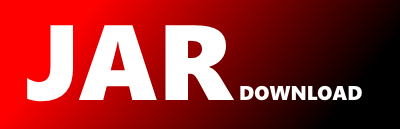
gov.nih.nlm.nls.lvg.Tools.ToAscii.toAscii Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lvg2010dist Show documentation
Show all versions of lvg2010dist Show documentation
LVG tools is used by Apache cTAKES.
The newest version!
import java.util.*;
import java.io.*;
import gov.nih.nlm.nls.lvg.CmdLineSyntax.*;
import gov.nih.nlm.nls.lvg.Lib.*;
import gov.nih.nlm.nls.lvg.Util.*;
import gov.nih.nlm.nls.lvg.Flows.*;
import gov.nih.nlm.nls.lvg.Api.*;
/*****************************************************************************
* This class is the command line program for ToAscii
*
* History:
*
* - SCR-6, chlu, 08-05-09, update Lexical Tool version to 2010
*
*
* @author NLM NLS Development Team
*
* @version V-2010
****************************************************************************/
public class toAscii extends SystemOption
{
// public method
// The flow chart for user-interface are:
// main() -> ToAscii.ExecuteCommands: to setup flags
// main() -> ToAscii.GetVariants() -> LineHandler()
public static void main(String[] args)
{
String optionStr = "";
Option io = new Option(optionStr);
// form optin String if there are arguments
if(args.length > 0)
{
optionStr = "";
for(int i = 0; i < args.length; i++)
{
if(i == 0)
{
optionStr = args[i];
}
else
{
optionStr += (" " + args[i]);
}
}
io = new Option(optionStr);
}
// define the system option flag & argument
toAscii toAscii = new toAscii();
// execute command according to option & argument
if(SystemOption.CheckSyntax(io, toAscii.GetOption(), false, true) == true)
{
// decode input option to form options
toAscii.ExecuteCommands(io, toAscii.GetOption());
// get config file from environment variable
boolean useClassPath = false;
if(configFile_ == null)
{
useClassPath = true;
configFile_ = "data.config.lvg";
}
// read in configuration file
if(conf_ == null)
{
conf_ = new Configuration(configFile_, useClassPath);
}
// init toASciiApi object
toAsciiApi_ = new ToAsciiApi(conf_);
if(conf_.GetConfiguration(Configuration.NO_OUTPUT) != null)
{
noOutputStr_ = conf_.GetConfiguration(Configuration.NO_OUTPUT);
}
if(Platform.IsWindow() == true)
{
promptStr_ =
"- Please input a term (type \"Ctl-z\" then \"Enter\" to quit) >";
}
else
{
promptStr_ = "- Please input a term (type \"Ctl-d\" to quit) >";
}
if(conf_.GetConfiguration(Configuration.LVG_PROMPT).equals(
"DEFAULT") == false)
{
promptStr_ = conf_.GetConfiguration(Configuration.LVG_PROMPT);
}
// read in input text and get the ToAscii for it
if(runFlag_ == true)
{
toAscii.GetVariants();
}
}
else
{
ToAsciiHelp.ToAsciiHelp(outWriter_, fileOutput_);
}
try
{
Close();
}
catch (Exception e)
{
e.printStackTrace();
}
}
// read data from user's line inputs
public static void GetVariants()
{
// decide the input source: from screen or file
if(inReader_ == null)
{
try
{
inReader_ = new BufferedReader(new InputStreamReader(
System.in, "UTF-8"));
}
catch (IOException e)
{
System.err.println("**Error: problem of reading std-in");
}
}
// perform ToAscii operation
try
{
while(true) // Loop forever - to go into prompt
{
if(promptFlag_ == true)
{
GetPrompt(); // Display a prompt to the user
}
// Read a line from the input source
if(LineHandler(inReader_.readLine()) == false)
{
break;
}
}
Close(); // close files & database
}
catch (Exception e)
{
e.printStackTrace();
}
}
// protected methods
protected void ExecuteCommand(OptionItem optionItem, Option systemOption)
{
OptionItem nameItem =
OptionUtility.GetItemByName(optionItem, systemOption, false);
Vector systemItems = systemOption.GetOptionItems();
if(CheckOption(nameItem, "-ci") == true)
{
try
{
// get config file from environment variable
boolean useClassPath = false;
String configFile = configFile_;
if(configFile == null)
{
useClassPath = true;
configFile = "data.config.lvg";
}
Configuration conf = conf_;
if(conf == null)
{
conf = new Configuration(configFile, useClassPath);
}
Out.Println(outWriter_, conf.GetInformation(), fileOutput_,
false);
}
catch (IOException e) { }
runFlag_ = false;
}
else if(CheckOption(nameItem, "-d") == true)
{
detailsFlag_ = true;
}
else if(CheckOption(nameItem, "-h") == true)
{
ToAsciiHelp.ToAsciiHelp(outWriter_, fileOutput_);
runFlag_ = false;
}
else if(CheckOption(nameItem, "-hs") == true)
{
systemOption.PrintOptionHierachy(); // not UTF-8
runFlag_ = false;
}
else if(CheckOption(nameItem, "-i:STR") == true)
{
String inFile = nameItem.GetOptionArgument();
if(inFile != null)
{
try
{
inReader_ = new BufferedReader(new InputStreamReader(
new FileInputStream(inFile), "UTF-8"));
}
catch (IOException e)
{
runFlag_ = false;
System.err.println(
"**Error: problem of opening/reading file " + inFile);
}
}
}
else if(CheckOption(nameItem, "-n") == true)
{
noOutputFlag_ = true;
}
else if(CheckOption(nameItem, "-o:STR") == true)
{
String outFile = nameItem.GetOptionArgument();
if(outFile != null)
{
try
{
outWriter_ = new BufferedWriter(new OutputStreamWriter(
new FileOutputStream(outFile), "UTF-8"));
fileOutput_ = true;
}
catch (IOException e)
{
runFlag_ = false;
System.err.println(
"**Error: problem of opening/writing file " + outFile);
}
}
}
else if(CheckOption(nameItem, "-p") == true)
{
promptFlag_ = true;
}
else if(CheckOption(nameItem, "-pio") == true)
{
preserveIoFlag_ = true;
}
else if(CheckOption(nameItem, "-v") == true)
{
try
{
Out.Println(outWriter_, "toAscii.2010", fileOutput_, false);
}
catch (IOException e) { }
runFlag_ = false;
}
else if(CheckOption(nameItem, "-x:STR") == true)
{
configFile_ = nameItem.GetOptionArgument();
}
}
protected void DefineFlag()
{
// define all option flags & arguments by giving a option string
String flagStr = "-ci -d -h -hs -i:STR -n -o:STR -p -pio -v -x:STR";
// init the system option
systemOption_ = new Option(flagStr);
// Add the full name for flags
systemOption_.SetFlagFullName("-ci", "Print_Config_Info");
systemOption_.SetFlagFullName("-d", "Print_Operation_Details");
systemOption_.SetFlagFullName("-h", "Help");
systemOption_.SetFlagFullName("-hs", "Hierarchy_Struture");
systemOption_.SetFlagFullName("-i", "Input_File");
systemOption_.SetFlagFullName("-n", "No_Output");
systemOption_.SetFlagFullName("-o", "Output_File");
systemOption_.SetFlagFullName("-p", "Show_Prompt");
systemOption_.SetFlagFullName("-pio", "Preserve_IO");
systemOption_.SetFlagFullName("-v", "Version");
systemOption_.SetFlagFullName("-x", "Load_Configuration_file");
}
// private methods
private static void Close() throws IOException
{
if(outWriter_ != null)
{
outWriter_.close();
}
if(inReader_ != null)
{
inReader_.close();
}
}
private static void GetPrompt() throws IOException
{
Out.Println(outWriter_, promptStr_, fileOutput_, false);
}
private static boolean LineHandler(String line)
throws IOException
{
// check input for quitiing
if(line == null)
{
return false;
}
// Get ToAscii result by going through flow -f:q7:q8
// get the term from appropriate field
LexItem in = new LexItem(line);
LexItem out = toAsciiApi_.Mutate(in, detailsFlag_);
// print results
// check no output flag
if((noOutputFlag_ == true) // no output flag
&& (out.GetTargetTerm().length() == 0)) // empty string
{
if(preserveIoFlag_ == true)
{
Out.Println(outWriter_, "Input: " + line, fileOutput_, false);
Out.Println(outWriter_, "Ascii: " + noOutputStr_, fileOutput_,
false);
}
else
{
Out.Println(outWriter_, noOutputStr_, fileOutput_, false);
}
}
else
{
if(preserveIoFlag_ == true)
{
Out.Println(outWriter_, "Input: " + line, fileOutput_, false);
Out.Println(outWriter_, "Ascii: " + out.GetTargetTerm(),
fileOutput_, false);
}
else
{
Out.Println(outWriter_, out.GetTargetTerm(), fileOutput_,
false);
}
// print details
if(detailsFlag_ == true)
{
Out.Println(outWriter_, out.GetDetailInformation(),
fileOutput_, false);
}
}
return true;
}
// data member
private static ToAsciiApi toAsciiApi_ = null;
private final static String NO_OUTPUT_STR = "-No Output-";
private static String noOutputStr_ = NO_OUTPUT_STR;
private static boolean detailsFlag_ = false; // flag for details print
private static boolean runFlag_ = true; // flag for running ToAscii
private static boolean noOutputFlag_ = false; // flag for return no output
private static boolean promptFlag_ = false; // flag for display prompt
private static boolean preserveIoFlag_ = false; // flag for preserveIo
private static BufferedReader inReader_ = null; // infile buffer
private static BufferedWriter outWriter_ = null; // outfile buffer
private static boolean fileOutput_ = false; // flag for file output
private static String configFile_ = null;
private static String promptStr_ = null;
private static Configuration conf_ = null;
static
{
try
{
outWriter_ = new BufferedWriter(new OutputStreamWriter(
System.out, "UTF-8"));
}
catch (IOException e)
{
System.err.println("**Error: problem of opening Std-out.");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy