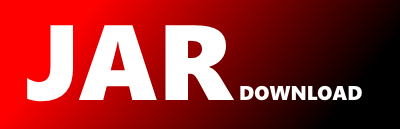
gov.nih.nlm.nls.lvg.Tools.GuiTool.GuiComp.InflectionPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lvg2010dist Show documentation
Show all versions of lvg2010dist Show documentation
LVG tools is used by Apache cTAKES.
The newest version!
package GuiTool.GuiComp;
import java.lang.*;
import java.util.*;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import javax.swing.event.*;
import gov.nih.nlm.nls.lvg.Lib.*;
import GuiTool.Global.*;
import GuiTool.GuiLib.*;
public class InflectionPanel extends JPanel
{
public InflectionPanel(ActionListener target, int col)
{
// Grid Layout
int row = (int) Math.ceil((double) LvgDef.INFLECTION_NUM/col);
setLayout(new GridLayout(row, col, 5, 3));
setBorder(BorderFactory.createTitledBorder(
BorderFactory.createEtchedBorder(), "Inflections"));
// init check box
for(int i = 0; i < LvgDef.INFLECTION_NUM; i++)
{
cb_[i] = new JCheckBox(LvgDef.INFLECTION[i] + " ("
+ LvgDef.INFLECTION_VALUE[i] + ")");
cb_[i].addActionListener(target);
add(cb_[i]);
}
}
public void UpdateCheckBox(long cat)
{
// update check box
for(int i = 0; i < LvgDef.INFLECTION_NUM; i++)
{
Vector catValues = Category.ToValues(cat);
boolean flag = false;
for(int j = 0; j < catValues.size(); j++)
{
long curCat = ((Long) catValues.elementAt(j)).longValue();
// disable check box if it is not a legal inflection
flag = flag ||
CatInfl.IsRelated(curCat, Inflection.GetBitValue(i));
}
cb_[i].setEnabled(flag);
}
}
public void ResetCheckBox()
{
for(int i = 0; i < LvgDef.INFLECTION_NUM; i++)
{
cb_[i].setEnabled(true);
}
}
public JCheckBox[] GetCheckBox()
{
return cb_;
}
// Test driver
public static void main(String[] args)
{
JFrame frame = new LibCloseableFrame("Inflection Panel");
frame.setContentPane(new InflectionPanel(new Handler(), 3)); // 3 col
frame.setSize(900, 250);
frame.setVisible(true);
}
// data members
private JCheckBox[] cb_ = new JCheckBox[LvgDef.INFLECTION_NUM];
private final static long serialVersionUID = 5L;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy