, a simple mapping is applied:
*
* - {@link CharsetUtil#NAME_GSM7} {@literal ->}
* {@link DataCoding#CHAR_ENC_DEFAULT}
* - {@link CharsetUtil#NAME_PACKED_GSM} {@literal ->}
* {@link DataCoding#CHAR_ENC_DEFAULT}
* - {@link CharsetUtil#NAME_GSM} {@literal ->}
* {@link DataCoding#CHAR_ENC_8BIT}
* - {@link CharsetUtil#NAME_GSM8} {@literal ->}
* {@link DataCoding#CHAR_ENC_8BIT}
* - {@link CharsetUtil#NAME_UCS_2} {@literal ->}
* {@link DataCoding#CHAR_ENC_UCS2}
*
*
*
* SMPP v3.4+
*
* The text message is encoded using {@link Charset}. According to that
* charset, the Data Coding Scheme is determined using only the
* Alphabet table. Therefore, a simple mapping is applied:
*
* - {@link CharsetUtil#NAME_GSM7} {@literal ->}
* {@link DataCoding#CHAR_ENC_DEFAULT}
* - {@link CharsetUtil#NAME_PACKED_GSM} {@literal ->}
* {@link DataCoding#CHAR_ENC_DEFAULT}
* - {@link CharsetUtil#NAME_GSM} {@literal ->}
* {@link DataCoding#CHAR_ENC_8BIT}
* - {@link CharsetUtil#NAME_GSM8} {@literal ->}
* {@link DataCoding#CHAR_ENC_8BIT}
* - {@link CharsetUtil#NAME_ISO_8859_1} {@literal ->}
* {@link DataCoding#CHAR_ENC_LATIN1}
* - {@link CharsetUtil#NAME_UCS_2} {@literal ->}
* {@link DataCoding#CHAR_ENC_UCS2}
*
*
*
*
* The value set using this method takes precedence over any property and
* default value configured using {@link #auto()}.
*
*
* .auto(false)
* .auto()
* .properties("${custom.property.high-priority}", "${custom.property.low-priority}")
* .defaultValue(true)
*
*
*
* .auto(false)
* .auto()
* .properties("${custom.property.high-priority}", "${custom.property.low-priority}")
* .defaultValue(true)
*
*
* In both cases, {@code auto(false)} is used.
*
*
* If this method is called several times, only the last value is used.
*
*
* If {@code null} value is set, it is like not setting a value at all. The
* property/default value configuration is applied.
*
* @param enable
* enable or disable automatic Data Coding Scheme detection
* @return this instance for fluent chaining
*/
public DataCodingSchemeBuilder auto(Boolean enable) {
autoValueBuilder.setValue(enable);
return this;
}
/**
* Enable/disable automatic mode based on SMPP interface version.
*
*
* {@link DataCodingProvider} implementation is selected based on SMPP
* interface version. SMPP v3.3 Data Coding Scheme values are defined in
* SMS
* Data Coding Scheme. SMPP 3.4 introduced a new list of data_coding
* values (PDU
* body).
*
*
* SMPP v3.3
*
* The text message is encoded using {@link Charset}. According to that
* charset, the Data Coding Scheme is determined using the General
* Data Coding group table. Therefore, a simple mapping is applied:
*
* - {@link CharsetUtil#NAME_GSM7} {@literal ->}
* {@link DataCoding#CHAR_ENC_DEFAULT}
* - {@link CharsetUtil#NAME_PACKED_GSM} {@literal ->}
* {@link DataCoding#CHAR_ENC_DEFAULT}
* - {@link CharsetUtil#NAME_GSM} {@literal ->}
* {@link DataCoding#CHAR_ENC_8BIT}
* - {@link CharsetUtil#NAME_GSM8} {@literal ->}
* {@link DataCoding#CHAR_ENC_8BIT}
* - {@link CharsetUtil#NAME_UCS_2} {@literal ->}
* {@link DataCoding#CHAR_ENC_UCS2}
*
*
*
* SMPP v3.4+
*
* The text message is encoded using {@link Charset}. According to that
* charset, the Data Coding Scheme is determined using only the
* Alphabet table. Therefore, a simple mapping is applied:
*
* - {@link CharsetUtil#NAME_GSM7} {@literal ->}
* {@link DataCoding#CHAR_ENC_DEFAULT}
* - {@link CharsetUtil#NAME_PACKED_GSM} {@literal ->}
* {@link DataCoding#CHAR_ENC_DEFAULT}
* - {@link CharsetUtil#NAME_GSM} {@literal ->}
* {@link DataCoding#CHAR_ENC_8BIT}
* - {@link CharsetUtil#NAME_GSM8} {@literal ->}
* {@link DataCoding#CHAR_ENC_8BIT}
* - {@link CharsetUtil#NAME_ISO_8859_1} {@literal ->}
* {@link DataCoding#CHAR_ENC_LATIN1}
* - {@link CharsetUtil#NAME_UCS_2} {@literal ->}
* {@link DataCoding#CHAR_ENC_UCS2}
*
*
*
* Custom {@link DataCodingProvider} also configured
*
* If a custom {@link DataCodingProvider} instance is registered, this
* instance is tried first (see {@link #custom(DataCodingProvider)} for more
* information). The automatic behavior is applied after if returned value
* of custom {@link DataCodingProvider} is {@code null}.
*
* Fixed value also configured
*
* If a fixed value is configured then it preempts any other configuration (
* {@link #auto(Boolean)} and {@link #custom(DataCodingProvider)} are not
* used at all).
*
*
*
* This method is mainly used by {@link Configurer}s to register some
* property keys and/or a default value. The aim is to let developer be able
* to externalize its configuration (using system properties, configuration
* file or anything else). If the developer doesn't configure any value for
* the registered properties, the default value is used (if set).
*
*
* .auto()
* .properties("${custom.property.high-priority}", "${custom.property.low-priority}")
* .defaultValue(true)
*
*
*
* Non-null value set using {@link #auto(Boolean)} takes precedence over
* property values and default value.
*
*
* .auto(false)
* .auto()
* .properties("${custom.property.high-priority}", "${custom.property.low-priority}")
* .defaultValue(true)
*
*
* The value {@code false} is used regardless of the value of the properties
* and default value.
*
*
* See {@link ConfigurationValueBuilder} for more information.
*
*
* @return the builder to configure property keys/default value
*/
public ConfigurationValueBuilder auto() {
return autoValueBuilder;
}
/**
* Use the same Data Coding Scheme value for all messages.
*
*
* The value set using this method takes precedence over any property and
* default value configured using {@link #value()}.
*
*
* .value((byte) 0x10)
* .value()
* .properties("${custom.property.high-priority}", "${custom.property.low-priority}")
* .defaultValue((byte) 0)
*
*
*
* .value((byte) 0x10)
* .value()
* .properties("${custom.property.high-priority}", "${custom.property.low-priority}")
* .defaultValue((byte) 0)
*
*
* In both cases, {@code value((byte) 0x10)} is used.
*
*
* If this method is called several times, only the last value is used.
*
*
* If {@code null} value is set, it is like not setting a value at all. The
* property/default value configuration is applied.
*
* @param value
* the Data Coding Scheme value for all messages
* @return this instance for fluent chaining
*/
public DataCodingSchemeBuilder value(Byte value) {
this.dcsValueBuilder.setValue(value);
return this;
}
/**
* Use the same Data Coding Scheme value for all messages.
*
*
* This method is mainly used by {@link Configurer}s to register some
* property keys and/or a default value. The aim is to let developer be able
* to externalize its configuration (using system properties, configuration
* file or anything else). If the developer doesn't configure any value for
* the registered properties, the default value is used (if set).
*
*
* .value()
* .properties("${custom.property.high-priority}", "${custom.property.low-priority}")
* .defaultValue((byte) 0)
*
*
*
* Non-null value set using {@link #value(Byte)} takes precedence over
* property values and default value.
*
*
* .value((byte) 0x10)
* .value()
* .properties("${custom.property.high-priority}", "${custom.property.low-priority}")
* .defaultValue((byte) 0)
*
*
* The value {@code (byte) 0x10} is used regardless of the value of the
* properties and default value.
*
*
* See {@link ConfigurationValueBuilder} for more information.
*
*
* @return the builder to configure property keys/default value
*/
public ConfigurationValueBuilder value() {
return dcsValueBuilder;
}
/**
* Register a custom strategy to determine Data Coding Scheme value.
*
*
* Automatic behavior (see {@link #auto(Boolean)} is still active but custom
* strategy is executed first. As the custom {@link DataCodingProvider} can
* return {@code null}, the automatic behavior is executed in that case.
*
*
* If {@code null} value is provided, custom strategy is disabled.
*
* @param custom
* the Strategy to determine Data Coding Scheme value.
* @return this instance for fluent chaining
*/
public DataCodingSchemeBuilder custom(DataCodingProvider custom) {
this.custom = custom;
return this;
}
@Override
@SuppressWarnings("squid:S5411")
public DataCodingProvider build() {
Byte dataCodingValue = dcsValueBuilder.getValue();
if (dataCodingValue != null) {
return buildContext.register(new FixedByteValueDataCodingProvider(dataCodingValue));
}
FirstSupportingDataCodingProvider firstSupporting = buildContext.register(new FirstSupportingDataCodingProvider());
if (custom != null) {
firstSupporting.register(custom);
}
if (autoValueBuilder.getValue(false)) {
registerAuto(firstSupporting);
}
return firstSupporting;
}
private void registerAuto(FirstSupportingDataCodingProvider firstSupporting) {
Byte interfaceVersion = interfaceVersionProvider.get();
if (interfaceVersion == SmppConstants.VERSION_3_3) {
firstSupporting.register(buildContext.register(new CharsetMapToGeneralGroupDataCodingProvider(false)));
return;
}
// 3.4+
firstSupporting.register(buildContext.register(new CharsetMapToCharacterEncodingGroupDataCodingProvider(false)));
}
}