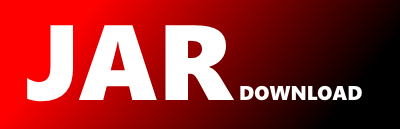
dw.xmlrpc.DokuJClient Maven / Gradle / Ivy
package dw.xmlrpc;
import java.io.File;
import java.io.IOException;
import java.net.MalformedURLException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.logging.Level;
import java.util.logging.Logger;
import dw.xmlrpc.exception.DokuException;
import dw.xmlrpc.exception.DokuIncompatibleVersionException;
import dw.xmlrpc.exception.DokuMethodDoesNotExistsException;
import dw.xmlrpc.exception.DokuMisConfiguredWikiException;
import dw.xmlrpc.exception.DokuNoChangesException;
import dw.xmlrpc.exception.DokuPageDoesNotExistException;
/**
* Main public class to actually make an xmlrpc query
*
* Instantiate one such client for a given wiki and a given user, then make
* xmlrpc query using its methods.
*
* Most methods may throw DokuException because many things can go wrong
* (bad url, wrong credential, no network, unreachable server, ...), so you may
* want to make sure you handle them correcty
*/
public class DokuJClient {
private final CoreClient _client;
private final Locker _locker;
private final Attacher _attacher;
private Logger _logger;
private final String COOKIE_PREFIX = "DW";
/**
* Let override the default Logger
*/
public void setLogger(Logger logger){
_logger = logger;
_client.setLogger(logger);
}
/**
* Instantiate a client for the given user on the given wiki
*
* The wiki should be configured in a way to let this user access the
* xmlrpc interface
*
* @param url Should looks like http[s]://server/mywiki/lib/exe/xmlrpc.php
* @param user Login of the user
* @param password Password of the user
* @throws MalformedURLException
* @throws DokuException
*/
public DokuJClient(String url, String user, String password) throws MalformedURLException, DokuException{
this(url);
loginWithRetry(user, password, 2);
}
/**
* Instantiate a client for an anonymous user on the given wiki
*
* Likely to be unsuitable for most wiki since anonymous user are often
* not authorized to use the xmlrpc interface
*
* @param url Should looks like http[s]://server/mywiki/lib/exe/xmlrpc.php
* @throws MalformedURLException
*/
public DokuJClient(String url) throws MalformedURLException{
this(CoreClientFactory.build(url));
}
public DokuJClient(DokuJClientConfig dokuConfig) throws DokuException{
this(CoreClientFactory.build(dokuConfig));
if ( dokuConfig.user() != null){
loginWithRetry(dokuConfig.user(), dokuConfig.password(), 2);
}
}
private DokuJClient(CoreClient client){
_client = client;
_locker = new Locker(_client);
_attacher = new Attacher(_client);
Logger logger = Logger.getLogger(DokuJClient.class.toString());
setLogger(logger);
}
public boolean hasDokuwikiCookies(){
for(String cookieKey : cookies().keySet()){
if ( cookieKey.startsWith(COOKIE_PREFIX) ){
return true;
}
}
return false;
}
public Map cookies(){
return _client.cookies();
}
//Because it's been observed that some hosting services sometime mess up a bit with cookies...
private void loginWithRetry(String user, String password, int nbMaxRetry) throws DokuException {
boolean success = false;
for(int retry=0 ; retry < nbMaxRetry && !success ; retry++ ){
success = login(user, password);
}
}
public Boolean login(String user, String password) throws DokuException{
Object[] params = new Object[]{user, password};
return (Boolean) genericQuery("dokuwiki.login", params) && hasDokuwikiCookies();
}
/**
* Uploads a file to the wiki
*
* @param attachmentId Id the file should have once uploaded (eg: ns1:ns2:myfile.gif)
* @param localPath The path to the file to upload
* @param overwrite TRUE to overwrite if a file with this id already exist on the wiki
* @throws IOException
* @throws DokuException
*/
public void putAttachment(String attachmentId, String localPath, boolean overwrite) throws IOException, DokuException{
putAttachment(attachmentId, new File(localPath), overwrite);
}
/**
* Uploads a file to the wiki
*
* @param attachmentId Id the file should have once uploaded (eg: ns1:ns2:myfile.gif)
* @param localFile The file to upload
* @param overwrite TRUE to overwrite if a file with this id already exist on the wiki
* @throws IOException
* @throws DokuException
*/
public void putAttachment(String attachmentId, File localFile, boolean overwrite) throws IOException, DokuException{
putAttachment(attachmentId, _attacher.serializeFile(localFile), overwrite);
}
/**
* Uploads a file to the wiki
*
* @param attachmentId Id the file should have once uploaded (eg: ns1:ns2:myfile.gif)
* @param localFile base64 encoded file
* @param overwrite TRUE to overwrite if a file with this id already exist on the wiki
* @throws IOException
* @throws DokuException
*/
public void putAttachment(String attachmentId, byte[] localFile, boolean overwrite) throws DokuException{
_attacher.putAttachment(attachmentId, localFile, overwrite);
}
/**
* Returns information about a media file
*
* @param fileId Id of the file on the wiki (eg: ns1:ns2:myfile.gif)
* @throws DokuException
*/
public AttachmentInfo getAttachmentInfo(String fileId) throws DokuException{
return _attacher.getAttachmentInfo(fileId);
}
/**
* Deletes a file. Fails if the file is still referenced from any page in the wiki.
*
* @param fileId Id of the file on the wiki (eg: ns1:ns2:myfile.gif)
* @throws DokuException
*/
public void deleteAttachment(String fileId) throws DokuException{
_attacher.deleteAttachment(fileId);
}
/**
* Let download a file from the wiki
*
* @param fileId Id of the file on the wiki (eg: ns1:ns2:myfile.gif)
* @param localPath Where to put the file
* @throws DokuException
* @throws IOException
*/
public File getAttachment(String fileId, String localPath) throws DokuException, IOException{
byte[] b = getAttachment(fileId);
File f = new File(localPath);
_attacher.deserializeFile(b, f);
return f;
}
/**
* Let download a file from the wiki
*
* @param fileId Id of the file on the wiki (eg: ns1:ns2:myfile.gif)
* @throws DokuException
* @return the data of the file, encoded in base64
*/
public byte[] getAttachment(String fileId) throws DokuException {
return _attacher.getAttachment(fileId);
}
/**
* Returns information about a list of media files in a given namespace
*
* @param namespace Where to look for files
* @throws DokuException
*/
public List getAttachments(String namespace) throws DokuException{
return getAttachments(namespace, null);
}
/**
* Returns information about a list of media files in a given namespace
*
* @param namespace Where to look for files
* @param additionalParams Potential additional parameters directly sent to Dokuwiki.
* Available parameters are:
* * recursive: TRUE if also files in subnamespaces are to be included, defaults to FALSE
* * pattern: an optional PREG compatible regex which has to match the file id
* @throws DokuException
*/
public List getAttachments(String namespace, Map additionalParams) throws DokuException{
return _attacher.getAttachments(namespace, additionalParams);
}
/**
* Returns a list of recent changed media since given timestamp
* @param timestamp
* @throws DokuException
*/
public List getRecentMediaChanges(Integer timestamp) throws DokuException{
return _attacher.getRecentMediaChanges(timestamp);
}
/**
* Wrapper around {@link #getRecentMediaChanges(Integer)}
* @param date Do not return changes older than this date
*/
public List getRecentMediaChanges(Date date) throws DokuException {
return getRecentMediaChanges((int)(date.getTime() / 1000));
}
/**
* Returns the current time at the remote wiki server as Unix timestamp
* @throws DokuException
*/
public Integer getTime() throws DokuException{
return (Integer) genericQuery("dokuwiki.getTime");
}
/**
* Returns the XML RPC interface version of the remote Wiki.
* This is DokuWiki implementation specific and independent of the supported
* standard API version returned by wiki.getRPCVersionSupported
* @throws DokuException
*/
public Integer getXMLRPCAPIVersion() throws DokuException{
return (Integer) genericQuery("dokuwiki.getXMLRPCAPIVersion");
}
/**
* Returns the DokuWiki version of the remote Wiki
* @throws DokuException
*/
public String getVersion() throws DokuException{
return (String) genericQuery("dokuwiki.getVersion");
}
/**
* Returns the available versions of a Wiki page.
*
* The number of pages in the result is controlled via the "recent" configuration setting of the wiki.
*
* @param pageId Id of the page (eg: ns1:ns2:mypage)
* @throws DokuException
*/
public List getPageVersions(String pageId) throws DokuException {
return getPageVersions(pageId, 0);
}
/**
* Returns the available versions of a Wiki page.
*
* The number of pages in the result is controlled via the recent configuration setting of the wiki.
*
* @param pageId Id of the page (eg: ns1:ns2:mypage)
* @param offset Can be used to list earlier versions in the history.
* @throws DokuException
*/
public List getPageVersions(String pageId, Integer offset) throws DokuException {
boolean useFixForLegacyWiki = false;
if ( offset > 0 ){
if ( getXMLRPCAPIVersion() < 10 ){
useFixForLegacyWiki = true;
offset--;
}
}
Object[] params = new Object[]{pageId, offset};
Object[] result = (Object[]) genericQuery("wiki.getPageVersions", params);
if ( useFixForLegacyWiki && result.length > 1 ){
result = Arrays.copyOfRange(result, 1, result.length);
}
return ObjectConverter.toPageVersion(result, pageId);
}
/**
* Returns the raw Wiki text for a specific revision of a Wiki page.
* @param pageId Id of the page (eg: ns1:ns2:mypage)
* @param timestamp Version of the page
* @throws DokuException
*/
public String getPageVersion(String pageId, Integer timestamp) throws DokuException{
Object[]params = new Object[]{pageId, timestamp};
return (String) genericQuery("wiki.getPageVersion", params);
}
/**
* Lists all pages within a given namespace
* @param namespace Namespace to look for (eg: ns1:ns2)
* @throws DokuException
*/
public List getPagelist(String namespace) throws DokuException {
return getPagelist(namespace, null);
}
/**
* Lists all pages within a given namespace
* @param namespace Namespace to look for (eg: ns1:ns2)
* @param options Options passed directly to dokuwiki's search_all_pages()
* @throws DokuException
*/
public List getPagelist(String namespace, Map options) throws DokuException {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy