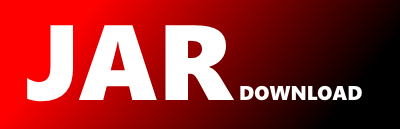
dw.xmlrpc.ObjectConverter Maven / Gradle / Ivy
package dw.xmlrpc;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.Map;
//! @cond
class ObjectConverter {
static List toPageDW(Object[] objs){
List result = new ArrayList();
for(Object o : objs){
result.add(toPageDW(o));
}
return result;
}
static PageDW toPageDW(Object o){
@SuppressWarnings("unchecked")
Map map = (Map) o;
String id = (String) map.get("id");
Integer size = (Integer) map.get("size");
Integer version = (Integer) map.get("rev");
Integer mtime = (Integer) map.get("mtime");
String hash = (String) map.get("hash");
return new PageDW(id, size, version, mtime, hash);
}
static List toSearchResult(Object[] objs){
List result = new ArrayList();
for ( Object o : objs ){
result.add(toSearchResult(o));
}
return result;
}
static SearchResult toSearchResult(Object o){
@SuppressWarnings("unchecked")
Map mapResult = (Map) o;
String id = (String) mapResult.get("id");
String title = (String) mapResult.get("title");
if ( title == null){
//for DW up to 2012-01-25
title = id;
}
Integer rev = (Integer) mapResult.get("rev");
Integer mtime = (Integer) mapResult.get("mtime");
Integer size = (Integer) mapResult.get("size");
Integer score = (Integer) mapResult.get("score");
String snippet = (String) mapResult.get("snippet");
return new SearchResult(id, title, rev, mtime, score, snippet, size);
}
static PageInfo toPageInfo(Object o){
@SuppressWarnings("unchecked")
Map resMap = (Map) o;
String name = (String) resMap.get("name");
Date modified = (Date) resMap.get("lastModified");
String author = (String) resMap.get("author");
Integer version = (Integer) resMap.get("version");
return new PageInfo(name, modified, author, version);
}
static List toPage(Object[] objs){
List result = new ArrayList();
for(Object o : objs){
result.add(toPage(o));
}
return result;
}
static Page toPage(Object o){
@SuppressWarnings("unchecked")
Map resMap = (Map) o;
String id = (String) resMap.get("id");
Integer perms = toPerms(resMap.get("perms"));
Date lastModified = (Date) resMap.get("lastModified");
Integer size = (Integer) resMap.get("size");
return new Page(id, perms, lastModified, size);
}
static List toString(Object[] objs){
List result = new ArrayList();
for(Object o : objs){
result.add((String) o);
}
return result;
}
static List toLinkInfo(Object[] objs){
List result = new ArrayList();
for ( Object o : objs ){
result.add(toLinkInfo(o));
}
return result;
}
static LinkInfo toLinkInfo(Object o){
@SuppressWarnings("unchecked")
Map resMap = (Map) o;
String type = (String) resMap.get("type");
String page = (String) resMap.get("page");
String href = (String) resMap.get("href");
return new LinkInfo(type, page, href);
}
static List toPageChange(Object[] objs){
List result = new ArrayList();
for(Object o : objs){
result.add(toPageChange(o));
}
return result;
}
static PageChange toPageChange(Object o){
@SuppressWarnings("unchecked")
Map resMap = (Map) o;
String id = (String) resMap.get("name");
Integer perms = toPerms(resMap.get("perms"));
Date lastModified = (Date) resMap.get("lastModified");
Integer size = null;
try {
size = (Integer) resMap.get("size");
} catch (ClassCastException e)
{
//Sometimes Dokuwiki fails to get a size (it seems to be the case if the
//corresponding page doesn't exist anymore)
size = null;
}
String author = (String) resMap.get("author");
Integer version = (Integer) resMap.get("version");
return new PageChange(id, perms, lastModified, size, author, version);
}
static List toPageVersion(Object[] objs, String pageId){
List result = new ArrayList();
for ( Object o : objs ){
result.add(toPageVersion(o, pageId));
}
return result;
}
static PageVersion toPageVersion(Object o, String pageId){
@SuppressWarnings("unchecked")
Map resMap = (Map) o;
String author = (String) resMap.get("author");
if ( author == null ){
author = (String) resMap.get("user");
}
String ip = (String) resMap.get("ip");
String type = (String) resMap.get("type");
String summary = (String) resMap.get("sum");
Date modified = (Date) resMap.get("modified");
Integer version = (Integer) resMap.get("version");
return new PageVersion(pageId, author, ip, type, summary, modified, version);
}
static Integer toPerms(Object o){
//Because DW may sometime return a string instead
//(fixed after Adora Belle (2012-10-03))
if (o instanceof Integer){
return (Integer) o;
}
return Integer.valueOf((String) o);
}
}
//! @endcond
© 2015 - 2025 Weber Informatics LLC | Privacy Policy