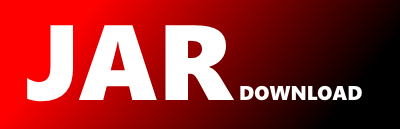
urn.oasis.names.tc.saml.protocol.ObjectFactory Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.4-2
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2014.10.27 at 06:20:33 PM CET
//
package urn.oasis.names.tc.saml.protocol;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the oasis.names.tc.saml._1_0.protocol package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _Query_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:protocol", "Query");
private final static QName _Request_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:protocol", "Request");
private final static QName _Status_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:protocol", "Status");
private final static QName _SubjectQuery_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:protocol", "SubjectQuery");
private final static QName _AttributeQuery_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:protocol", "AttributeQuery");
private final static QName _AuthorizationDecisionQuery_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:protocol", "AuthorizationDecisionQuery");
private final static QName _Response_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:protocol", "Response");
private final static QName _AuthenticationQuery_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:protocol", "AuthenticationQuery");
private final static QName _AssertionArtifact_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:protocol", "AssertionArtifact");
private final static QName _StatusCode_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:protocol", "StatusCode");
private final static QName _RespondWith_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:protocol", "RespondWith");
private final static QName _StatusMessage_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:protocol", "StatusMessage");
private final static QName _StatusDetail_QNAME = new QName("urn:oasis:names:tc:SAML:1.0:protocol", "StatusDetail");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: oasis.names.tc.saml._1_0.protocol
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link StatusCodeType }
*
*/
public StatusCodeType createStatusCodeType() {
return new StatusCodeType();
}
/**
* Create an instance of {@link AuthorizationDecisionQueryType }
*
*/
public AuthorizationDecisionQueryType createAuthorizationDecisionQueryType() {
return new AuthorizationDecisionQueryType();
}
/**
* Create an instance of {@link StatusType }
*
*/
public StatusType createStatusType() {
return new StatusType();
}
/**
* Create an instance of {@link ResponseType }
*
*/
public ResponseType createResponseType() {
return new ResponseType();
}
/**
* Create an instance of {@link RequestType }
*
*/
public RequestType createRequestType() {
return new RequestType();
}
/**
* Create an instance of {@link StatusDetailType }
*
*/
public StatusDetailType createStatusDetailType() {
return new StatusDetailType();
}
/**
* Create an instance of {@link AttributeQueryType }
*
*/
public AttributeQueryType createAttributeQueryType() {
return new AttributeQueryType();
}
/**
* Create an instance of {@link AuthenticationQueryType }
*
*/
public AuthenticationQueryType createAuthenticationQueryType() {
return new AuthenticationQueryType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link QueryAbstractType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:protocol", name = "Query")
public JAXBElement createQuery(QueryAbstractType value) {
return new JAXBElement(_Query_QNAME, QueryAbstractType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link RequestType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:protocol", name = "Request")
public JAXBElement createRequest(RequestType value) {
return new JAXBElement(_Request_QNAME, RequestType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StatusType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:protocol", name = "Status")
public JAXBElement createStatus(StatusType value) {
return new JAXBElement(_Status_QNAME, StatusType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link SubjectQueryAbstractType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:protocol", name = "SubjectQuery")
public JAXBElement createSubjectQuery(SubjectQueryAbstractType value) {
return new JAXBElement(_SubjectQuery_QNAME, SubjectQueryAbstractType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AttributeQueryType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:protocol", name = "AttributeQuery")
public JAXBElement createAttributeQuery(AttributeQueryType value) {
return new JAXBElement(_AttributeQuery_QNAME, AttributeQueryType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AuthorizationDecisionQueryType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:protocol", name = "AuthorizationDecisionQuery")
public JAXBElement createAuthorizationDecisionQuery(AuthorizationDecisionQueryType value) {
return new JAXBElement(_AuthorizationDecisionQuery_QNAME, AuthorizationDecisionQueryType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ResponseType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:protocol", name = "Response")
public JAXBElement createResponse(ResponseType value) {
return new JAXBElement(_Response_QNAME, ResponseType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AuthenticationQueryType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:protocol", name = "AuthenticationQuery")
public JAXBElement createAuthenticationQuery(AuthenticationQueryType value) {
return new JAXBElement(_AuthenticationQuery_QNAME, AuthenticationQueryType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:protocol", name = "AssertionArtifact")
public JAXBElement createAssertionArtifact(String value) {
return new JAXBElement(_AssertionArtifact_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StatusCodeType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:protocol", name = "StatusCode")
public JAXBElement createStatusCode(StatusCodeType value) {
return new JAXBElement(_StatusCode_QNAME, StatusCodeType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link QName }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:protocol", name = "RespondWith")
public JAXBElement createRespondWith(QName value) {
return new JAXBElement(_RespondWith_QNAME, QName.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:protocol", name = "StatusMessage")
public JAXBElement createStatusMessage(String value) {
return new JAXBElement(_StatusMessage_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StatusDetailType }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:oasis:names:tc:SAML:1.0:protocol", name = "StatusDetail")
public JAXBElement createStatusDetail(StatusDetailType value) {
return new JAXBElement(_StatusDetail_QNAME, StatusDetailType.class, null, value);
}
}