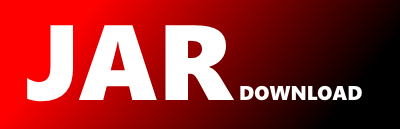
gov.nasa.pds.registry.common.connection.aws.SearchRespWrap Maven / Gradle / Ivy
package gov.nasa.pds.registry.common.connection.aws;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.commons.lang3.NotImplementedException;
import org.opensearch.client.opensearch.OpenSearchClient;
import org.opensearch.client.opensearch._types.Time;
import org.opensearch.client.opensearch._types.aggregations.StringTermsBucket;
import org.opensearch.client.opensearch.core.ScrollRequest;
import org.opensearch.client.opensearch.core.ScrollResponse;
import org.opensearch.client.opensearch.core.SearchResponse;
import org.opensearch.client.opensearch.core.search.Hit;
import gov.nasa.pds.registry.common.Response;
import gov.nasa.pds.registry.common.es.dao.dd.LddInfo;
import gov.nasa.pds.registry.common.es.dao.dd.LddVersions;
@SuppressWarnings("unchecked") // JSON heterogenous structures requires raw casting
class SearchRespWrap implements Response.Search {
final private OpenSearchClient client;
final private SearchResponse
© 2015 - 2025 Weber Informatics LLC | Privacy Policy