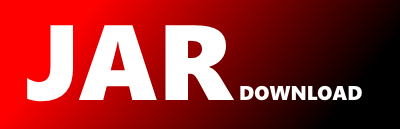
gov.nih.nlm.nls.lvg.Db.DbDerivation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lvg2010dist Show documentation
Show all versions of lvg2010dist Show documentation
LVG tools is used by Apache cTAKES.
The newest version!
package gov.nih.nlm.nls.lvg.Db;
import java.sql.*;
import java.util.*;
import gov.nih.nlm.nls.lvg.Lib.*;
/*****************************************************************************
* This class provides high level interfaces to derivation table in LVG database.
*
* History:
*
*
*
* @author NLM NLS Development Team
*
* @see DerivationRecord
* @see
*
* Desgin Document
*
* @version V-2010
****************************************************************************/
public class DbDerivation
{
/**
* Get all derivation records for a specific term (lower case)
* from LVG database.
*
* @param inStr term for derivations (lower case)
* @param conn database connection
*
* @return all derivation records for the specified term, inStr
*
* @exception SQLException if there is a database error happens
*/
public static Vector GetDerivations(String inStr,
Connection conn) throws SQLException
{
Vector derivations = new Vector();
// Forward
String query = "SELECT term1, cat1, term2, cat2 FROM Derivation "
+ "WHERE termLc1= ?";
PreparedStatement ps = conn.prepareStatement(query);
ps.setString(1, inStr.toLowerCase());
// get data from table derivation
ResultSet rs = ps.executeQuery();
while(rs.next())
{
DerivationRecord derivationRecord = new DerivationRecord(
rs.getString(1), // term1
rs.getInt(2), // cat1
rs.getString(3), // term2
rs.getInt(4)); // cat2
derivations.addElement(derivationRecord);
}
// Clean up
rs.close();
ps.close();
// Backward
String query2 = "SELECT term2, cat2, term1, cat1 FROM Derivation "
+ "WHERE termLc2= ?";
PreparedStatement ps2 = conn.prepareStatement(query2);
ps2.setString(1, inStr.toLowerCase());
// get data from table derivation
ResultSet rs2 = ps2.executeQuery();
while(rs2.next())
{
DerivationRecord derivationRecord = new DerivationRecord(
rs2.getString(1), // term2
rs2.getInt(2), // cat2
rs2.getString(3), // term1
rs2.getInt(4)); // cat1
derivations.addElement(derivationRecord);
}
// Clean up
rs.close();
ps2.close();
return derivations;
}
/**
* Test driver for this class.
*/
public static void main (String[] args)
{
String testStr = "multiple";
if(args.length == 1)
{
testStr = args[0];
}
// read in configuration file
Configuration conf = new Configuration("data.config.lvg", true);
// obtain a connection
try
{
Connection conn = DbBase.OpenConnection(conf);
if(conn != null)
{
// Get Derivations
Vector derivationList
= GetDerivations(testStr, conn);
System.out.println("----- Total Derivations found: " +
derivationList.size());
for(int i = 0; i < derivationList.size(); i++)
{
if(i == 0)
{
System.out.println("=== Found Derivations ===");
}
DerivationRecord record = derivationList.elementAt(i);
System.out.println(record.GetSource() + "|" +
record.GetSourceCat() + "|" + record.GetTarget() + "|" +
record.GetTargetCat());
}
DbBase.CloseConnection(conn, conf);
}
}
catch (SQLException sqle)
{
System.err.println(sqle.getMessage());
}
catch (Exception e)
{
System.err.println(e.getMessage());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy