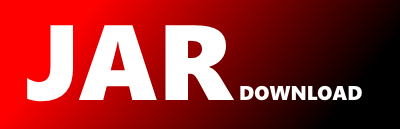
gov.nasa.pds.registry.common.connection.aws.CreateIndexConfigWrap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of registry-common Show documentation
Show all versions of registry-common Show documentation
Common code used by Harvest and Registry Manager.
The newest version!
package gov.nasa.pds.registry.common.connection.aws;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.opensearch.client.opensearch._types.analysis.Analyzer;
import org.opensearch.client.opensearch._types.analysis.CustomAnalyzer;
import org.opensearch.client.opensearch._types.mapping.DynamicMapping;
import org.opensearch.client.opensearch._types.mapping.DynamicTemplate;
import org.opensearch.client.opensearch._types.mapping.Property;
import org.opensearch.client.opensearch._types.mapping.TextProperty;
import org.opensearch.client.opensearch._types.mapping.TypeMapping;
import org.opensearch.client.opensearch.indices.CreateIndexRequest;
import org.opensearch.client.opensearch.indices.IndexSettings;
import org.opensearch.client.opensearch.indices.IndexSettingsAnalysis;
import org.opensearch.client.opensearch.indices.IndexSettingsMapping;
import org.opensearch.client.opensearch.indices.IndexSettingsMappingLimit;
import com.google.gson.Gson;
@SuppressWarnings("unchecked") // evil but necessary because of JSON heterogeneous structures
class CreateIndexConfigWrap {
static CreateIndexRequest.Builder update (CreateIndexRequest.Builder builder, String withJsonConfig) {
Map config = (Map)new Gson().fromJson(withJsonConfig, Object.class);
for (String pk : config.keySet()) {
if (pk.equalsIgnoreCase("mappings")) updateMappings (builder, (Map)config.get(pk));
else if (pk.equalsIgnoreCase("settings")) updateSettings (builder, (Map)config.get(pk));
else throw new RuntimeException("Unknown config key '" + pk + "' requiring fix to JSON or CreateIndexConfigWrap.update()");
}
return builder;
}
private static void updateAnalysis (IndexSettings.Builder builder, Map analysis) {
IndexSettingsAnalysis.Builder craftsman = new IndexSettingsAnalysis.Builder();
for (String pk : analysis.keySet()) {
if (pk.equalsIgnoreCase("normalizer")) {
Analyzer.Builder journeyman = new Analyzer.Builder();
Map analyzers = (Map)analysis.get(pk);
for (String ak : analyzers.keySet()) {
Map analyzer = (Map)analyzers.get(ak);
String atype = (String)analyzer.get("type");
if (atype.equalsIgnoreCase("custom"))
journeyman.custom(new CustomAnalyzer.Builder().filter((List)analyzer.get("filter")).tokenizer(ak.equalsIgnoreCase("keyword_lowercase") ? "keyword" : ak).build());
else throw new RuntimeException("Unknown analyzer type '" + atype + "' requiring fix to JSON or CreateIndexConfigWrap.updateAnalysis()");
}
craftsman.analyzer(pk, journeyman.build());
}
else throw new RuntimeException("Unknown analysis key '" + pk + "' requiring fix to JSON or CreateIndexConfigWrap.updateAnalysis()");
}
builder.analysis(craftsman.build());
}
private static void updateMappings (CreateIndexRequest.Builder builder, Map mappings) {
TypeMapping.Builder craftsman = new TypeMapping.Builder();
for (String pk : mappings.keySet()) {
if (pk.equalsIgnoreCase("dynamic")) craftsman.dynamic((Boolean)mappings.get(pk) ? DynamicMapping.True : DynamicMapping.False);
else if (pk.equalsIgnoreCase("dynamic_templates")) {
ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy