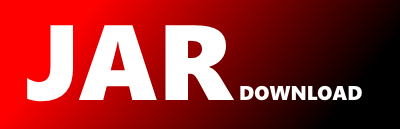
rules.jshint.W001.html Maven / Gradle / Ivy
Show all versions of sonar-web-frontend-js Show documentation
When do I get this error?
The "'hasOwnProperty' is a really bad name" error is thrown when JSHint
encounters an assignment to an object property with the identifier
hasOwnProperty
. This applies to both object literals and to normal
assignment statements. In the following example we define an object with a
property called hasOwnProperty
:
x 1var demo = {
2 hasOwnProperty: 1
3};
4
Why do I get this error?
This error is raised to highlight confusing code that could cause problems in
the future. Your code may run as expected but it's likely to cause issues with
maintenance and be confusing to other developers.
Most objects in JavaScript have access (via inheritance from Object.prototype
)
to the hasOwnProperty
method which is used to check whether a given property
is defined on a given object. This method is commonly used within for...in
loops to ensure only enumerable properties of the object in question are
handled, and not enumerable properties of objects further down its prototype
chain. See the somewhat related "The body of a for in should be wrapped in an
if statement to filter unwanted properties from the prototype" error for
more details:
12 1/*global doSomething */
2var me = {
3 name: "James",
4 age: 23
5};
6
7for (var prop in me) {
8 if (me.hasOwnProperty(prop)) {
9 doSomething(prop);
10 }
11}
12
In that example, were you to add a property hasOwnProperty: 1
to me
, the
guard in the for...in
loop would fail with an error telling you that
hasOwnProperty
is not a function. If the value of your custom hasOwnProperty
is a function then it will be invoked and the code may work but would be very
misleading to anyone else reading.
The solution to this error is to simply not use hasOwnProperty
as a property
identifer. If you are concerned that the value of hasOwnProperty
is no longer
the native function you may want to call the native function in the context of
your object in for...in
loop guards:
13 1/*global doSomething */
2var me = {
3 name: "James",
4 age: 23,
5 hasOwnProperty: 1 // This would cause the previous example to fail
6};
7
8for (var prop in me) {
9 if (Object.hasOwnProperty.call(me, prop)) { // Use the real hasOwnProperty
10 doSomething(prop);
11 }
12}
13
In JSHint 1.0.0 and above you have the ability to ignore any warning with a
special option syntax. The identifier of this
warning is W001. This means you can tell JSHint to not issue this warning
with the /*jshint -W001 */
directive.