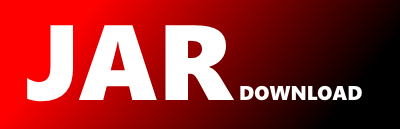
fr.velossity.sample.device.impl.MockDeviceImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iPOJOProducerWB Show documentation
Show all versions of iPOJOProducerWB Show documentation
Sample of producer using iPOJO
package fr.velossity.sample.device.impl;
import java.util.Random;
import fr.velossity.sample.device.Device;
import fr.velossity.sample.device.Measurement;
import fr.velossity.sample.device.eventing.MeasurementHandler;
/**
*
* @author C. Saint-Marcel
*
*/
public class MockDeviceImpl implements Device, Runnable {
private String identifier;
private Thread measureGenerator;
/**
* iPOJO injected field.
*/
private MeasurementHandler myMeasurementHandler;
private Measurement lastMeasurement;
/**
* iPOJO constructor.
*/
public MockDeviceImpl() {
super();
}
/**
* Default constructor.
*/
public MockDeviceImpl(String identifier) {
this();
this.identifier = identifier;
}
public String getIdentifier() {
return identifier;
}
public String getType() {
return "Mock";
}
/**
* Mock device is always functional
*/
public String getState() {
return DEVICE_STATE_FUNCTIONAL;
}
public Measurement getLastMeasure() {
return lastMeasurement;
}
public void activate() {
if(measureGenerator == null) {
measureGenerator = new Thread(this);
measureGenerator.start();
}
}
public void deactivate() {
measureGenerator = null;
}
public void run() {
Thread mg = measureGenerator;
while (measureGenerator == mg) {
// Regenerate last measurement
lastMeasurement = new Measurement(MockDeviceImpl.this, new Random().nextFloat()* 1000, "Watt", System.currentTimeMillis());
// INVOKES the handler with the new measurement
myMeasurementHandler.newMeasure(lastMeasurement);
try {
synchronized (this) {
wait(10000);
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy