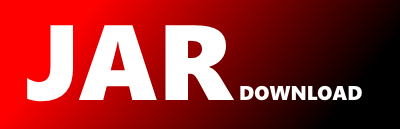
fun.bigtable.kraken.util.JsonUtils Maven / Gradle / Ivy
package fun.bigtable.kraken.util;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonParseException;
import com.google.gson.JsonPrimitive;
import com.google.gson.JsonSerializationContext;
import com.google.gson.JsonSerializer;
import com.google.gson.reflect.TypeToken;
import java.lang.reflect.Type;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import java.util.Objects;
/**
* 基于Gson的json工具类
*/
public class JsonUtils {
private static Gson gson;
public static Gson getInstance() {
if (Objects.isNull(gson)) {
synchronized (JsonUtils.class) {
if (Objects.isNull(gson)) {
GsonBuilder gsonBuilder = new GsonBuilder();
gsonBuilder.serializeNulls();
gsonBuilder.registerTypeAdapter(LocalDateTime.class, new LocalDateAdapter());
gson = gsonBuilder.create();
}
}
}
return gson;
}
/**
* 转 json 字符串
*/
public static String toJson(Object o) {
return getInstance().toJson(o);
}
/**
* 转对象
*/
public static T parserObject(String json, Class classOfT) {
return getInstance().fromJson(json, classOfT);
}
/**
* 自定义
*/
public static T parserCustom(String json, TypeToken typeToken) {
return getInstance().fromJson(json, typeToken.getType());
}
public static class LocalDateAdapter implements JsonSerializer, JsonDeserializer {
@Override
public JsonElement serialize(LocalDateTime localDateTime, Type type, JsonSerializationContext jsonSerializationContext) {
return new JsonPrimitive(localDateTime.format(DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss")));
}
@Override
public LocalDateTime deserialize(JsonElement json, Type typeOfT, JsonDeserializationContext context) throws JsonParseException {
if (!json.isJsonNull()) {
String asString = json.getAsString();
return DateTimeUtils.parserDateTime(asString);
}
return null;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy