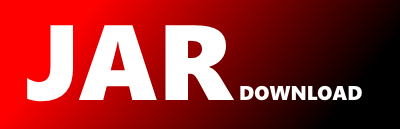
fun.freechat.client.model.AgentDetailsDTO Maven / Gradle / Ivy
/*
* FreeChat OpenAPI Definition
* # FreeChat: Create Friends for Yourself with AI English | [中文版](https://github.com/freechat-fun/freechat/blob/main/README.zh-CN.md) ## Introduction Welcome! FreeChat aims to build a cloud-native, robust, and quickly commercializable enterprise-level AI virtual character platform. It also serves as a prompt engineering platform. ## Features - Primarily uses Java and emphasizes **security, robustness, scalability, traceability, and maintainability**. - Boasts **account systems and permission management**, supporting OAuth2 authentication. Introduces the \"organization\" concept and related permission constraint functions. - Extensively employs distributed technologies and caching to support **high concurrency** access. - Provides flexible character customization options, supports direct intervention in prompts, and supports **configuring multiple backends for each character**. - **Offers a comprehensive range of Open APIs**, with more than 180 interfaces and provides java/python/typescript SDKs. These interfaces enable easy construction of systems for end-users. - Supports setting **RAG** (Retrieval Augmented Generation) for characters. - Supports **long-term memory, preset memory** for characters. - Supports characters evoking **proactive chat**. - Supports setting **quota limits** for characters. - Supports characters **importing and exporting**. - Supports individual **debugging and sharing prompts**. ## Snapshots ### On PC #### Home Page  #### Development View  #### Chat View  ### On Mobile  
  ## Character Design ```mermaid flowchart TD A(Character) --> B(Profile) A --> C(Knowledge/RAG) A --> D(Album) A --> E(Backend-1) A --> F(Backend-n...) E --> G(Message Window) E --> H(Long Term Memory Settings) E --> I(Quota Limit) E --> J(Chat Prompt Task) E --> K(Greeting Prompt Task) E --> L(Moderation Settings) J --> M(Model & Parameters) J --> N(API Keys) J --> O(Prompt Refence) J --> P(Tool Specifications) O --> Q(Template) O --> R(Variables) O --> S(Version) O --> T(...) style K stroke-dasharray: 5, 5 style L stroke-dasharray: 5, 5 style P stroke-dasharray: 5, 5 ``` After setting up an unified persona and knowledge for a character, different backends can be configured. For example, different model may be adopted for different users based on cost considerations. ## How to Play ### Online Website You can visit [freechat.fun](https://freechat.fun) to experience FreeChat. Share your designed AI character! ### Running in a Kubernetes Cluster FreeChat is dedicated to the principles of cloud-native design. If you have a Kubernetes cluster, you can deploy FreeChat to your environment by following these steps: 1. Put the Kubernetes configuration file in the `configs/helm/` directory, named `kube-private.conf`. 2. Place the Helm configuration file in the same directory, named `values-private.yaml`. Make sure to reference the default `values.yaml` and customize the variables as needed. 3. Switch to the `scripts/` directory. 4. If needed, run `install-in.sh` to deploy `ingress-nginx` on the Kubernetes cluster. 5. If needed, run `install-cm.sh` to deploy `cert-manager` on the Kubernetes cluster, which automatically issues certificates for domains specified in `ingress.hosts`. 6. Run `install-pvc.sh` to install PersistentVolumeClaim resources. > By default, FreeChat operates files by accessing the \"local file system.\" You may want to use high-availability distributed storage in the cloud. As a cloud-native-designed system, we recommend interfacing through Kubernetes CSI to avoid individually adapting storage products for each cloud platform. Most cloud service providers offer cloud storage drivers for Kubernetes, with a series of predefined StorageClass resources. Please choose the appropriate configuration according to your actual needs and set it in Helm's `global.storageClass` option. > > *In the future, FreeChat may be refactored to use MinIO's APIs directly, as it is now installed in the Kubernetes cluster as a dependency (serving Milvus).* 7. Run `install.sh` script to install FreeChat and its dependencies. 8. FreeChat aims to provide Open API services. If you like the interactive experience of [freechat.fun](https://freechat.fun), run `install-web.sh` to deploy the front-end application. 9. Run `restart.sh` to restart the service. 10. If you modified any Helm configuration files, use `upgrade.sh` to update the corresponding Kubernetes resources. 11. To remove specific resources, run the `uninstall*.sh` script corresponding to the resource you want to uninstall. As a cloud-native application, the services FreeChat relies on are obtained and deployed to your cluster through the helm repository. If you prefer cloud services with SLA (Service Level Agreement) guarantees, simply make the relevant settings in `configs/helm/values-private.yaml`: ```yaml mysql: deployment: enabled: false url: auth: rootPassword: username: password: redis: deployment: enabled: false url: auth: password: milvus: deployment: enabled: false url: milvus: auth: token: ``` With this, FreeChat will not automatically install these services, but rather use the configuration information to connect directly. If your Kubernetes cluster does not have a standalone monitoring system, you can enable the following switch. This will install Prometheus and Grafana services in the same namespace, dedicated to monitoring the status of the services under the FreeChat application: ```yaml prometheus: deployment: enabled: true grafana: deployment: enabled: true ``` ### Running Locally You can also run FreeChat locally. Currently supported on MacOS and Linux (although only tested on MacOS). You need to install the Docker toolset and have a network that can access [Docker Hub](https://hub.docker.com/). Once ready, enter the `scripts/` directory and run `local-run.sh`, which will download and run the necessary docker containers. After a successful startup, you can access `http://localhost` via a browser to see the locally running freechat.fun. The built-in administrator username and password are \"admin:freechat\". Use `local-run.sh --help` to view the supported options of the script. Good luck! ### Running in an IDE To run FreeChat in an IDE, you need to start all dependent services first but do not need to run the container for the FreeChat application itself. You can execute the `scripts/local-deps.sh` script to start services like `MySQL`, `Redis`, `Milvus`, etc., locally. Once done, open and debug `freechat-start/src/main/java/fun/freechat/Application.java`。Make sure you have set the following startup VM options: ```shell -Dspring.config.location=classpath:/application.yml,classpath:/application-local.yml \\ -DAPP_HOME=local-data/freechat \\ -Dspring.profiles.active=local ``` ### Use SDK #### Java - **Dependency** ```xml fun.freechat freechat-sdk ${freechat-sdk.version} ``` - **Example** ```java import fun.freechat.client.ApiClient; import fun.freechat.client.ApiException; import fun.freechat.client.Configuration; import fun.freechat.client.api.AccountApi; import fun.freechat.client.auth.ApiKeyAuth; import fun.freechat.client.model.UserDetailsDTO; public class AccountClientExample { public static void main(String[] args) { ApiClient defaultClient = Configuration.getDefaultApiClient(); defaultClient.setBasePath(\"https://freechat.fun\"); // Configure HTTP bearer authorization: bearerAuth HttpBearerAuth bearerAuth = (HttpBearerAuth) defaultClient.getAuthentication(\"bearerAuth\"); bearerAuth.setBearerToken(\"FREECHAT_TOKEN\"); AccountApi apiInstance = new AccountApi(defaultClient); try { UserDetailsDTO result = apiInstance.getUserDetails(); System.out.println(result); } catch (ApiException e) { e.printStackTrace(); } } } ``` #### Python - **Installation** ```shell pip install freechat-sdk ``` - **Example** ```python import freechat_sdk from freechat_sdk.rest import ApiException from pprint import pprint # Defining the host is optional and defaults to https://freechat.fun # See configuration.py for a list of all supported configuration parameters. configuration = freechat_sdk.Configuration( host = \"https://freechat.fun\" ) # Configure Bearer authorization: bearerAuth configuration = freechat_sdk.Configuration( access_token = os.environ[\"FREECHAT_TOKEN\"] ) # Enter a context with an instance of the API client with freechat_sdk.ApiClient(configuration) as api_client: # Create an instance of the API class api_instance = freechat_sdk.AccountApi(api_client) try: details = api_instance.get_user_details() pprint(details) except ApiException as e: print(\"Exception when calling AccountClient->get_user_details: %s\\n\" % e) ``` #### TypeScript - **Installation** ```shell npm install freechat-sdk --save ``` - **Example** Refer to [FreeChatApiContext.tsx](https://github.com/freechat-fun/freechat/blob/main/freechat-web/src/contexts/FreeChatApiProvider.tsx) ## System Dependencies | | Projects | ---- | ---- | Application Framework | [Spring Boot](https://spring.io/projects/spring-boot/) | LLM Framework | [LangChain4j](https://docs.langchain4j.dev/) | Model Providers | [OpenAI](https://platform.openai.com/), [Azure OpenAI](https://oai.azure.com/), [DashScope(Alibaba)](https://dashscope.aliyun.com/) | Database Systems | [MySQL](https://www.mysql.com/), [Redis](https://redis.io/), [Milvus](https://milvus.io/) | Monitoring & Alerting | [Kube State Metrics](https://kubernetes.io/docs/concepts/cluster-administration/kube-state-metrics/), [Prometheus](https://prometheus.io/), [Promtail](https://grafana.com/docs/loki/latest/send-data/promtail/), [Loki](https://grafana.com/oss/loki/), [Grafana](https://grafana.com/) | OpenAPI Tools | [Springdoc-openapi](https://springdoc.org/), [OpenAPI Generator](https://github.com/OpenAPITools/openapi-generator), [OpenAPI Explorer](https://github.com/Authress-Engineering/openapi-explorer) ## Collaboration ### Application Integration The FreeChat system is entirely oriented towards Open APIs. The site [freechat.fun](https://freechat.fun) is developed using its TypeScript SDK and hardly depends on private interfaces. You can use these online interfaces to develop your own applications or sites, making them fit your preferences. Currently, FreeChat is completely free with no paid plans (after all, users use their own API Key to call LLM services). ### Model Integration FreeChat aims to explore AI virtual character technology with anthropomorphic characteristics. So far, it supports model services from OpenAI GPT and Alibaba Qwen series models. However, we are more interested in supporting models that are under research and can endow AI with more personality traits. If you are researching this area and hope FreeChat supports your model, please contact us. We look forward to AI technology helping people create their own \"soul mates\" in the future.
*
* The version of the OpenAPI document: 2.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package fun.freechat.client.model;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import fun.freechat.client.model.AiModelInfoDTO;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import fun.freechat.client.JSON;
/**
* Agent detailed content
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.9.0")
public class AgentDetailsDTO {
public static final String SERIALIZED_NAME_REQUEST_ID = "requestId";
@SerializedName(SERIALIZED_NAME_REQUEST_ID)
private String requestId;
public static final String SERIALIZED_NAME_AGENT_ID = "agentId";
@SerializedName(SERIALIZED_NAME_AGENT_ID)
private Long agentId;
public static final String SERIALIZED_NAME_AGENT_UID = "agentUid";
@SerializedName(SERIALIZED_NAME_AGENT_UID)
private String agentUid;
public static final String SERIALIZED_NAME_GMT_CREATE = "gmtCreate";
@SerializedName(SERIALIZED_NAME_GMT_CREATE)
private OffsetDateTime gmtCreate;
public static final String SERIALIZED_NAME_GMT_MODIFIED = "gmtModified";
@SerializedName(SERIALIZED_NAME_GMT_MODIFIED)
private OffsetDateTime gmtModified;
public static final String SERIALIZED_NAME_PARENT_UID = "parentUid";
@SerializedName(SERIALIZED_NAME_PARENT_UID)
private String parentUid;
public static final String SERIALIZED_NAME_VISIBILITY = "visibility";
@SerializedName(SERIALIZED_NAME_VISIBILITY)
private String visibility;
public static final String SERIALIZED_NAME_FORMAT = "format";
@SerializedName(SERIALIZED_NAME_FORMAT)
private String format;
public static final String SERIALIZED_NAME_VERSION = "version";
@SerializedName(SERIALIZED_NAME_VERSION)
private Integer version;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_USERNAME = "username";
@SerializedName(SERIALIZED_NAME_USERNAME)
private String username;
public static final String SERIALIZED_NAME_TAGS = "tags";
@SerializedName(SERIALIZED_NAME_TAGS)
private List tags = new ArrayList<>();
public static final String SERIALIZED_NAME_AI_MODELS = "aiModels";
@SerializedName(SERIALIZED_NAME_AI_MODELS)
private List aiModels = new ArrayList<>();
public static final String SERIALIZED_NAME_CONFIG = "config";
@SerializedName(SERIALIZED_NAME_CONFIG)
private String config;
public static final String SERIALIZED_NAME_EXAMPLE = "example";
@SerializedName(SERIALIZED_NAME_EXAMPLE)
private String example;
public static final String SERIALIZED_NAME_PARAMETERS = "parameters";
@SerializedName(SERIALIZED_NAME_PARAMETERS)
private String parameters;
public static final String SERIALIZED_NAME_EXT = "ext";
@SerializedName(SERIALIZED_NAME_EXT)
private String ext;
public static final String SERIALIZED_NAME_DRAFT = "draft";
@SerializedName(SERIALIZED_NAME_DRAFT)
private String draft;
public AgentDetailsDTO() {
}
public AgentDetailsDTO requestId(String requestId) {
this.requestId = requestId;
return this;
}
/**
* Request identifier
* @return requestId
*/
@javax.annotation.Nullable
public String getRequestId() {
return requestId;
}
public void setRequestId(String requestId) {
this.requestId = requestId;
}
public AgentDetailsDTO agentId(Long agentId) {
this.agentId = agentId;
return this;
}
/**
* Agent identifier, will change after publish
* @return agentId
*/
@javax.annotation.Nullable
public Long getAgentId() {
return agentId;
}
public void setAgentId(Long agentId) {
this.agentId = agentId;
}
public AgentDetailsDTO agentUid(String agentUid) {
this.agentUid = agentUid;
return this;
}
/**
* Agent immutable identifier
* @return agentUid
*/
@javax.annotation.Nullable
public String getAgentUid() {
return agentUid;
}
public void setAgentUid(String agentUid) {
this.agentUid = agentUid;
}
public AgentDetailsDTO gmtCreate(OffsetDateTime gmtCreate) {
this.gmtCreate = gmtCreate;
return this;
}
/**
* Creation time
* @return gmtCreate
*/
@javax.annotation.Nullable
public OffsetDateTime getGmtCreate() {
return gmtCreate;
}
public void setGmtCreate(OffsetDateTime gmtCreate) {
this.gmtCreate = gmtCreate;
}
public AgentDetailsDTO gmtModified(OffsetDateTime gmtModified) {
this.gmtModified = gmtModified;
return this;
}
/**
* Modification time
* @return gmtModified
*/
@javax.annotation.Nullable
public OffsetDateTime getGmtModified() {
return gmtModified;
}
public void setGmtModified(OffsetDateTime gmtModified) {
this.gmtModified = gmtModified;
}
public AgentDetailsDTO parentUid(String parentUid) {
this.parentUid = parentUid;
return this;
}
/**
* Referenced agent
* @return parentUid
*/
@javax.annotation.Nullable
public String getParentUid() {
return parentUid;
}
public void setParentUid(String parentUid) {
this.parentUid = parentUid;
}
public AgentDetailsDTO visibility(String visibility) {
this.visibility = visibility;
return this;
}
/**
* Visibility: private, public, public_org, hidden
* @return visibility
*/
@javax.annotation.Nullable
public String getVisibility() {
return visibility;
}
public void setVisibility(String visibility) {
this.visibility = visibility;
}
public AgentDetailsDTO format(String format) {
this.format = format;
return this;
}
/**
* Agent format, currently supported: langflow
* @return format
*/
@javax.annotation.Nullable
public String getFormat() {
return format;
}
public void setFormat(String format) {
this.format = format;
}
public AgentDetailsDTO version(Integer version) {
this.version = version;
return this;
}
/**
* Version
* @return version
*/
@javax.annotation.Nullable
public Integer getVersion() {
return version;
}
public void setVersion(Integer version) {
this.version = version;
}
public AgentDetailsDTO name(String name) {
this.name = name;
return this;
}
/**
* Agent name
* @return name
*/
@javax.annotation.Nullable
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public AgentDetailsDTO description(String description) {
this.description = description;
return this;
}
/**
* Agent description
* @return description
*/
@javax.annotation.Nullable
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public AgentDetailsDTO username(String username) {
this.username = username;
return this;
}
/**
* Agent owner
* @return username
*/
@javax.annotation.Nullable
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public AgentDetailsDTO tags(List tags) {
this.tags = tags;
return this;
}
public AgentDetailsDTO addTagsItem(String tagsItem) {
if (this.tags == null) {
this.tags = new ArrayList<>();
}
this.tags.add(tagsItem);
return this;
}
/**
* Tag set
* @return tags
*/
@javax.annotation.Nullable
public List getTags() {
return tags;
}
public void setTags(List tags) {
this.tags = tags;
}
public AgentDetailsDTO aiModels(List aiModels) {
this.aiModels = aiModels;
return this;
}
public AgentDetailsDTO addAiModelsItem(AiModelInfoDTO aiModelsItem) {
if (this.aiModels == null) {
this.aiModels = new ArrayList<>();
}
this.aiModels.add(aiModelsItem);
return this;
}
/**
* Supported model set
* @return aiModels
*/
@javax.annotation.Nullable
public List getAiModels() {
return aiModels;
}
public void setAiModels(List aiModels) {
this.aiModels = aiModels;
}
public AgentDetailsDTO config(String config) {
this.config = config;
return this;
}
/**
* Agent configuration
* @return config
*/
@javax.annotation.Nullable
public String getConfig() {
return config;
}
public void setConfig(String config) {
this.config = config;
}
public AgentDetailsDTO example(String example) {
this.example = example;
return this;
}
/**
* Agent example
* @return example
*/
@javax.annotation.Nullable
public String getExample() {
return example;
}
public void setExample(String example) {
this.example = example;
}
public AgentDetailsDTO parameters(String parameters) {
this.parameters = parameters;
return this;
}
/**
* Agent parameters, JSON format
* @return parameters
*/
@javax.annotation.Nullable
public String getParameters() {
return parameters;
}
public void setParameters(String parameters) {
this.parameters = parameters;
}
public AgentDetailsDTO ext(String ext) {
this.ext = ext;
return this;
}
/**
* Additional information, JSON format
* @return ext
*/
@javax.annotation.Nullable
public String getExt() {
return ext;
}
public void setExt(String ext) {
this.ext = ext;
}
public AgentDetailsDTO draft(String draft) {
this.draft = draft;
return this;
}
/**
* Draft content
* @return draft
*/
@javax.annotation.Nullable
public String getDraft() {
return draft;
}
public void setDraft(String draft) {
this.draft = draft;
}
/**
* A container for additional, undeclared properties.
* This is a holder for any undeclared properties as specified with
* the 'additionalProperties' keyword in the OAS document.
*/
private Map additionalProperties;
/**
* Set the additional (undeclared) property with the specified name and value.
* If the property does not already exist, create it otherwise replace it.
*
* @param key name of the property
* @param value value of the property
* @return the AgentDetailsDTO instance itself
*/
public AgentDetailsDTO putAdditionalProperty(String key, Object value) {
if (this.additionalProperties == null) {
this.additionalProperties = new HashMap();
}
this.additionalProperties.put(key, value);
return this;
}
/**
* Return the additional (undeclared) property.
*
* @return a map of objects
*/
public Map getAdditionalProperties() {
return additionalProperties;
}
/**
* Return the additional (undeclared) property with the specified name.
*
* @param key name of the property
* @return an object
*/
public Object getAdditionalProperty(String key) {
if (this.additionalProperties == null) {
return null;
}
return this.additionalProperties.get(key);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AgentDetailsDTO agentDetailsDTO = (AgentDetailsDTO) o;
return Objects.equals(this.requestId, agentDetailsDTO.requestId) &&
Objects.equals(this.agentId, agentDetailsDTO.agentId) &&
Objects.equals(this.agentUid, agentDetailsDTO.agentUid) &&
Objects.equals(this.gmtCreate, agentDetailsDTO.gmtCreate) &&
Objects.equals(this.gmtModified, agentDetailsDTO.gmtModified) &&
Objects.equals(this.parentUid, agentDetailsDTO.parentUid) &&
Objects.equals(this.visibility, agentDetailsDTO.visibility) &&
Objects.equals(this.format, agentDetailsDTO.format) &&
Objects.equals(this.version, agentDetailsDTO.version) &&
Objects.equals(this.name, agentDetailsDTO.name) &&
Objects.equals(this.description, agentDetailsDTO.description) &&
Objects.equals(this.username, agentDetailsDTO.username) &&
Objects.equals(this.tags, agentDetailsDTO.tags) &&
Objects.equals(this.aiModels, agentDetailsDTO.aiModels) &&
Objects.equals(this.config, agentDetailsDTO.config) &&
Objects.equals(this.example, agentDetailsDTO.example) &&
Objects.equals(this.parameters, agentDetailsDTO.parameters) &&
Objects.equals(this.ext, agentDetailsDTO.ext) &&
Objects.equals(this.draft, agentDetailsDTO.draft)&&
Objects.equals(this.additionalProperties, agentDetailsDTO.additionalProperties);
}
@Override
public int hashCode() {
return Objects.hash(requestId, agentId, agentUid, gmtCreate, gmtModified, parentUid, visibility, format, version, name, description, username, tags, aiModels, config, example, parameters, ext, draft, additionalProperties);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AgentDetailsDTO {\n");
sb.append(" requestId: ").append(toIndentedString(requestId)).append("\n");
sb.append(" agentId: ").append(toIndentedString(agentId)).append("\n");
sb.append(" agentUid: ").append(toIndentedString(agentUid)).append("\n");
sb.append(" gmtCreate: ").append(toIndentedString(gmtCreate)).append("\n");
sb.append(" gmtModified: ").append(toIndentedString(gmtModified)).append("\n");
sb.append(" parentUid: ").append(toIndentedString(parentUid)).append("\n");
sb.append(" visibility: ").append(toIndentedString(visibility)).append("\n");
sb.append(" format: ").append(toIndentedString(format)).append("\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" username: ").append(toIndentedString(username)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" aiModels: ").append(toIndentedString(aiModels)).append("\n");
sb.append(" config: ").append(toIndentedString(config)).append("\n");
sb.append(" example: ").append(toIndentedString(example)).append("\n");
sb.append(" parameters: ").append(toIndentedString(parameters)).append("\n");
sb.append(" ext: ").append(toIndentedString(ext)).append("\n");
sb.append(" draft: ").append(toIndentedString(draft)).append("\n");
sb.append(" additionalProperties: ").append(toIndentedString(additionalProperties)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
public static HashSet openapiFields;
public static HashSet openapiRequiredFields;
static {
// a set of all properties/fields (JSON key names)
openapiFields = new HashSet();
openapiFields.add("requestId");
openapiFields.add("agentId");
openapiFields.add("agentUid");
openapiFields.add("gmtCreate");
openapiFields.add("gmtModified");
openapiFields.add("parentUid");
openapiFields.add("visibility");
openapiFields.add("format");
openapiFields.add("version");
openapiFields.add("name");
openapiFields.add("description");
openapiFields.add("username");
openapiFields.add("tags");
openapiFields.add("aiModels");
openapiFields.add("config");
openapiFields.add("example");
openapiFields.add("parameters");
openapiFields.add("ext");
openapiFields.add("draft");
// a set of required properties/fields (JSON key names)
openapiRequiredFields = new HashSet();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to AgentDetailsDTO
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
if (jsonElement == null) {
if (!AgentDetailsDTO.openapiRequiredFields.isEmpty()) { // has required fields but JSON element is null
throw new IllegalArgumentException(String.format("The required field(s) %s in AgentDetailsDTO is not found in the empty JSON string", AgentDetailsDTO.openapiRequiredFields.toString()));
}
}
JsonObject jsonObj = jsonElement.getAsJsonObject();
if ((jsonObj.get("requestId") != null && !jsonObj.get("requestId").isJsonNull()) && !jsonObj.get("requestId").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `requestId` to be a primitive type in the JSON string but got `%s`", jsonObj.get("requestId").toString()));
}
if ((jsonObj.get("agentUid") != null && !jsonObj.get("agentUid").isJsonNull()) && !jsonObj.get("agentUid").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `agentUid` to be a primitive type in the JSON string but got `%s`", jsonObj.get("agentUid").toString()));
}
if ((jsonObj.get("parentUid") != null && !jsonObj.get("parentUid").isJsonNull()) && !jsonObj.get("parentUid").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `parentUid` to be a primitive type in the JSON string but got `%s`", jsonObj.get("parentUid").toString()));
}
if ((jsonObj.get("visibility") != null && !jsonObj.get("visibility").isJsonNull()) && !jsonObj.get("visibility").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `visibility` to be a primitive type in the JSON string but got `%s`", jsonObj.get("visibility").toString()));
}
if ((jsonObj.get("format") != null && !jsonObj.get("format").isJsonNull()) && !jsonObj.get("format").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `format` to be a primitive type in the JSON string but got `%s`", jsonObj.get("format").toString()));
}
if ((jsonObj.get("name") != null && !jsonObj.get("name").isJsonNull()) && !jsonObj.get("name").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `name` to be a primitive type in the JSON string but got `%s`", jsonObj.get("name").toString()));
}
if ((jsonObj.get("description") != null && !jsonObj.get("description").isJsonNull()) && !jsonObj.get("description").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `description` to be a primitive type in the JSON string but got `%s`", jsonObj.get("description").toString()));
}
if ((jsonObj.get("username") != null && !jsonObj.get("username").isJsonNull()) && !jsonObj.get("username").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `username` to be a primitive type in the JSON string but got `%s`", jsonObj.get("username").toString()));
}
// ensure the optional json data is an array if present
if (jsonObj.get("tags") != null && !jsonObj.get("tags").isJsonNull() && !jsonObj.get("tags").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `tags` to be an array in the JSON string but got `%s`", jsonObj.get("tags").toString()));
}
if (jsonObj.get("aiModels") != null && !jsonObj.get("aiModels").isJsonNull()) {
JsonArray jsonArrayaiModels = jsonObj.getAsJsonArray("aiModels");
if (jsonArrayaiModels != null) {
// ensure the json data is an array
if (!jsonObj.get("aiModels").isJsonArray()) {
throw new IllegalArgumentException(String.format("Expected the field `aiModels` to be an array in the JSON string but got `%s`", jsonObj.get("aiModels").toString()));
}
// validate the optional field `aiModels` (array)
for (int i = 0; i < jsonArrayaiModels.size(); i++) {
AiModelInfoDTO.validateJsonElement(jsonArrayaiModels.get(i));
};
}
}
if ((jsonObj.get("config") != null && !jsonObj.get("config").isJsonNull()) && !jsonObj.get("config").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `config` to be a primitive type in the JSON string but got `%s`", jsonObj.get("config").toString()));
}
if ((jsonObj.get("example") != null && !jsonObj.get("example").isJsonNull()) && !jsonObj.get("example").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `example` to be a primitive type in the JSON string but got `%s`", jsonObj.get("example").toString()));
}
if ((jsonObj.get("parameters") != null && !jsonObj.get("parameters").isJsonNull()) && !jsonObj.get("parameters").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `parameters` to be a primitive type in the JSON string but got `%s`", jsonObj.get("parameters").toString()));
}
if ((jsonObj.get("ext") != null && !jsonObj.get("ext").isJsonNull()) && !jsonObj.get("ext").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `ext` to be a primitive type in the JSON string but got `%s`", jsonObj.get("ext").toString()));
}
if ((jsonObj.get("draft") != null && !jsonObj.get("draft").isJsonNull()) && !jsonObj.get("draft").isJsonPrimitive()) {
throw new IllegalArgumentException(String.format("Expected the field `draft` to be a primitive type in the JSON string but got `%s`", jsonObj.get("draft").toString()));
}
}
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!AgentDetailsDTO.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'AgentDetailsDTO' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter thisAdapter
= gson.getDelegateAdapter(this, TypeToken.get(AgentDetailsDTO.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, AgentDetailsDTO value) throws IOException {
JsonObject obj = thisAdapter.toJsonTree(value).getAsJsonObject();
obj.remove("additionalProperties");
// serialize additional properties
if (value.getAdditionalProperties() != null) {
for (Map.Entry entry : value.getAdditionalProperties().entrySet()) {
if (entry.getValue() instanceof String)
obj.addProperty(entry.getKey(), (String) entry.getValue());
else if (entry.getValue() instanceof Number)
obj.addProperty(entry.getKey(), (Number) entry.getValue());
else if (entry.getValue() instanceof Boolean)
obj.addProperty(entry.getKey(), (Boolean) entry.getValue());
else if (entry.getValue() instanceof Character)
obj.addProperty(entry.getKey(), (Character) entry.getValue());
else {
JsonElement jsonElement = gson.toJsonTree(entry.getValue());
if (jsonElement.isJsonArray()) {
obj.add(entry.getKey(), jsonElement.getAsJsonArray());
} else {
obj.add(entry.getKey(), jsonElement.getAsJsonObject());
}
}
}
}
elementAdapter.write(out, obj);
}
@Override
public AgentDetailsDTO read(JsonReader in) throws IOException {
JsonElement jsonElement = elementAdapter.read(in);
validateJsonElement(jsonElement);
JsonObject jsonObj = jsonElement.getAsJsonObject();
// store additional fields in the deserialized instance
AgentDetailsDTO instance = thisAdapter.fromJsonTree(jsonObj);
for (Map.Entry entry : jsonObj.entrySet()) {
if (!openapiFields.contains(entry.getKey())) {
if (entry.getValue().isJsonPrimitive()) { // primitive type
if (entry.getValue().getAsJsonPrimitive().isString())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsString());
else if (entry.getValue().getAsJsonPrimitive().isNumber())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsNumber());
else if (entry.getValue().getAsJsonPrimitive().isBoolean())
instance.putAdditionalProperty(entry.getKey(), entry.getValue().getAsBoolean());
else
throw new IllegalArgumentException(String.format("The field `%s` has unknown primitive type. Value: %s", entry.getKey(), entry.getValue().toString()));
} else if (entry.getValue().isJsonArray()) {
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), List.class));
} else { // JSON object
instance.putAdditionalProperty(entry.getKey(), gson.fromJson(entry.getValue(), HashMap.class));
}
}
}
return instance;
}
}.nullSafe();
}
}
/**
* Create an instance of AgentDetailsDTO given an JSON string
*
* @param jsonString JSON string
* @return An instance of AgentDetailsDTO
* @throws IOException if the JSON string is invalid with respect to AgentDetailsDTO
*/
public static AgentDetailsDTO fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, AgentDetailsDTO.class);
}
/**
* Convert an instance of AgentDetailsDTO to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy