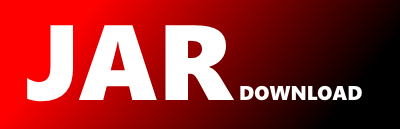
gov.nasa.pds.citool.ingestor.CatalogObject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of catalog-legacy Show documentation
Show all versions of catalog-legacy Show documentation
The Legacy Catalog Tool provides functionality for ingesting PDS3 catalog files into the PDS4 infrastructure including the Registry Service.
package gov.nasa.pds.citool.ingestor;
import java.net.MalformedURLException;
import java.io.File;
import java.util.List;
import java.util.ArrayList;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import java.util.Iterator;
import java.text.SimpleDateFormat;
import gov.nasa.pds.tools.label.Label;
import gov.nasa.pds.tools.label.Scalar;
import gov.nasa.pds.tools.label.Set;
import gov.nasa.pds.tools.label.Sequence;
import gov.nasa.pds.tools.label.ObjectStatement;
import gov.nasa.pds.tools.label.AttributeStatement;
import gov.nasa.pds.tools.label.PointerStatement;
import gov.nasa.pds.tools.label.Value;
import gov.nasa.pds.tools.containers.FileReference;
import gov.nasa.pds.citool.report.IngestReport;
import gov.nasa.pds.citool.util.References;
import gov.nasa.pds.citool.file.FileObject;
import gov.nasa.pds.citool.file.MD5Checksum;
import gov.nasa.pds.citool.registry.model.Metadata;
import gov.nasa.pds.citool.registry.model.RegistryObject;
/**
* Class to parse a PDS catalog file
*
* @author hlee
*/
public class CatalogObject {
private final static String[] CAT_OBJ_TYPES = { "MISSION",
"INSTRUMENT_HOST", "INSTRUMENT", "DATA_SET", "REFERENCE",
"PERSONNEL", "TARGET", "VOLUME", "DATA_SET_HOUSEKEEPING",
"DATA_SET_RELEASE", "SOFTWARE", "DATA_SET_MAP_PROJECTION" };
private String _catObjType;
private boolean _isLocal;
private IngestReport _report;
private Label _label;
private Map _pdsLabelMap;
private FileObject _fileObj;
private List _pointerFiles;
private String _filename;
private float _version;
private RegistryObject _product;
private Metadata _metadata;
private List _resrcObjs = null;
private Map _targetInfos;
public CatalogObject(IngestReport report)
{
this._report = report;
this._catObjType = null;
this._label = null;
this._fileObj = null;
this._pointerFiles = null;
this._isLocal = true;
this._filename = null;
this._version = 1.0f;
this._product = null;
this._metadata = null;
}
public String getFilename() {
return this._filename;
}
public Label getLabel() {
return this._label;
}
public Map getTargetInfos() {
return _targetInfos;
}
public String getCatObjType() {
return _catObjType;
}
public void setIsLocal(boolean local) {
this._isLocal = local;
}
public boolean getIsLocal() {
return this._isLocal;
}
public void setVersion(float version) {
this._version = version;
}
public float getVersion() {
return this._version;
}
public Metadata getMetadata() {
return this._metadata;
}
/**
* Set a file object
*/
public void setFileObject() {
try {
File product = new File(_label.getLabelURI().toURL().getFile());
SimpleDateFormat format = new SimpleDateFormat(
"yyyy-MM-dd'T'HH:mm:ss.SSSS'Z'");
String lastModified = format.format(new Date(product.lastModified()));
// Create a file object of the label file
_fileObj = new FileObject(product.getName(),
product.getParent(), product.length(),
lastModified, MD5Checksum.getMD5Checksum(product.toString()));
} catch (Exception e) {
e.printStackTrace();
}
}
public FileObject getFileObject() {
return this._fileObj;
}
public List getPointerFiles() {
return this._pointerFiles;
}
public RegistryObject getExtrinsicObject() {
return this._product;
}
public void setExtrinsicObject(RegistryObject product) {
this._product = product;
}
public List getResrcObjs() {
return this._resrcObjs;
}
/**
* Gets the catalog object from the LIST, sets to hash map
*
* @param objList
* List of the catalog object statement(s)
* @param pdsLabelMap
* Hashmap of the PDS label keyword and value for all ATTRIBUTE
*/
protected Map getCatalogObj(
List objList,
Map pdsLabelMap) {
String objType = this._catObjType;
Map lblMap = null;
// first level catalog object
for (ObjectStatement objSmt : objList) {
//System.out.println("CATALOG object type = " + objType);
if (objType.equalsIgnoreCase("VOLUME")) {
List objList2 = objSmt.getObjects();
for (ObjectStatement objSmt2: objList2) {
// how to handle multiple CATALOG objects???? or DATA_PRODUCER
if (objSmt2.getIdentifier().toString().equalsIgnoreCase("CATALOG")) {
List ptList = objSmt2.getPointers();
_pointerFiles = new ArrayList();
for (PointerStatement ptSmt : ptList) {
if (ptSmt.hasMultipleReferences()) {
List refFiles = ptSmt.getFileRefs();
for (FileReference fileRef : refFiles) {
_pointerFiles.add(fileRef.getPath());
}
} else {
_pointerFiles.add(ptSmt.getValue().toString());
}
}
}
}
}
List attrList = objSmt.getAttributes();
String keyId = null;
String keyDesc = null;
for (AttributeStatement attrSmt : attrList) {
pdsLabelMap.put(attrSmt.getElementIdentifier(), attrSmt);
//System.out.println(attrSmt.getElementIdentifier() + " = " + attrSmt.getValue().toString());
// multivalues
if (attrSmt.getValue() instanceof Set) {
List valueList = getValueList(attrSmt.getValue());
for (int i=0; i();
// second nested level
List objList2 = objSmt.getObjects();
for (ObjectStatement smt2 : objList2) {
List objAttr = smt2.getAttributes();
lblMap = new HashMap(pdsLabelMap);
//System.out.println("2nd object name = " + smt2.getIdentifier());
if (objType.equalsIgnoreCase("DATA_SET_HOUSEKEEPING") &&
smt2.getIdentifier().toString().equals("RESOURCE_INFORMATION")) {
_resrcObjs.add(smt2);
}
// TODO: how to handle same keywords in different
// object?????
for (AttributeStatement attrSmt : objAttr) {
lblMap.put(attrSmt.getElementIdentifier(), attrSmt);
//System.out.println("attrSmt.getElementIdentifier() = " + attrSmt.getElementIdentifier());
if (attrSmt.getElementIdentifier().toString().equals("TARGET_TYPE")) {
String targetName = objSmt.getAttribute("TARGET_NAME").getValue().toString();
//System.out.println("target type = " + attrSmt.getValue() + " target name = " + targetName);
_targetInfos.put(targetName, attrSmt.getValue().toString());
}
if (attrSmt.getValue() instanceof Set) {
List valueList = getValueList(attrSmt.getValue());
for (int i=0; i objList3 = smt2.getObjects();
for (ObjectStatement smt3 : objList3) {
List objAttr2 = smt3.getAttributes();
//System.out.println("3rd object name = " + smt3.getIdentifier());
for (AttributeStatement attrSmt2 : objAttr2) {
lblMap.put(attrSmt2.getElementIdentifier(), attrSmt2);
//System.out.println(attrSmt2.getElementIdentifier() + " = " + attrSmt2.getValue().toString());
if (attrSmt2.getValue() instanceof Set) {
List valueList = getValueList(attrSmt2.getValue());
for (int i=0; i(pdsLabelMap);
pdsLabelMap = lblMap;
}
}
}
return pdsLabelMap;
}
/**
* Converts a label object to List type
*
* @param label
* Label object
*/
protected List object2List(Label label) {
List objList = null;
for (int i = 0; i < CAT_OBJ_TYPES.length; i++) {
if (label.getObjects(CAT_OBJ_TYPES[i]).size() > 0) {
_catObjType = CAT_OBJ_TYPES[i];
objList = label.getObjects(CAT_OBJ_TYPES[i]);
}
}
return objList;
}
private boolean isValidCatalogFile(String objType) {
for (int i=0; i getPdsLabelMap() {
return this._pdsLabelMap;
}
/**
* Processes the given label and converts attributes into the hashmap
* object.
*
* @param label Label object
*/
public boolean processLabel(Label label) {
this._label = label;
boolean isValid = true;
try {
this._filename = label.getLabelURI().toURL().getFile();
this._metadata = new Metadata();
List attrList = label.getAttributes();
_pdsLabelMap = new HashMap();
_targetInfos = new HashMap();
for (Iterator i = attrList.iterator(); i.hasNext();) {
AttributeStatement attrSmt = (AttributeStatement) i.next();
_pdsLabelMap.put(attrSmt.getElementIdentifier(), attrSmt);
_metadata.addMetadata(attrSmt.getElementIdentifier(), attrSmt.getValue().toString());
}
List objLists = label.getObjects();
for (Iterator i=objLists.iterator(); i.hasNext();) {
ObjectStatement objSmt = (ObjectStatement) i.next();
isValid = isValidCatalogFile(objSmt.getIdentifier().toString());
}
if (isValid) {
List objList = object2List(label);
//System.out.println("objList = " + objList.toString());
if (objList!=null) {
_pdsLabelMap = getCatalogObj(objList, _pdsLabelMap);
}
}
} catch (MalformedURLException mue) {
mue.printStackTrace();
} catch (Exception ex) {
ex.printStackTrace();
}
return isValid;
}
/**
* Determine the given string value is a multivalued or not
*
* @return list of string value if the given value is multivalued (surrounding within { ... })
* otherwise, null value is returned.
*/
public static List getValueList(Value value) {
List valueList = new ArrayList();
if (value instanceof Set) {
Iterator it = ((Set) value).iterator();
while (it.hasNext()) {
final String strValue = it.next().toString();
valueList.add(strValue);
}
}
else if (value instanceof Sequence) {
// TODO: add algorithm here
}
else
return null;
return valueList;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy