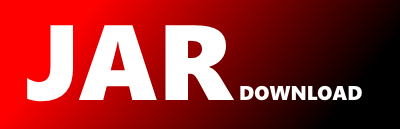
src.gov.nasa.worldwindx.examples.KeepingObjectsInView Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of worldwind Show documentation
Show all versions of worldwind Show documentation
World Wind is a collection of components that interactively display 3D geographic information within Java applications or applets.
/*
* Copyright (C) 2012 United States Government as represented by the Administrator of the
* National Aeronautics and Space Administration.
* All Rights Reserved.
*/
package gov.nasa.worldwindx.examples;
import gov.nasa.worldwind.*;
import gov.nasa.worldwind.animation.*;
import gov.nasa.worldwind.avlist.*;
import gov.nasa.worldwind.event.*;
import gov.nasa.worldwind.geom.*;
import gov.nasa.worldwind.globes.Globe;
import gov.nasa.worldwind.layers.*;
import gov.nasa.worldwind.render.*;
import gov.nasa.worldwind.render.airspaces.*;
import gov.nasa.worldwind.util.*;
import gov.nasa.worldwind.view.orbit.OrbitView;
import gov.nasa.worldwindx.examples.util.ExtentVisibilitySupport;
import javax.swing.*;
import javax.swing.Box;
import javax.swing.Timer;
import java.awt.*;
import java.awt.event.*;
import java.util.*;
/**
* KeepingObjectsInView demonstrates keeping a set of scene elements visible by using the utility class {@link
* gov.nasa.worldwindx.examples.util.ExtentVisibilitySupport}. To run this demonstration, execute this class' main
* method, then follow the on-screen instructions.
*
* The key functionality demonstrated by KeepingObjectsVisible is found in the internal classes {@link
* KeepingObjectsInView.ViewController} and {@link gov.nasa.worldwindx.examples.KeepingObjectsInView.ViewAnimator}.
*
* @author dcollins
* @version $Id: KeepingObjectsInView.java 1171 2013-02-11 21:45:02Z dcollins $
*/
public class KeepingObjectsInView extends ApplicationTemplate
{
public static class AppFrame extends ApplicationTemplate.AppFrame
{
protected Iterable> objectsToTrack;
protected ViewController viewController;
protected RenderableLayer helpLayer;
public AppFrame()
{
// Create an iterable of the objects we want to keep in view.
this.objectsToTrack = createObjectsToTrack();
// Set up a view controller to keep the objects in view.
this.viewController = new ViewController(this.getWwd());
this.viewController.setObjectsToTrack(this.objectsToTrack);
// Set up a layer to render the objects we're tracking.
this.addObjectsToWorldWindow(this.objectsToTrack);
// Set up swing components to toggle the view controller's behavior.
this.initSwingComponents();
// Set up a one-shot timer to zoom to the objects once the app launches.
Timer timer = new Timer(1000, new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
enableHelpAnnotation();
viewController.gotoScene();
}
});
timer.setRepeats(false);
timer.start();
}
protected void enableHelpAnnotation()
{
if (this.helpLayer != null)
return;
this.helpLayer = new RenderableLayer();
this.helpLayer.addRenderable(createHelpAnnotation(getWwd()));
insertBeforePlacenames(this.getWwd(), this.helpLayer);
}
protected void disableHelpAnnotation()
{
if (this.helpLayer == null)
return;
this.getWwd().getModel().getLayers().remove(this.helpLayer);
this.helpLayer.removeAllRenderables();
this.helpLayer = null;
}
protected void addObjectsToWorldWindow(Iterable> objectsToTrack)
{
// Set up a layer to render the icons. Disable WWIcon view clipping, since view tracking works best when an
// icon's screen rectangle is known even when the icon is outside the view frustum.
IconLayer iconLayer = new IconLayer();
iconLayer.setViewClippingEnabled(false);
iconLayer.setName("Icons To Track");
insertBeforePlacenames(this.getWwd(), iconLayer);
// Set up a layer to render the markers.
RenderableLayer shapesLayer = new RenderableLayer();
shapesLayer.setName("Shapes to Track");
insertBeforePlacenames(this.getWwd(), shapesLayer);
this.getLayerPanel().update(this.getWwd());
// Add the objects to track to the layers.
for (Object o : objectsToTrack)
{
if (o instanceof WWIcon)
iconLayer.addIcon((WWIcon) o);
else if (o instanceof Renderable)
shapesLayer.addRenderable((Renderable) o);
}
// Set up a SelectListener to drag the spheres.
this.getWwd().addSelectListener(new SelectListener()
{
protected BasicDragger dragger = new BasicDragger(getWwd());
public void selected(SelectEvent event)
{
// Delegate dragging computations to a dragger.
this.dragger.selected(event);
if (event.getEventAction().equals(SelectEvent.DRAG))
{
disableHelpAnnotation();
viewController.sceneChanged();
}
}
});
}
protected void initSwingComponents()
{
// Create a checkbox to enable/disable the view controller.
JCheckBox checkBox = new JCheckBox("Enable view tracking", true);
checkBox.setAlignmentX(Component.LEFT_ALIGNMENT);
checkBox.addActionListener(new ActionListener()
{
public void actionPerformed(ActionEvent event)
{
boolean selected = ((AbstractButton) event.getSource()).isSelected();
viewController.setEnabled(selected);
}
});
JButton button = new JButton("Go to objects");
button.setAlignmentX(Component.LEFT_ALIGNMENT);
button.addActionListener(new ActionListener()
{
public void actionPerformed(ActionEvent event)
{
viewController.gotoScene();
}
});
Box box = Box.createVerticalBox();
box.setBorder(BorderFactory.createEmptyBorder(30, 30, 30, 30)); // top, left, bottom, right
box.add(checkBox);
box.add(Box.createVerticalStrut(5));
box.add(button);
this.getLayerPanel().add(box, BorderLayout.SOUTH);
}
}
public static Iterable> createObjectsToTrack()
{
ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy