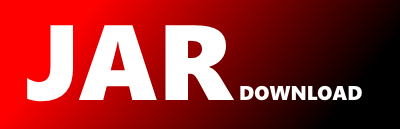
gov.nih.nlm.nls.lvg.Flows.ToRecursiveDerivations Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lvg2010dist Show documentation
Show all versions of lvg2010dist Show documentation
LVG tools is used by Apache cTAKES.
The newest version!
package gov.nih.nlm.nls.lvg.Flows;
import java.util.*;
import java.sql.*;
import gov.nih.nlm.nls.lvg.Lib.*;
import gov.nih.nlm.nls.lvg.Util.*;
import gov.nih.nlm.nls.lvg.Db.*;
import gov.nih.nlm.nls.lvg.Trie.*;
/*****************************************************************************
* This class generates derivations from the input term, recursively, until there
* are no more, or until a cycle is detected.
*
* History:
*
*
*
* @author NLM NLS Development Team
*
* @see
* Design Document
* @see ToDerivation
*
* @version V-2010
****************************************************************************/
public class ToRecursiveDerivations extends Transformation implements Cloneable
{
// public methods
/**
* Performs the mutation of this flow component.
*
* @param in a LexItem as the input for this flow component
* @param conn LVG database connection
* @param trie LVG Ram trie
* @param restrictFlag a numberical flag to restrict out into LVG_ONLY
* LVG_OR_ALL, or ALL (defined in OutputFilter).
* @param detailsFlag a boolean flag for processing details information
* @param mutateFlag a boolean flag for processing mutate information
* @param detailFlowFlag a boolean flag for showing the flow history in
* details. For instance use ddd instead of R.
*
* @return Vector - results from this flow component
*
* @see DbBase
* @see OutputFilter
*/
public static Vector Mutate(LexItem in, Connection conn,
RamTrie trie, int restrictFlag, boolean detailsFlag,
boolean mutateFlag, boolean detailFlowFlag)
{
// Mutate:
Init(in.GetSourceTerm());
GetRecursiveDerivations(in, conn, trie, restrictFlag, INFO, true,
detailsFlag, mutateFlag, null);
UpdateFlowHistory(detailFlowFlag); // remove the original term
return GetDerivationVector();
}
/**
* A unit test driver for this flow component.
*/
public static void main(String[] args)
{
// load config file
Configuration conf = new Configuration("data.config.lvg", true);
String testStr = GetTestStr(args, "medicine"); // get input String
int minTermLen = Integer.parseInt(
conf.GetConfiguration(Configuration.MIN_TERM_LENGTH));
String lvgDir = conf.GetConfiguration(Configuration.LVG_DIR);
int minTrieStemLength = Integer.parseInt(
conf.GetConfiguration(Configuration.DIR_TRIE_STEM_LENGTH));
// Mutate: connect to DB
LexItem in = new LexItem(testStr, Category.ALL_BIT_VALUE,
Inflection.ALL_BIT_VALUE);
Vector outs = new Vector();
try
{
Connection conn = DbBase.OpenConnection(conf);
boolean isInflection = false;
RamTrie trie = new RamTrie(isInflection, minTermLen, lvgDir,
minTrieStemLength);
if(conn != null)
{
outs = ToRecursiveDerivations.Mutate(in, conn, trie,
OutputFilter.LVG_ONLY, true, true, false);
}
DbBase.CloseConnection(conn, conf);
}
catch (Exception e)
{
System.err.println(e.getMessage());
}
PrintResults(in, outs); // print out results
}
// private method
/**
* Get derivational variants for a specified term by Lvg facts or rules.
*
* @param in a LexItem as the input for this flow component
* @param conn LVG database connection
* @param trie LVG Ram trie
* @param restrictFlag a numberical flag to restrict out into LVG_ONLY
* LVG_OR_ALL, or ALL (defined in OutputFilter).
* @param infoStr the header of detail information, usually is the
* full name of the current flo
* @param appendFlowHistory A boolean flag used to append flow history
* when it's value is true. This flag should be set to ture only for the
* very first time and then set to be false to avoid duplicate flow symbols
* in the flow history.
* @param detailsFlag a boolean flag for processing details information
* @param mutateFlag a boolean flag for processing mutate information
* @param rFlowHistory ccumulate flow history for recursive derivation
*
* @return the results from this flow component - a collection (Vector)
* of LexItems
*
* @see DbBase
* @see OutputFilter
*/
private static Vector GetDerivations(LexItem in, Connection conn,
RamTrie trie, int restrictFlag, String infoStr,
boolean appendFlowHistory, boolean detailsFlag, boolean mutateFlag,
String rFlowHistory)
{
String inStr = in.GetSourceTerm();
Vector outs = new Vector();
// check if input is nothing
if(in.GetSourceTerm().length() == 0)
{
return outs;
}
// update flow hsitory for details information
String flowName = rFlowHistory;
try
{
long inCat = in.GetSourceCategory().GetValue();
long inInfl = in.GetSourceInflection().GetValue();
// Fact: get derivation from database
Vector factList
= DbDerivation.GetDerivations(inStr, conn);
// update LexItems
for(int i = 0; i < factList.size(); i++)
{
DerivationRecord record = factList.elementAt(i);
String term = record.GetTarget();
long curCat = record.GetSourceCat();
// input category filter:
// inflection information is not in database, can't be check
if(InputFilter.IsLegal(inCat, curCat) == false)
{
continue;
}
// details & mutate
String details = null;
String mutate = null;
if(detailsFlag == true)
{
details = infoStr + " (FACT)";
}
if(mutateFlag == true)
{
String fs = GlobalBehavior.GetFieldSeparator();
mutate = "FACT" + fs
+ record.GetPureString(fs)
+ rFlowHistory + fs;
}
LexItem temp = UpdateLexItem(in, term, flowName,
record.GetTargetCat(),
Inflection.GetBitValue(Inflection.BASE_BIT),
details, mutate, false);
outs.addElement(temp);
}
// Rule: Use trie to get the result from rule
Vector ruleList =
trie.GetDerivationsByRules(inStr, inCat, inInfl, true);
// update LexItems
for(int i = 0; i < ruleList.size(); i++)
{
RuleResult record = ruleList.elementAt(i);
String term = record.GetOutTerm();
// details & mutate
String details = null;
String mutate = null;
if(detailsFlag == true)
{
details = infoStr + " (RULE|" + record.GetRuleString()
+ ")";
}
if(mutateFlag == true)
{
String fs = GlobalBehavior.GetFieldSeparator();
mutate = "RULE" + fs
+ record.GetInTerm() + fs
+ record.GetOutTerm() + fs
+ record.GetRuleString() + fs
+ rFlowHistory + fs;
}
LexItem temp = UpdateLexItem(in, term, flowName,
Category.ToValue(record.GetOutCategory()),
Inflection.ToValue(record.GetOutInflection()),
details, mutate, false);
outs.addElement(temp);
}
// Restrict the outputs
outs = ToDerivation.RestrictDerivations(outs, conn, restrictFlag);
}
catch (SQLException e)
{
System.err.println(
"** Error: Sql Exception in ToRecursiveDerivation Flow.");
System.err.println(e.toString());
}
// Sort: category, length, case incentive sort
LexItemComparator lc = new LexItemComparator();
lc.SetRule(LexItemComparator.LVG_RULE);
Collections.sort(outs, lc);
// mark tag for noun/adj and others
long adjValue = Category.GetBitValue(Category.ADJ_BIT);
long nounValue = Category.GetBitValue(Category.NOUN_BIT);
for(int i = 0; i < outs.size(); i++)
{
LexItem temp = outs.elementAt(i);
//check noun/adj
boolean curTagFlag =
((temp.GetSourceCategory().Contains(adjValue)
&& (temp.GetTargetCategory().Contains(nounValue)))
|| (temp.GetSourceCategory().Contains(nounValue)
&& (temp.GetTargetCategory().Contains(adjValue))));
Tag tag = new Tag(temp.GetTag());
boolean tagFlag =
curTagFlag && tag.GetBitFlag(Tag.DERV_NOUN_ADJ_BIT);
String tagStr = ((tagFlag)?"NounAdj":"NotNounAdj");
tag.SetBitFlag(Tag.DERV_NOUN_ADJ_BIT, tagFlag);
temp.SetTag(tag.GetValue());
String mutateInfo = temp.GetMutateInformation() + tagStr +
GlobalBehavior.GetFieldSeparator();
temp.SetMutateInformation(mutateInfo);
}
return outs;
}
private static void GetRecursiveDerivations(LexItem in, Connection conn,
RamTrie trie, int restrictFlag, String infoStr, boolean topLevel,
boolean detailsFlag, boolean mutateFlag, String rFlowHistory)
{
CalRecursiveDerivations(in, conn, trie, restrictFlag, infoStr,
topLevel, detailsFlag, mutateFlag, rFlowHistory);
// put result from hash table to a Vector
derivations_ = new Vector(derivationHt_.values());
}
private static void CalRecursiveDerivations(LexItem in, Connection conn,
RamTrie trie, int restrictFlag, String infoStr, boolean topLevel,
boolean detailsFlag, boolean mutateFlag, String rFlowHistory)
{
// calculate detail recursive history
StringBuffer buffer = new StringBuffer();
if(rFlowHistory == null)
{
String prevHistory = in.GetFlowHistory();
rFlowHistory = new String();
if(prevHistory == null)
{
prevHistory = new String();
}
else
{
buffer.append(prevHistory);
buffer.append("+");
}
}
buffer.append(Flow.GetBitName(Flow.DERIVATION, 1));
rFlowHistory += buffer.toString();
Vector temp = GetDerivations(in, conn, trie, restrictFlag,
infoStr, topLevel, detailsFlag, mutateFlag, rFlowHistory);
// Add into derivation List
for(int i = 0; i < temp.size(); i++)
{
LexItem tempRec = temp.elementAt(i);
TermCatCatKey tempKey = new TermCatCatKey(tempRec.GetTargetTerm(),
ToFruitfulVariants.GetFirstCategory(tempRec),
(int) tempRec.GetTargetCategory().GetValue());
if(derivationHt_.containsKey(tempKey) == true)
{
LexItem existRec = derivationHt_.get(tempKey);
String existFh = existRec.GetFlowHistory();
String tempFh = tempRec.GetFlowHistory();
// do the recursive when the new one with same key and less hist
if(existFh.length() > tempFh.length())
{
derivationHt_.remove(tempKey);
derivationHt_.put(tempKey, tempRec);
LexItem newLexItem = LexItem.TargetToSource(tempRec);
CalRecursiveDerivations(newLexItem, conn, trie,
restrictFlag, infoStr, false, detailsFlag, mutateFlag,
rFlowHistory);
}
}
else if(tempRec.GetTargetTerm().equals(orgInputTerm_) == false)
{
derivationHt_.put(tempKey, tempRec);
LexItem newLexItem = LexItem.TargetToSource(tempRec);
CalRecursiveDerivations(newLexItem, conn, trie, restrictFlag,
infoStr, false, detailsFlag, mutateFlag, rFlowHistory);
}
}
}
private static void Init(String inputTerm)
{
orgInputTerm_ = inputTerm;
derivationHt_.clear();
derivations_.removeAllElements();
}
// remove the very first elements since it is the original term
private static void UpdateFlowHistory(boolean detailFlowFlag)
{
// change the detail flow flag to "R"
if(detailFlowFlag == false)
{
String flowName = Flow.GetBitName(Flow.RECURSIVE_DERIVATIONS, 1);
for(int i = 0; i < derivations_.size(); i++)
{
LexItem cur = derivations_.elementAt(i);
cur.SetFlowHistory(flowName);
}
}
}
private static Vector GetDerivationVector()
{
return derivations_;
}
// data members
private static final String INFO = "Recursive Derivation";
private static Vector derivations_ = new Vector();
private static Hashtable derivationHt_
= new Hashtable();
private static String orgInputTerm_ = new String();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy