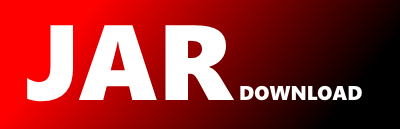
fun.langel.cql.rv.Rows Maven / Gradle / Ivy
The newest version!
package fun.langel.cql.rv;
import fun.langel.cql.exception.MappingException;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
/**
* @author [email protected](GuHan)
* @since 2021/11/4 11:31 上午
**/
public class Rows implements ReturnValue> {
private final List rows = new LinkedList<>();
private long size;
public static final Rows EMPTY = new Rows();
public Rows() {
this(null);
}
public Rows(final List rows) {
if (rows == null || rows.isEmpty()) {
return;
}
this.rows.addAll(rows);
}
public void add(final Row row) {
this.rows.add(row);
}
public void setSize(final long size) {
this.size = size;
}
public long size() {
if (this.size != 0) {
return this.size;
}
if (this.rows == null || this.rows.isEmpty()) {
return 0;
}
return this.rows.size();
}
@Override
public List getValue() {
return this.rows;
}
@Override
public Object mapTo(Class> klass) throws MappingException {
if (this.rows == null) {
return null;
}
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy