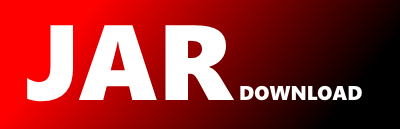
gov.nasa.pds.registry.common.connection.aws.MGetRespWrap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of registry-common Show documentation
Show all versions of registry-common Show documentation
Common code used by Harvest and Registry Manager.
The newest version!
package gov.nasa.pds.registry.common.connection.aws;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import org.opensearch.client.opensearch.core.MgetResponse;
import org.opensearch.client.opensearch.core.mget.MultiGetResponseItem;
import gov.nasa.pds.registry.common.es.dao.dd.DataTypeNotFoundException;
import gov.nasa.pds.registry.common.util.Tuple;
class MGetRespWrap extends GetRespWrap {
final private MgetResponse
© 2015 - 2025 Weber Informatics LLC | Privacy Policy