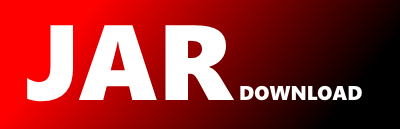
rules.jshint.W076.html Maven / Gradle / Ivy
Show all versions of sonar-web-frontend-js Show documentation
History
This warning has existed in two forms across the three main linters. It was
introduced in the original version of JSLint and has remained (in a way) in all
three tools ever since.
In JSLint and JSHint the warning given is "Unexpected parameter '{a}' in get
{b} function"
In ESLint the message used is the more generic "Unexpected identifier"
The situations that produce the warning have not changed despite changes to the
text of the warning itself.
When do I get this error?
The "Unexpected parameter '{a}' in get {b} function" error, and the
alternative "Unexpected identifier", is thrown when JSLint, JSHint or ESLint
encounters a named parameter in the signature of a property getter function.
In the following example we create an object x with a getter and setter. The
getter will always return half of the set value:
x 1var x = {
2 actual: 10,
3 get x (value) {
4 "use strict";
5 return this.actual / 2;
6 },
7 set x (value) {
8 "use strict";
9 this.actual = value;
10 }
11};
12
Line 2: Redefinition of 'x' from line 0. Line 2: A get function takes no parameters. Line 2: Unexpected space between 'x' and '('. Line 2: Unused 'value'. Line 4: Unexpected 'this'. Line 6: Redefinition of 'x' from line 0. Line 6: Unexpected space between 'x' and '('. Line 8: Unexpected 'this'.
Why do I get this error?
This error is raised to highlight a completely pointless and potentially
confusing piece of code. Your code will run without error if you do not change
it, but could be confusing to other developers and adds unnecessary bytes to the
weight of your script. ECMAScript 5 added new syntax for object property getter
and setter functions. The specification states the following in reference to
getters (ES5 §8.6.1):
The function’s [[Call]] internal method... is called with an empty arguments
list to return the property value each time a get access of the property is
performed.
Since the runtime will never pass any arguments to the getter function, there is
no need to provide any named parameters in the function signature. Simply remove
them to fix the error:
12 1var x = {
2 actual: 10,
3 get x () {
4 "use strict";
5 return this.actual / 2;
6 },
7 set x (value) {
8 "use strict";
9 this.actual = value;
10 }
11};
12
In JSHint 1.0.0 and above you have the ability to ignore any warning with a
special option syntax. The identifier of this warning is W076.
This means you can tell JSHint to not issue this warning with the /*jshint
-W076 */
directive.