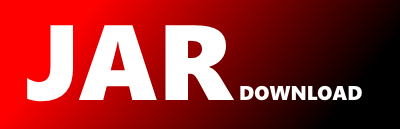
gov.nasa.pds.citool.logging.IngestFormatter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of catalog-legacy Show documentation
Show all versions of catalog-legacy Show documentation
The Legacy Catalog Tool provides functionality for ingesting PDS3 catalog files into the PDS4 infrastructure including the Registry Service.
package gov.nasa.pds.citool.logging;
import gov.nasa.pds.citool.logging.ToolsLevel;
import gov.nasa.pds.citool.logging.ToolsLogRecord;
import java.util.ArrayList;
import java.util.List;
import java.util.logging.Formatter;
import java.util.logging.Handler;
import java.util.logging.LogRecord;
/**
* Class to format the report when running the Catalog Ingestion Tool
* to ingest catalog files.
*
* @author hyunlee
*
*/
public class IngestFormatter extends Formatter {
private List records;
private StringBuffer config;
private StringBuffer parameters;
private StringBuffer body;
private StringBuffer summary;
private boolean headerPrinted;
private static String lineFeed = System.getProperty("line.separator", "\n");
private static String doubleLineFeed = System.getProperty("line.separator", "\n") + System.getProperty("line.separator", "\n");
public IngestFormatter() {
records = new ArrayList();
config = new StringBuffer();
headerPrinted = false;
parameters = new StringBuffer("Parameter Settings:" + doubleLineFeed);
summary = new StringBuffer("Summary:" + doubleLineFeed);
body = new StringBuffer("Catalog Ingestion Details:" + lineFeed);
}
public String format(LogRecord record) {
ToolsLogRecord toolsRecord = (ToolsLogRecord) record;
if (toolsRecord.getLevel() == ToolsLevel.CONFIGURATION) {
config.append(" " + toolsRecord.getMessage() + lineFeed);
}
else if (toolsRecord.getLevel() == ToolsLevel.PARAMETER) {
parameters.append(" " + toolsRecord.getMessage() + lineFeed);
}
else
return processRecords(toolsRecord);
return "";
}
private String processRecords(ToolsLogRecord record) {
body.append(lineFeed + " " + record.getMessage());
records = new ArrayList();
return "";
}
private void processSummary() {
summary.append(" Catalog Ingestion is completed." + lineFeed);
//summary.append(" Number of processed files: " + gov.nasa.pds.citool.ingestor.Ingestor.fileCount + lineFeed);
summary.append(" Number of successful ingestion to the table: " + gov.nasa.pds.citool.ingestor.CatalogDB.okCount + lineFeed);
summary.append(" Number of failed ingestion to the table: " + gov.nasa.pds.citool.ingestor.CatalogDB.failCount + lineFeed);
//summary.append(" Number of new standard values ingested: " + gov.nasa.pds.citool.ingestor.CatalogDB.newStdValueCount + lineFeed);
}
public String getTail(Handler handler) {
StringBuffer report = new StringBuffer("");
processSummary();
report.append(doubleLineFeed + "PDS Catalog Ingestion Tool Report" + doubleLineFeed);
report.append(config);
report.append(lineFeed);
report.append(parameters);
report.append(lineFeed);
report.append(summary);
report.append(lineFeed);
report.append(body);
report.append(doubleLineFeed + "End of Report" + doubleLineFeed);
return report.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy