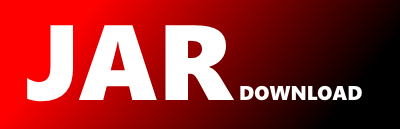
nom.tam.util.ColumnTable Maven / Gradle / Ivy
Go to download
Java library for reading and writing FITS files. FITS, the Flexible Image Transport System, is the format commonly used in the archiving and transport of astronomical data.
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy