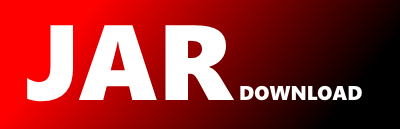
fr.zebasto.spring.identity.contract.service.UserService Maven / Gradle / Ivy
package fr.zebasto.spring.identity.contract.service;
import fr.zebasto.spring.identity.contract.model.Group;
import fr.zebasto.spring.identity.contract.model.Permission;
import fr.zebasto.spring.identity.contract.model.Role;
import fr.zebasto.spring.identity.contract.model.User;
import java.io.Serializable;
import java.util.List;
/**
* Description of the service that manages {@link User}
*
* @param The parametrized type used to define the entity
* @param The parametrized type used to identify an entity {@link Serializable}
* @author Bastien Cecchinato
* @since 1.0.0
*/
public interface UserService, I extends Serializable> extends CrudService {
/**
* Find user by login.
*
* @param login User login
* @return the user or null if more than one user is found
* @since 1.0.0
*/
T findByLogin(String login);
/**
* Update the password for the given user
*
* @param user the user to with the new password
* @return the updated user
* @since 1.0.0
*/
T updatePassword(T user);
/**
* Authenticate the user with Login and password
*
* @param login the login of the user
* @param password the password of the user
* @return the authenticated user or null if no user found with such login
* and password
* @since 1.0.0
*/
T authenticateUser(String login, String password);
/**
* Remove a group from one user's groups
*
* @param userLogin the login of the user to whom to group should be remove
* @param groupName the name of the group to remove from the user's group list
* @since 1.0.0
*/
void removeGroupFromUser(String userLogin, String groupName);
/**
* gets the User's inherited Permissions
*
* @param login the login of the user
* @return permissions of the user. In this implementation inherited
* permissions from group to which the user belong are taken into
* accounts
* @since 1.0.0
*/
List getUserPermissions(String login);
/**
* gets the User's inherited Permissions for a given application
*
* @param login the login of the user
* @param application the application name
* @return permissions of the user. In this implementation inherited
* permissions from group to which the user belong are taken into
* accounts
* @since 1.0.0
*/
List getUserPermissions(String login, String application);
/**
* gets the User's direct Permissions
*
* @param login the login of the user
* @return permissions of the user.
* @since 1.0.0
*/
List getUserDirectPermissions(String login);
/**
* Add a permission to an user
*
* @param userLogin the login of the user
* @param permission the permission to be added
* @since 1.0.0
*/
void addPermissionToUser(String userLogin, Permission permission);
/**
* Remove the permission for the given user
*
* @param userLogin the login of the user
* @param permission the permission to delete
* @since 1.0.0
*/
void removePermissionFromUser(String userLogin, Permission permission);
/**
* Add a group from one user's groups
*
* @param userLogin the login of the user to whom to group should be added
* @param groupName the name of the group to add from the user's group list
* @since 1.0.0
*/
void addGroupToUser(String userLogin, String groupName);
/**
* Gets the user of a group.
*
* @param groupName The name of the group.
* @return A list of users corresponding to the given group.
* @since 1.0.0
*/
List getUsersFromGroup(String groupName);
/**
* Gets all the users that have a role, direct or inherited.
*
* @param roles A list of roles to look for.
* @return A list of users having at least one of the roles defined as
* parameter.
* @since 1.0.0
*/
List findAllUsersWithRoles(List roles);
/**
* Add a role to a user.
*
* @param userLogin User to which the role will be added.
* @param roleName The role that will be added.
* @since 1.0.0
*/
void addRoleToUser(String userLogin, String roleName);
/**
* Remove a role from a user.
*
* @param userLogin User to which the role will be removed.
* @param roleName The role that will be removed.
* @since 1.0.0
*/
void removeRoleFromUser(String userLogin, String roleName);
/**
* Get all the groups a user owns.
*
* @param userLogin User to gather groups from.
* @return A list of groups that the given user has.
* @since 1.0.0
*/
List getAllUserGroups(String userLogin);
/**
* Get all the roles a user owns.
*
* @param userLogin User to gather roles from.
* @return A list of roles that the given user has.
* @since 1.0.0
*/
List getAllUserRoles(String userLogin);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy