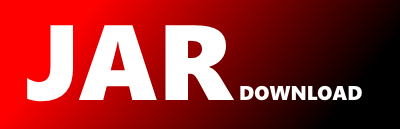
bin.registry-mgr Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of registry Show documentation
Show all versions of registry Show documentation
The Registry provides provides the PDS-specific search protocol and the search capability for the PDS search index generated through the Search Core software. The core functionality for this service is satisfied by Apache Solr.
The newest version!
#!/usr/bin/env bash
# Copyright 2019, California Institute of Technology ("Caltech").
# U.S. Government sponsorship acknowledged.
#
# All rights reserved.
#
# Redistribution and use in source and binary forms, with or without
# modification, are permitted provided that the following conditions are met:
#
# * Redistributions of source code must retain the above copyright notice,
# this list of conditions and the following disclaimer.
# * Redistributions must reproduce the above copyright notice, this list of
# conditions and the following disclaimer in the documentation and/or other
# materials provided with the distribution.
# * Neither the name of Caltech nor its operating division, the Jet Propulsion
# Laboratory, nor the names of its contributors may be used to endorse or
# promote products derived from this software without specific prior written
# permission.
#
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
# AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
# IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
# ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
# LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
# CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
# SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
# INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
# CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
# ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
# POSSIBILITY OF SUCH DAMAGE.
DATA_COLLECTION="data"
ALL_COLLECTIONS="registry xpath data"
PROPS=("-Dauto=yes")
RECURSIVE=""
FILES=()
DELETE=
SCRIPT_DIR=$(cd "$( dirname $0 )" && pwd)
PARENT_DIR=$(cd ${SCRIPT_DIR}/.. && pwd)
LIB_DIR=${PARENT_DIR}/lib
TOOL_JAR=(${LIB_DIR}/solr-core-*.jar)
function print_usage() {
echo ""
echo "Usage: $0 [OPTIONS] [SOLR-DOCS]"
echo ""
echo "Options:"
echo " -h Print Help"
echo " -host Solr server host (default: localhost)"
echo " -port Solr server port (default: 8983)"
echo " -delete-pkg Delete a specific Harvest ingestion package"
echo " -delete-all Delete all data from all Registry collections"
echo ""
echo "Optional Parameters:"
echo " SOLR-DOCS solr-docs directory or an xml file"
echo ""
}
# Parse command line parameters
while [ $# -gt 0 ]; do
if [[ -d "$1" ]]; then
# Directory
RECURSIVE=yes
FILES+=("$1")
elif [[ -f "$1" ]]; then
# File
FILES+=("$1")
else
if [[ "$1" == "-port" ]]; then
shift
PROPS+=("-Dport=$1")
elif [[ "$1" == "-host" ]]; then
shift
PROPS+=("-Dhost=$1")
elif [[ "$1" == "-delete-pkg" ]]; then
shift
DELETE="package_id:$1 "
break
elif [[ "$1" == "-delete-all" ]]; then
shift
DELETE="*:* "
break
elif [[ "$1" == "-h" ]]; then
print_usage
exit
else
echo -e "\nUnrecognized argument: $1\n"
exit 1
fi
fi
shift
done
# Setup Java
if [ -n "$JAVA_HOME" ]; then
JAVA="$JAVA_HOME/bin/java"
else
JAVA=java
fi
# Test that Java exists and is executable
"$JAVA" -version >/dev/null 2>&1 || { echo >&2 "Java is required to run this tool! Please install Java 8 or greater before running this script."; exit 1; }
# If delete request, let's delete and exit
if [ ! -z "$DELETE" ]; then
for collection in $ALL_COLLECTIONS; do
#PROPS=("-Dc=$collection" "-Ddata=files")
PROPS=("-Dc=$collection" "-Ddata=args")
echo "$JAVA" -classpath "${TOOL_JAR[0]}" "${PROPS[@]}" org.apache.solr.util.SimplePostTool ${DELETE}
"$JAVA" -classpath "${TOOL_JAR[0]}" "${PROPS[@]}" org.apache.solr.util.SimplePostTool ${DELETE}
done
rm -f $DELETE_FILE
exit 0
fi
# No file / directory parameter
if [[ "${#FILES[@]}" -eq 0 ]]; then
DEFAULT_DOCS_DIR=${HOME}/registry-data/solr-docs
echo "INFO: No SOLR-DOCS parameter (Use -h flag for help)."
echo "INFO: Looking for Solr documents in default location: ${DEFAULT_DOCS_DIR}"
if [ ! -e "${DEFAULT_DOCS_DIR}" ]; then
echo
echo "ERROR: ${DEFAULT_DOCS_DIR} does not exist." 1>&2
echo " Verify Registry installation completed successfully." 1>&2
echo
exit 1
fi
# Must be a directory
if [[ -d "${DEFAULT_DOCS_DIR}" ]]; then
RECURSIVE=yes
FILES+=("${DEFAULT_DOCS_DIR}")
else
echo "ERROR: ${DEFAULT_DOCS_DIR} must be a directory." 1>&2
exit 1
fi
echo "INFO: Found Solr documents in ${REGISTRY_DATA}"
fi
# Parameters for Solr post tool
PROPS+=("-Dc=$DATA_COLLECTION" "-Ddata=files")
if [[ -n "$RECURSIVE" ]]; then
PROPS+=("-Drecursive=yes")
fi
# Call Solr post tool
echo "$JAVA" -classpath "${TOOL_JAR[0]}" "${PROPS[@]}" org.apache.solr.util.SimplePostTool "${FILES[@]}"
"$JAVA" -classpath "${TOOL_JAR[0]}" "${PROPS[@]}" org.apache.solr.util.SimplePostTool "${FILES[@]}"
exit 0
© 2015 - 2025 Weber Informatics LLC | Privacy Policy