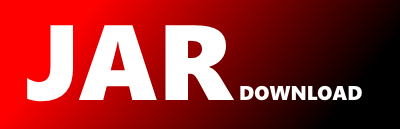
src.gov.nasa.worldwindx.examples.GeoRSS Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of worldwind Show documentation
Show all versions of worldwind Show documentation
World Wind is a collection of components that interactively display 3D geographic information within Java applications or applets.
/*
* Copyright (C) 2012 United States Government as represented by the Administrator of the
* National Aeronautics and Space Administration.
* All Rights Reserved.
*/
package gov.nasa.worldwindx.examples;
import gov.nasa.worldwind.formats.georss.GeoRSSParser;
import gov.nasa.worldwind.layers.RenderableLayer;
import gov.nasa.worldwind.render.Renderable;
/**
* Illustrates how to create a shape from a GeoRSS document. This example creates two shapes from hard-coded example
* GeoRSS documents.
*
* @author dcollins
* @version $Id: GeoRSS.java 1171 2013-02-11 21:45:02Z dcollins $
*/
public class GeoRSS extends ApplicationTemplate
{
private static class AppFrame extends ApplicationTemplate.AppFrame
{
public AppFrame()
{
super(true, true, false);
RenderableLayer layer = this.buildGeoRSSLayer();
layer.setName("GeoRSS Shapes");
insertBeforePlacenames(this.getWwd(), layer);
this.getLayerPanel().update(this.getWwd());
}
private RenderableLayer buildGeoRSSLayer()
{
RenderableLayer layer = new RenderableLayer();
java.util.List shapes;
shapes = GeoRSSParser.parseShapes(GeoRSS_DOCSTRING_A);
if (shapes != null)
addRenderables(layer, shapes);
shapes = GeoRSSParser.parseShapes(GeoRSS_DOCSTRING_B);
if (shapes != null)
addRenderables(layer, shapes);
return layer;
}
private void addRenderables(RenderableLayer layer, Iterable renderables)
{
for (Renderable r : renderables)
layer.addRenderable(r);
}
}
private static final String GeoRSS_DOCSTRING_A =
"" +
"" +
" Earthquakes " +
" International earthquake observation labs " +
" " +
" 2005-12-13T18:30:02Z " +
" " +
" Dr. Thaddeus Remor " +
" [email protected] " +
" " +
" urn:uuid:60a76c80-d399-11d9-b93C-0003939e0af6 " +
" " +
" M 3.2, Mona Passage " +
" " +
" urn:uuid:1225c695-cfb8-4ebb-aaaa-80da344efa6a " +
" 2005-08-17T07:02:32Z " +
" We just had a big one. " +
" " +
" " +
" " +
" " +
" " +
" 45.256 -110.45 46.46 -109.48 43.84 -109.86 45.256 -110.45" +
" " +
" " +
" " +
" " +
" " +
" " +
" ";
private static final String GeoRSS_DOCSTRING_B =
"" +
" scribble " +
" http://example.com/atom " +
" Christopher Schmidt " +
"" +
" http://example.com/19.atom " +
" " +
" Feature #19 " +
" Some content. " +
" " +
" 23.1811523438 -159.609375 " +
" 22.5 -161.564941406 " +
" 20.654296875 -160.422363281 " +
" 18.4350585938 -156.247558594 " +
" 18.3471679688 -154.731445312 " +
" 19.951171875 -153.588867188 " +
" 21.8188476562 -155.983886719" +
" 23.02734375 -158.994140625" +
" 23.0932617188 -159.631347656" +
" " +
" " +
" ";
public static void main(String[] args)
{
ApplicationTemplate.start("World Wind GeoRSS", AppFrame.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy