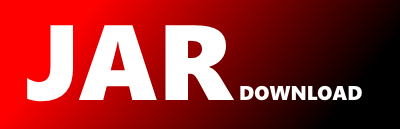
gov.nih.nlm.nls.lvg.Db.DbSpellingVariants Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lvg2010dist Show documentation
Show all versions of lvg2010dist Show documentation
LVG tools is used by Apache cTAKES.
The newest version!
package gov.nih.nlm.nls.lvg.Db;
import java.sql.*;
import java.util.*;
import gov.nih.nlm.nls.lvg.Lib.*;
/*****************************************************************************
* This class provides high level interfaces to check spelling variants from
* Inflection table in LVG database.
*
* History:
*
*
*
* @author NLM NLS Development Team
*
* @see
* Desgin Document
*
* @version V-2010
****************************************************************************/
public class DbSpellingVariants
{
/**
* Get all spelling variant from inflection records for a specified term
* from LVG database.
*
* @param inStr term for finding spellng variants
* @param conn database connection
*
* @return all acronym records with key form is same as inStr
*
* @exception SQLException if there is a database error happens
*/
public static Vector GetSpellingVariants(String inStr,
Connection conn) throws SQLException
{
Vector records =
DbInflectionUtil.GetRecordsByIfTerm(inStr, conn, false);
// eliminate duplicate recrods with same EUI, Cat, Infl
Vector pureRecords
= EliminateDuplicateRecords(records);
Vector svRecords
= GetInflectionsByEuiCatInf(pureRecords, conn);
// Sort
SpellingVarComparator sc
= new SpellingVarComparator();
Collections.sort(svRecords, sc);
return svRecords;
}
// private methods
// Eliminate records with same EUI, Category, and Inflection
private static Vector EliminateDuplicateRecords(
Vector ins)
{
Vector outs = new Vector();
for(int i = 0; i < ins.size(); i++)
{
InflectionRecord record = ins.elementAt(i);
if(IsContainRecord(outs, record) == false)
{
outs.addElement(record);
}
}
return outs;
}
// check if it contains an element
private static boolean IsContainRecord(Vector records,
InflectionRecord record)
{
// check if the records is empty
if(record == null)
{
return false;
}
for(int i = 0; i < records.size(); i++)
{
InflectionRecord cur = records.elementAt(i);
if((record.GetEui().equals(cur.GetEui()))
&& (record.GetCategory() == cur.GetCategory())
&& (record.GetInflection() == cur.GetInflection()))
{
return true;
}
}
return false;
}
// multiple input inflection records
private static Vector GetInflectionsByEuiCatInf(
Vector ins, Connection conn) throws SQLException
{
Vector out = new Vector();
for(int i = 0; i < ins.size(); i++)
{
InflectionRecord record = ins.elementAt(i);
Vector temp
= GetInflectionsByEuiCatInf(record, conn);
out.addAll(temp);
}
return out;
}
// one input inflection record
private static Vector GetInflectionsByEuiCatInf(
InflectionRecord in, Connection conn) throws SQLException
{
String query = "SELECT ifTerm, termCat, termInfl, eui, unTerm, ctTerm "
+ "FROM Inflection WHERE eui = ? AND termCat = ? AND termInfl = ?";
PreparedStatement ps = conn.prepareStatement(query);
ps.setString(1, in.GetEui());
ps.setInt(2, in.GetCategory());
ps.setLong(3, in.GetInflection());
return DbInflectionUtil.GetRecordsByPreparedStatement(ps, false);
}
/**
* Test driver for this class
*/
public static void main (String[] args)
{
Configuration conf = new Configuration("data.config.lvg", true);
String testStr = "color";
if(args.length == 1)
{
testStr = args[0];
}
// obtain a connection
try
{
Connection conn = DbBase.OpenConnection(conf);
if(conn != null)
{
// test for inflection terms
Vector records =
GetSpellingVariants(testStr, conn);
System.out.println("----- Total records found: " +
records.size());
for(int i = 0; i < records.size(); i++)
{
InflectionRecord record = records.elementAt(i);
System.out.println(record.GetInflectedTerm() + "|"
+ record.GetUninflectedTerm() + "|"
+ record.GetCitationTerm() + "|"
+ record.GetEui() + "|"
+ record.GetCategory() + "|"
+ record.GetInflection());
}
DbBase.CloseConnection(conn, conf);
}
}
catch (SQLException sqle)
{
System.err.println(sqle.getMessage());
}
catch (Exception e)
{
System.err.println(e.getMessage());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy