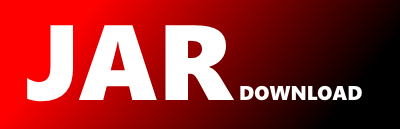
fun.mike.crypto.pgp.alpha.KeyRingFinder Maven / Gradle / Ivy
package fun.mike.crypto.pgp.alpha;
import java.util.LinkedList;
import java.util.List;
import java.util.Optional;
import org.bouncycastle.openpgp.PGPException;
import org.bouncycastle.openpgp.PGPPublicKeyRing;
import org.bouncycastle.openpgp.PGPPublicKeyRingCollection;
import org.bouncycastle.openpgp.PGPSecretKeyRing;
import org.bouncycastle.openpgp.PGPSecretKeyRingCollection;
public class KeyRingFinder {
public static Optional findPublicKeyRing(PGPPublicKeyRingCollection coll,
String userId) {
List matchingPublicKeyRings =
getMatchingPublicKeyRings(coll, userId);
if (matchingPublicKeyRings.isEmpty()) {
return Optional.empty();
}
if (matchingPublicKeyRings.size() == 1) {
return Optional.of(matchingPublicKeyRings.get(0));
}
String message = String.format("Found %d matching public key rings for user ID \"%s\".",
matchingPublicKeyRings.size(),
userId);
throw new IllegalArgumentException(message);
}
private static List getMatchingPublicKeyRings(PGPPublicKeyRingCollection coll,
String userId) {
try {
List publicKeyRings = new LinkedList<>();
coll.getKeyRings(userId).forEachRemaining(publicKeyRings::add);
return publicKeyRings;
} catch (PGPException ex) {
throw new CryptoException(ex);
}
}
public static Optional findSecretKeyRing(PGPSecretKeyRingCollection coll,
String userId) {
List matchingSecretKeyRings =
getMatchingSecretKeyRings(coll, userId);
if (matchingSecretKeyRings.isEmpty()) {
return Optional.empty();
}
if (matchingSecretKeyRings.size() == 1) {
return Optional.of(matchingSecretKeyRings.get(0));
}
String message = String.format("Found %d matching secret key rings for user ID \"%s\".",
matchingSecretKeyRings.size(),
userId);
throw new IllegalArgumentException(message);
}
private static List getMatchingSecretKeyRings(PGPSecretKeyRingCollection coll,
String userId) {
try {
List secretKeyRings = new LinkedList<>();
coll.getKeyRings(userId, true).forEachRemaining(secretKeyRings::add);
return secretKeyRings;
} catch (PGPException ex) {
throw new CryptoException(ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy