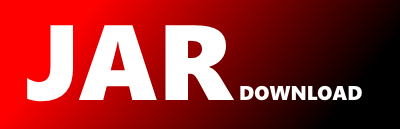
gov.nih.nlm.nls.lvg.Flows.ToStripNecNos Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lvg2010dist Show documentation
Show all versions of lvg2010dist Show documentation
LVG tools is used by Apache cTAKES.
The newest version!
package gov.nih.nlm.nls.lvg.Flows;
import java.util.*;
import java.io.*;
import gov.nih.nlm.nls.lvg.Util.*;
import gov.nih.nlm.nls.lvg.Lib.*;
/*****************************************************************************
* This class strips NEC and NOS from a specified term. NOS (Not Otherwise
* Specified) and NEC (Not Elsewhere Classified) can be found as part of
* Metathesaurus concept names. This class has following features:
*
* - strip NEC AND NOS
*
- Strip NEC/NOS$
*
- Strip NEC, NOS$
*
- Strip ,NEC$
*
- Strip ,NOS$
*
- Strip , NEC$
*
- Strip , NOS$
*
- Strip (NEC)
*
- Strip (NOS)
*
- Strip NEC
*
- Strip NOS
*
- Remove ',' if it is at the end of the stripped output.
*
* where $ is the end the string.
*
The design and implementation of this class is not general. More details
* specification need to be addressed, such as in the case of ", NEC" & ", NOS".
* In addition, the issue of tokenizing and puncuations need to be addessed.
*
*
History:
*
*
*
* @author NLM NLS Development Team
*
* @see
* Design Document
* @see
* Tokenize & Punctuations
*
* @version V-2010
****************************************************************************/
public class ToStripNecNos extends Transformation implements Cloneable
{
// public methods
/**
* Performs the mutation of this flow component.
*
* @param in a LexItem as the input for this flow component
* @param detailsFlag a boolean flag for processing details information
* @param mutateFlag a boolean flag for processing mutate information
*
* @return Vector - results from this flow component
*/
public static Vector Mutate(LexItem in, boolean detailsFlag,
boolean mutateFlag)
{
// mutate the term
String term = StripNecNos(in.GetSourceTerm());
// details & mutate
String details = null;
String mutate = null;
if(detailsFlag == true)
{
details = INFO;
}
if(mutateFlag == true)
{
mutate = Transformation.NO_MUTATE_INFO;
}
// updatea target
Vector out = new Vector();
LexItem temp = UpdateLexItem(in, term, Flow.STRIP_NEC_NOS,
Transformation.UPDATE, Transformation.UPDATE, details, mutate);
out.addElement(temp);
return out;
}
/**
* A unit test driver for this flow component.
*/
public static void main(String[] args)
{
String testStr = GetTestStr(args, "Deaf mutism, NEC");
// mutate
LexItem in = new LexItem(testStr);
Vector outs = ToStripNecNos.Mutate(in, true, true);
PrintResults(in, outs); // print out results
}
// private methods
private static String StripNecNos(String inStr)
{
// strip word if they are in the NEC NOS list
String tempStr = inStr + " $"; // add $ to the end of the term
String out = Strip.StripStrings(tempStr, necNosList_, true);
int length = out.length();
if(out.charAt(length-1) == '$')
{
out = out.substring(0, out.length()-1); // take out "$"
}
out = out.trim();
// take off ',' if it is at the end
length = out.length();
if(out.charAt(length-1) == ',')
{
out = out.substring(0, out.length()-1); // take out ","
}
return out.trim();
}
// data members
private static final String INFO = "Strip NEC and NOS";
private static Vector necNosList_ = new Vector();
// init the list of the format of NEC and NOS to be stripped
static
{
necNosList_.addElement("NEC AND NOS");
necNosList_.addElement("NEC/NOS $");
necNosList_.addElement("NEC NOS $");
necNosList_.addElement("NEC, NOS $");
necNosList_.addElement(", NEC $");
necNosList_.addElement(", NOS $");
necNosList_.addElement(",NEC $");
necNosList_.addElement(",NOS $");
necNosList_.addElement("(NEC)");
necNosList_.addElement("(NOS)");
necNosList_.addElement("NEC");
necNosList_.addElement("NOS");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy