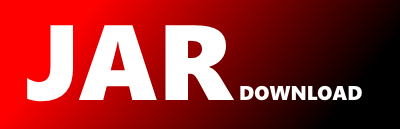
fun.bigtable.kraken.exception.BusinessAssert Maven / Gradle / Ivy
package fun.bigtable.kraken.exception;
import fun.bigtable.kraken.authority.IUserBelongCheck;
import org.apache.commons.collections4.CollectionUtils;
import org.springframework.util.ObjectUtils;
import java.util.Arrays;
import java.util.Collection;
import java.util.Objects;
/**
* 断言工具类
*/
public class BusinessAssert {
/**
* 表达式为真则抛出异常
*/
public static void ifTrue(boolean expression, String errorMsg) throws BusinessException {
if (expression) {
throw BusinessException.error(errorMsg);
}
}
public static void ifFalse(boolean expression, String errorMsg) throws BusinessException {
if (!expression) {
throw BusinessException.error( errorMsg);
}
}
/**
* 集合为空则抛出异常
*/
public static void ifCollectionEmpty(Collection> collection, String errorMsg) throws BusinessException {
ifTrue(CollectionUtils.isEmpty(collection), errorMsg);
}
/**
* 对象为空则抛出异常
*/
public static void ifNull(Object object, String errorMsg) throws BusinessException {
ifTrue(Objects.isNull(object), errorMsg);
}
/**
* 为空抛出异常
*
* @param object 对象
* @param errorMsg 错位描述
* @throws BusinessException 业务异常
*/
public static void ifEmpty(Object object, String errorMsg) throws BusinessException {
ifTrue(ObjectUtils.isEmpty(object), errorMsg);
}
public static void collectionSizeGtOne(Collection> collection, String errorMsg) throws BusinessException {
ifTrue(collection.size() > 1, errorMsg);
}
/**
* 所有参数不能为null
* 错误信息在前
*/
public static void allNonNull(String errMsg, Object... check) throws BusinessException {
ifTrue(Objects.isNull(check) || Arrays.stream(check).anyMatch(Objects::isNull), errMsg);
}
/**
* 参数不为空
*/
public static void paramNotNull(Object param) throws BusinessException {
if(Objects.isNull(param)){
throw BusinessException.error("参数为空");
}
}
public static void ifTrue(boolean expression, String errorMsg, Type errorType) throws BusinessException {
if (expression) {
throw BusinessException.error(errorMsg);
}
}
/**
* 校验必传参数
*/
public static void checkMustParam(Object... object) throws BusinessException {
allNonNull("必要参数未传",object);
}
/**
* 数据越权检查
*
* @param belongId 归属用户id
* @param belongCheck 需要判断的数据,需要实现指定接口
*/
public static void userDataCheck(Long belongId, IUserBelongCheck belongCheck) {
ifFalse(Objects.equals(belongCheck.getBelonging(), belongId),"数据越权");
}
/**
* 检查数据是否存在并返回
*/
public static T linkDataOpt(T data){
ifNull(data, "无关联数据");
return data;
}
private BusinessAssert() {
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy