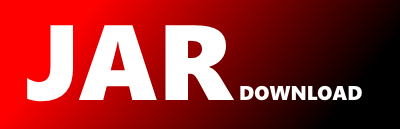
gov.nist.secauto.metaschema.model.common.metapath.antlr.metapath10Parser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of metaschema-model-common Show documentation
Show all versions of metaschema-model-common Show documentation
The core API for working with Metaschema-based models.
// Generated from metapath10.g4 by ANTLR 4.9.2
package gov.nist.secauto.metaschema.model.common.metapath.antlr;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
import java.util.List;
import java.util.Iterator;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast"})
public class metapath10Parser extends Parser {
static { RuntimeMetaData.checkVersion("4.9.2", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
AT=1, BANG=2, CB=3, CC=4, CEQ=5, COLON=6, COLONCOLON=7, COMMA=8, CP=9,
CS=10, D=11, DD=12, DOLLAR=13, EG=14, EQ=15, GE=16, GG=17, GT=18, LE=19,
LL=20, LT=21, MINUS=22, NE=23, OB=24, OC=25, OP=26, P=27, PLUS=28, POUND=29,
PP=30, QM=31, SC=32, SLASH=33, SS=34, STAR=35, KW_AND=36, KW_DIV=37, KW_EMPTY_SEQUENCE=38,
KW_EQ=39, KW_EXCEPT=40, KW_GE=41, KW_GT=42, KW_IDIV=43, KW_INTERSECT=44,
KW_LE=45, KW_LT=46, KW_MOD=47, KW_NE=48, KW_OR=49, KW_UNION=50, IntegerLiteral=51,
DecimalLiteral=52, DoubleLiteral=53, StringLiteral=54, Comment=55, LocalName=56,
NCName=57, Whitespace=58;
public static final int
RULE_metapath = 0, RULE_expr = 1, RULE_exprsingle = 2, RULE_orexpr = 3,
RULE_andexpr = 4, RULE_comparisonexpr = 5, RULE_stringconcatexpr = 6,
RULE_additiveexpr = 7, RULE_multiplicativeexpr = 8, RULE_unionexpr = 9,
RULE_intersectexceptexpr = 10, RULE_arrowexpr = 11, RULE_unaryexpr = 12,
RULE_valueexpr = 13, RULE_generalcomp = 14, RULE_valuecomp = 15, RULE_pathexpr = 16,
RULE_relativepathexpr = 17, RULE_stepexpr = 18, RULE_axisstep = 19, RULE_forwardstep = 20,
RULE_nametest = 21, RULE_wildcard = 22, RULE_postfixexpr = 23, RULE_argumentlist = 24,
RULE_predicatelist = 25, RULE_predicate = 26, RULE_arrowfunctionspecifier = 27,
RULE_primaryexpr = 28, RULE_literal = 29, RULE_numericliteral = 30, RULE_parenthesizedexpr = 31,
RULE_contextitemexpr = 32, RULE_functioncall = 33, RULE_argument = 34,
RULE_eqname = 35;
private static String[] makeRuleNames() {
return new String[] {
"metapath", "expr", "exprsingle", "orexpr", "andexpr", "comparisonexpr",
"stringconcatexpr", "additiveexpr", "multiplicativeexpr", "unionexpr",
"intersectexceptexpr", "arrowexpr", "unaryexpr", "valueexpr", "generalcomp",
"valuecomp", "pathexpr", "relativepathexpr", "stepexpr", "axisstep",
"forwardstep", "nametest", "wildcard", "postfixexpr", "argumentlist",
"predicatelist", "predicate", "arrowfunctionspecifier", "primaryexpr",
"literal", "numericliteral", "parenthesizedexpr", "contextitemexpr",
"functioncall", "argument", "eqname"
};
}
public static final String[] ruleNames = makeRuleNames();
private static String[] makeLiteralNames() {
return new String[] {
null, "'@'", "'!'", "']'", "'}'", "':='", "':'", "'::'", "','", "')'",
"':*'", "'.'", "'..'", "'$'", "'=>'", "'='", "'>='", "'>>'", "'>'", "'<='",
"'<<'", "'<'", "'-'", "'!='", "'['", "'{'", "'('", "'|'", "'+'", "'#'",
"'||'", "'?'", "'*:'", "'/'", "'//'", "'*'", "'and'", "'div'", "'empty-sequence'",
"'eq'", "'except'", "'ge'", "'gt'", "'idiv'", "'intersect'", "'le'",
"'lt'", "'mod'", "'ne'", "'or'", "'union'"
};
}
private static final String[] _LITERAL_NAMES = makeLiteralNames();
private static String[] makeSymbolicNames() {
return new String[] {
null, "AT", "BANG", "CB", "CC", "CEQ", "COLON", "COLONCOLON", "COMMA",
"CP", "CS", "D", "DD", "DOLLAR", "EG", "EQ", "GE", "GG", "GT", "LE",
"LL", "LT", "MINUS", "NE", "OB", "OC", "OP", "P", "PLUS", "POUND", "PP",
"QM", "SC", "SLASH", "SS", "STAR", "KW_AND", "KW_DIV", "KW_EMPTY_SEQUENCE",
"KW_EQ", "KW_EXCEPT", "KW_GE", "KW_GT", "KW_IDIV", "KW_INTERSECT", "KW_LE",
"KW_LT", "KW_MOD", "KW_NE", "KW_OR", "KW_UNION", "IntegerLiteral", "DecimalLiteral",
"DoubleLiteral", "StringLiteral", "Comment", "LocalName", "NCName", "Whitespace"
};
}
private static final String[] _SYMBOLIC_NAMES = makeSymbolicNames();
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() { return "metapath10.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public ATN getATN() { return _ATN; }
public metapath10Parser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
public static class MetapathContext extends ParserRuleContext {
public ExprContext expr() {
return getRuleContext(ExprContext.class,0);
}
public TerminalNode EOF() { return getToken(metapath10Parser.EOF, 0); }
public MetapathContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_metapath; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitMetapath(this);
else return visitor.visitChildren(this);
}
}
public final MetapathContext metapath() throws RecognitionException {
MetapathContext _localctx = new MetapathContext(_ctx, getState());
enterRule(_localctx, 0, RULE_metapath);
try {
enterOuterAlt(_localctx, 1);
{
setState(72);
expr();
setState(73);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ExprContext extends ParserRuleContext {
public List exprsingle() {
return getRuleContexts(ExprsingleContext.class);
}
public ExprsingleContext exprsingle(int i) {
return getRuleContext(ExprsingleContext.class,i);
}
public List COMMA() { return getTokens(metapath10Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(metapath10Parser.COMMA, i);
}
public ExprContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expr; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitExpr(this);
else return visitor.visitChildren(this);
}
}
public final ExprContext expr() throws RecognitionException {
ExprContext _localctx = new ExprContext(_ctx, getState());
enterRule(_localctx, 2, RULE_expr);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(75);
exprsingle();
setState(80);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(76);
match(COMMA);
setState(77);
exprsingle();
}
}
setState(82);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ExprsingleContext extends ParserRuleContext {
public OrexprContext orexpr() {
return getRuleContext(OrexprContext.class,0);
}
public ExprsingleContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_exprsingle; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitExprsingle(this);
else return visitor.visitChildren(this);
}
}
public final ExprsingleContext exprsingle() throws RecognitionException {
ExprsingleContext _localctx = new ExprsingleContext(_ctx, getState());
enterRule(_localctx, 4, RULE_exprsingle);
try {
enterOuterAlt(_localctx, 1);
{
setState(83);
orexpr();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class OrexprContext extends ParserRuleContext {
public List andexpr() {
return getRuleContexts(AndexprContext.class);
}
public AndexprContext andexpr(int i) {
return getRuleContext(AndexprContext.class,i);
}
public List KW_OR() { return getTokens(metapath10Parser.KW_OR); }
public TerminalNode KW_OR(int i) {
return getToken(metapath10Parser.KW_OR, i);
}
public OrexprContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_orexpr; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitOrexpr(this);
else return visitor.visitChildren(this);
}
}
public final OrexprContext orexpr() throws RecognitionException {
OrexprContext _localctx = new OrexprContext(_ctx, getState());
enterRule(_localctx, 6, RULE_orexpr);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(85);
andexpr();
setState(90);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==KW_OR) {
{
{
setState(86);
match(KW_OR);
setState(87);
andexpr();
}
}
setState(92);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AndexprContext extends ParserRuleContext {
public List comparisonexpr() {
return getRuleContexts(ComparisonexprContext.class);
}
public ComparisonexprContext comparisonexpr(int i) {
return getRuleContext(ComparisonexprContext.class,i);
}
public List KW_AND() { return getTokens(metapath10Parser.KW_AND); }
public TerminalNode KW_AND(int i) {
return getToken(metapath10Parser.KW_AND, i);
}
public AndexprContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_andexpr; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitAndexpr(this);
else return visitor.visitChildren(this);
}
}
public final AndexprContext andexpr() throws RecognitionException {
AndexprContext _localctx = new AndexprContext(_ctx, getState());
enterRule(_localctx, 8, RULE_andexpr);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(93);
comparisonexpr();
setState(98);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==KW_AND) {
{
{
setState(94);
match(KW_AND);
setState(95);
comparisonexpr();
}
}
setState(100);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ComparisonexprContext extends ParserRuleContext {
public List stringconcatexpr() {
return getRuleContexts(StringconcatexprContext.class);
}
public StringconcatexprContext stringconcatexpr(int i) {
return getRuleContext(StringconcatexprContext.class,i);
}
public ValuecompContext valuecomp() {
return getRuleContext(ValuecompContext.class,0);
}
public GeneralcompContext generalcomp() {
return getRuleContext(GeneralcompContext.class,0);
}
public ComparisonexprContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_comparisonexpr; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitComparisonexpr(this);
else return visitor.visitChildren(this);
}
}
public final ComparisonexprContext comparisonexpr() throws RecognitionException {
ComparisonexprContext _localctx = new ComparisonexprContext(_ctx, getState());
enterRule(_localctx, 10, RULE_comparisonexpr);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(101);
stringconcatexpr();
setState(108);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << EQ) | (1L << GE) | (1L << GT) | (1L << LE) | (1L << LT) | (1L << NE) | (1L << KW_EQ) | (1L << KW_GE) | (1L << KW_GT) | (1L << KW_LE) | (1L << KW_LT) | (1L << KW_NE))) != 0)) {
{
setState(104);
_errHandler.sync(this);
switch (_input.LA(1)) {
case KW_EQ:
case KW_GE:
case KW_GT:
case KW_LE:
case KW_LT:
case KW_NE:
{
setState(102);
valuecomp();
}
break;
case EQ:
case GE:
case GT:
case LE:
case LT:
case NE:
{
setState(103);
generalcomp();
}
break;
default:
throw new NoViableAltException(this);
}
setState(106);
stringconcatexpr();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class StringconcatexprContext extends ParserRuleContext {
public List additiveexpr() {
return getRuleContexts(AdditiveexprContext.class);
}
public AdditiveexprContext additiveexpr(int i) {
return getRuleContext(AdditiveexprContext.class,i);
}
public List PP() { return getTokens(metapath10Parser.PP); }
public TerminalNode PP(int i) {
return getToken(metapath10Parser.PP, i);
}
public StringconcatexprContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_stringconcatexpr; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitStringconcatexpr(this);
else return visitor.visitChildren(this);
}
}
public final StringconcatexprContext stringconcatexpr() throws RecognitionException {
StringconcatexprContext _localctx = new StringconcatexprContext(_ctx, getState());
enterRule(_localctx, 12, RULE_stringconcatexpr);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(110);
additiveexpr();
setState(115);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==PP) {
{
{
setState(111);
match(PP);
setState(112);
additiveexpr();
}
}
setState(117);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AdditiveexprContext extends ParserRuleContext {
public List multiplicativeexpr() {
return getRuleContexts(MultiplicativeexprContext.class);
}
public MultiplicativeexprContext multiplicativeexpr(int i) {
return getRuleContext(MultiplicativeexprContext.class,i);
}
public List PLUS() { return getTokens(metapath10Parser.PLUS); }
public TerminalNode PLUS(int i) {
return getToken(metapath10Parser.PLUS, i);
}
public List MINUS() { return getTokens(metapath10Parser.MINUS); }
public TerminalNode MINUS(int i) {
return getToken(metapath10Parser.MINUS, i);
}
public AdditiveexprContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_additiveexpr; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitAdditiveexpr(this);
else return visitor.visitChildren(this);
}
}
public final AdditiveexprContext additiveexpr() throws RecognitionException {
AdditiveexprContext _localctx = new AdditiveexprContext(_ctx, getState());
enterRule(_localctx, 14, RULE_additiveexpr);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(118);
multiplicativeexpr();
setState(123);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==MINUS || _la==PLUS) {
{
{
setState(119);
_la = _input.LA(1);
if ( !(_la==MINUS || _la==PLUS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(120);
multiplicativeexpr();
}
}
setState(125);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class MultiplicativeexprContext extends ParserRuleContext {
public List unionexpr() {
return getRuleContexts(UnionexprContext.class);
}
public UnionexprContext unionexpr(int i) {
return getRuleContext(UnionexprContext.class,i);
}
public List STAR() { return getTokens(metapath10Parser.STAR); }
public TerminalNode STAR(int i) {
return getToken(metapath10Parser.STAR, i);
}
public List KW_DIV() { return getTokens(metapath10Parser.KW_DIV); }
public TerminalNode KW_DIV(int i) {
return getToken(metapath10Parser.KW_DIV, i);
}
public List KW_IDIV() { return getTokens(metapath10Parser.KW_IDIV); }
public TerminalNode KW_IDIV(int i) {
return getToken(metapath10Parser.KW_IDIV, i);
}
public List KW_MOD() { return getTokens(metapath10Parser.KW_MOD); }
public TerminalNode KW_MOD(int i) {
return getToken(metapath10Parser.KW_MOD, i);
}
public MultiplicativeexprContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_multiplicativeexpr; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitMultiplicativeexpr(this);
else return visitor.visitChildren(this);
}
}
public final MultiplicativeexprContext multiplicativeexpr() throws RecognitionException {
MultiplicativeexprContext _localctx = new MultiplicativeexprContext(_ctx, getState());
enterRule(_localctx, 16, RULE_multiplicativeexpr);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(126);
unionexpr();
setState(131);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << STAR) | (1L << KW_DIV) | (1L << KW_IDIV) | (1L << KW_MOD))) != 0)) {
{
{
setState(127);
_la = _input.LA(1);
if ( !((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << STAR) | (1L << KW_DIV) | (1L << KW_IDIV) | (1L << KW_MOD))) != 0)) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(128);
unionexpr();
}
}
setState(133);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class UnionexprContext extends ParserRuleContext {
public List intersectexceptexpr() {
return getRuleContexts(IntersectexceptexprContext.class);
}
public IntersectexceptexprContext intersectexceptexpr(int i) {
return getRuleContext(IntersectexceptexprContext.class,i);
}
public List KW_UNION() { return getTokens(metapath10Parser.KW_UNION); }
public TerminalNode KW_UNION(int i) {
return getToken(metapath10Parser.KW_UNION, i);
}
public List P() { return getTokens(metapath10Parser.P); }
public TerminalNode P(int i) {
return getToken(metapath10Parser.P, i);
}
public UnionexprContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_unionexpr; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitUnionexpr(this);
else return visitor.visitChildren(this);
}
}
public final UnionexprContext unionexpr() throws RecognitionException {
UnionexprContext _localctx = new UnionexprContext(_ctx, getState());
enterRule(_localctx, 18, RULE_unionexpr);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(134);
intersectexceptexpr();
setState(139);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==P || _la==KW_UNION) {
{
{
setState(135);
_la = _input.LA(1);
if ( !(_la==P || _la==KW_UNION) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(136);
intersectexceptexpr();
}
}
setState(141);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IntersectexceptexprContext extends ParserRuleContext {
public List arrowexpr() {
return getRuleContexts(ArrowexprContext.class);
}
public ArrowexprContext arrowexpr(int i) {
return getRuleContext(ArrowexprContext.class,i);
}
public List KW_INTERSECT() { return getTokens(metapath10Parser.KW_INTERSECT); }
public TerminalNode KW_INTERSECT(int i) {
return getToken(metapath10Parser.KW_INTERSECT, i);
}
public List KW_EXCEPT() { return getTokens(metapath10Parser.KW_EXCEPT); }
public TerminalNode KW_EXCEPT(int i) {
return getToken(metapath10Parser.KW_EXCEPT, i);
}
public IntersectexceptexprContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_intersectexceptexpr; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitIntersectexceptexpr(this);
else return visitor.visitChildren(this);
}
}
public final IntersectexceptexprContext intersectexceptexpr() throws RecognitionException {
IntersectexceptexprContext _localctx = new IntersectexceptexprContext(_ctx, getState());
enterRule(_localctx, 20, RULE_intersectexceptexpr);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(142);
arrowexpr();
setState(147);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==KW_EXCEPT || _la==KW_INTERSECT) {
{
{
setState(143);
_la = _input.LA(1);
if ( !(_la==KW_EXCEPT || _la==KW_INTERSECT) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(144);
arrowexpr();
}
}
setState(149);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ArrowexprContext extends ParserRuleContext {
public UnaryexprContext unaryexpr() {
return getRuleContext(UnaryexprContext.class,0);
}
public List EG() { return getTokens(metapath10Parser.EG); }
public TerminalNode EG(int i) {
return getToken(metapath10Parser.EG, i);
}
public List arrowfunctionspecifier() {
return getRuleContexts(ArrowfunctionspecifierContext.class);
}
public ArrowfunctionspecifierContext arrowfunctionspecifier(int i) {
return getRuleContext(ArrowfunctionspecifierContext.class,i);
}
public List argumentlist() {
return getRuleContexts(ArgumentlistContext.class);
}
public ArgumentlistContext argumentlist(int i) {
return getRuleContext(ArgumentlistContext.class,i);
}
public ArrowexprContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_arrowexpr; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitArrowexpr(this);
else return visitor.visitChildren(this);
}
}
public final ArrowexprContext arrowexpr() throws RecognitionException {
ArrowexprContext _localctx = new ArrowexprContext(_ctx, getState());
enterRule(_localctx, 22, RULE_arrowexpr);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(150);
unaryexpr();
setState(157);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==EG) {
{
{
setState(151);
match(EG);
setState(152);
arrowfunctionspecifier();
setState(153);
argumentlist();
}
}
setState(159);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class UnaryexprContext extends ParserRuleContext {
public ValueexprContext valueexpr() {
return getRuleContext(ValueexprContext.class,0);
}
public List MINUS() { return getTokens(metapath10Parser.MINUS); }
public TerminalNode MINUS(int i) {
return getToken(metapath10Parser.MINUS, i);
}
public List PLUS() { return getTokens(metapath10Parser.PLUS); }
public TerminalNode PLUS(int i) {
return getToken(metapath10Parser.PLUS, i);
}
public UnaryexprContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_unaryexpr; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitUnaryexpr(this);
else return visitor.visitChildren(this);
}
}
public final UnaryexprContext unaryexpr() throws RecognitionException {
UnaryexprContext _localctx = new UnaryexprContext(_ctx, getState());
enterRule(_localctx, 24, RULE_unaryexpr);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(163);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,11,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(160);
_la = _input.LA(1);
if ( !(_la==MINUS || _la==PLUS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
}
setState(165);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,11,_ctx);
}
setState(166);
valueexpr();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ValueexprContext extends ParserRuleContext {
public PathexprContext pathexpr() {
return getRuleContext(PathexprContext.class,0);
}
public ValueexprContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_valueexpr; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitValueexpr(this);
else return visitor.visitChildren(this);
}
}
public final ValueexprContext valueexpr() throws RecognitionException {
ValueexprContext _localctx = new ValueexprContext(_ctx, getState());
enterRule(_localctx, 26, RULE_valueexpr);
try {
enterOuterAlt(_localctx, 1);
{
setState(168);
pathexpr();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class GeneralcompContext extends ParserRuleContext {
public TerminalNode EQ() { return getToken(metapath10Parser.EQ, 0); }
public TerminalNode NE() { return getToken(metapath10Parser.NE, 0); }
public TerminalNode LT() { return getToken(metapath10Parser.LT, 0); }
public TerminalNode LE() { return getToken(metapath10Parser.LE, 0); }
public TerminalNode GT() { return getToken(metapath10Parser.GT, 0); }
public TerminalNode GE() { return getToken(metapath10Parser.GE, 0); }
public GeneralcompContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_generalcomp; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitGeneralcomp(this);
else return visitor.visitChildren(this);
}
}
public final GeneralcompContext generalcomp() throws RecognitionException {
GeneralcompContext _localctx = new GeneralcompContext(_ctx, getState());
enterRule(_localctx, 28, RULE_generalcomp);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(170);
_la = _input.LA(1);
if ( !((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << EQ) | (1L << GE) | (1L << GT) | (1L << LE) | (1L << LT) | (1L << NE))) != 0)) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ValuecompContext extends ParserRuleContext {
public TerminalNode KW_EQ() { return getToken(metapath10Parser.KW_EQ, 0); }
public TerminalNode KW_NE() { return getToken(metapath10Parser.KW_NE, 0); }
public TerminalNode KW_LT() { return getToken(metapath10Parser.KW_LT, 0); }
public TerminalNode KW_LE() { return getToken(metapath10Parser.KW_LE, 0); }
public TerminalNode KW_GT() { return getToken(metapath10Parser.KW_GT, 0); }
public TerminalNode KW_GE() { return getToken(metapath10Parser.KW_GE, 0); }
public ValuecompContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_valuecomp; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitValuecomp(this);
else return visitor.visitChildren(this);
}
}
public final ValuecompContext valuecomp() throws RecognitionException {
ValuecompContext _localctx = new ValuecompContext(_ctx, getState());
enterRule(_localctx, 30, RULE_valuecomp);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(172);
_la = _input.LA(1);
if ( !((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << KW_EQ) | (1L << KW_GE) | (1L << KW_GT) | (1L << KW_LE) | (1L << KW_LT) | (1L << KW_NE))) != 0)) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PathexprContext extends ParserRuleContext {
public TerminalNode SLASH() { return getToken(metapath10Parser.SLASH, 0); }
public RelativepathexprContext relativepathexpr() {
return getRuleContext(RelativepathexprContext.class,0);
}
public TerminalNode SS() { return getToken(metapath10Parser.SS, 0); }
public PathexprContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_pathexpr; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitPathexpr(this);
else return visitor.visitChildren(this);
}
}
public final PathexprContext pathexpr() throws RecognitionException {
PathexprContext _localctx = new PathexprContext(_ctx, getState());
enterRule(_localctx, 32, RULE_pathexpr);
try {
setState(181);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,13,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
{
setState(174);
match(SLASH);
setState(176);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,12,_ctx) ) {
case 1:
{
setState(175);
relativepathexpr();
}
break;
}
}
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
{
setState(178);
match(SS);
setState(179);
relativepathexpr();
}
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(180);
relativepathexpr();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class RelativepathexprContext extends ParserRuleContext {
public List stepexpr() {
return getRuleContexts(StepexprContext.class);
}
public StepexprContext stepexpr(int i) {
return getRuleContext(StepexprContext.class,i);
}
public List SLASH() { return getTokens(metapath10Parser.SLASH); }
public TerminalNode SLASH(int i) {
return getToken(metapath10Parser.SLASH, i);
}
public List SS() { return getTokens(metapath10Parser.SS); }
public TerminalNode SS(int i) {
return getToken(metapath10Parser.SS, i);
}
public RelativepathexprContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_relativepathexpr; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitRelativepathexpr(this);
else return visitor.visitChildren(this);
}
}
public final RelativepathexprContext relativepathexpr() throws RecognitionException {
RelativepathexprContext _localctx = new RelativepathexprContext(_ctx, getState());
enterRule(_localctx, 34, RULE_relativepathexpr);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(183);
stepexpr();
setState(188);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==SLASH || _la==SS) {
{
{
setState(184);
_la = _input.LA(1);
if ( !(_la==SLASH || _la==SS) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(185);
stepexpr();
}
}
setState(190);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class StepexprContext extends ParserRuleContext {
public PostfixexprContext postfixexpr() {
return getRuleContext(PostfixexprContext.class,0);
}
public AxisstepContext axisstep() {
return getRuleContext(AxisstepContext.class,0);
}
public StepexprContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_stepexpr; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitStepexpr(this);
else return visitor.visitChildren(this);
}
}
public final StepexprContext stepexpr() throws RecognitionException {
StepexprContext _localctx = new StepexprContext(_ctx, getState());
enterRule(_localctx, 36, RULE_stepexpr);
try {
setState(193);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,15,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(191);
postfixexpr();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(192);
axisstep();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AxisstepContext extends ParserRuleContext {
public ForwardstepContext forwardstep() {
return getRuleContext(ForwardstepContext.class,0);
}
public PredicatelistContext predicatelist() {
return getRuleContext(PredicatelistContext.class,0);
}
public AxisstepContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_axisstep; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitAxisstep(this);
else return visitor.visitChildren(this);
}
}
public final AxisstepContext axisstep() throws RecognitionException {
AxisstepContext _localctx = new AxisstepContext(_ctx, getState());
enterRule(_localctx, 38, RULE_axisstep);
try {
enterOuterAlt(_localctx, 1);
{
setState(195);
forwardstep();
setState(196);
predicatelist();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ForwardstepContext extends ParserRuleContext {
public NametestContext nametest() {
return getRuleContext(NametestContext.class,0);
}
public TerminalNode AT() { return getToken(metapath10Parser.AT, 0); }
public ForwardstepContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_forwardstep; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitForwardstep(this);
else return visitor.visitChildren(this);
}
}
public final ForwardstepContext forwardstep() throws RecognitionException {
ForwardstepContext _localctx = new ForwardstepContext(_ctx, getState());
enterRule(_localctx, 40, RULE_forwardstep);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(199);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==AT) {
{
setState(198);
match(AT);
}
}
setState(201);
nametest();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class NametestContext extends ParserRuleContext {
public EqnameContext eqname() {
return getRuleContext(EqnameContext.class,0);
}
public WildcardContext wildcard() {
return getRuleContext(WildcardContext.class,0);
}
public NametestContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_nametest; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitNametest(this);
else return visitor.visitChildren(this);
}
}
public final NametestContext nametest() throws RecognitionException {
NametestContext _localctx = new NametestContext(_ctx, getState());
enterRule(_localctx, 42, RULE_nametest);
try {
setState(205);
_errHandler.sync(this);
switch (_input.LA(1)) {
case KW_AND:
case KW_DIV:
case KW_EMPTY_SEQUENCE:
case KW_EQ:
case KW_EXCEPT:
case KW_GE:
case KW_GT:
case KW_IDIV:
case KW_INTERSECT:
case KW_LE:
case KW_LT:
case KW_MOD:
case KW_NE:
case KW_OR:
case KW_UNION:
case LocalName:
enterOuterAlt(_localctx, 1);
{
setState(203);
eqname();
}
break;
case STAR:
enterOuterAlt(_localctx, 2);
{
setState(204);
wildcard();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class WildcardContext extends ParserRuleContext {
public TerminalNode STAR() { return getToken(metapath10Parser.STAR, 0); }
public WildcardContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_wildcard; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitWildcard(this);
else return visitor.visitChildren(this);
}
}
public final WildcardContext wildcard() throws RecognitionException {
WildcardContext _localctx = new WildcardContext(_ctx, getState());
enterRule(_localctx, 44, RULE_wildcard);
try {
enterOuterAlt(_localctx, 1);
{
setState(207);
match(STAR);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PostfixexprContext extends ParserRuleContext {
public PrimaryexprContext primaryexpr() {
return getRuleContext(PrimaryexprContext.class,0);
}
public List predicate() {
return getRuleContexts(PredicateContext.class);
}
public PredicateContext predicate(int i) {
return getRuleContext(PredicateContext.class,i);
}
public PostfixexprContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_postfixexpr; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitPostfixexpr(this);
else return visitor.visitChildren(this);
}
}
public final PostfixexprContext postfixexpr() throws RecognitionException {
PostfixexprContext _localctx = new PostfixexprContext(_ctx, getState());
enterRule(_localctx, 46, RULE_postfixexpr);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(209);
primaryexpr();
setState(213);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==OB) {
{
{
setState(210);
predicate();
}
}
setState(215);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ArgumentlistContext extends ParserRuleContext {
public TerminalNode OP() { return getToken(metapath10Parser.OP, 0); }
public TerminalNode CP() { return getToken(metapath10Parser.CP, 0); }
public List argument() {
return getRuleContexts(ArgumentContext.class);
}
public ArgumentContext argument(int i) {
return getRuleContext(ArgumentContext.class,i);
}
public List COMMA() { return getTokens(metapath10Parser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(metapath10Parser.COMMA, i);
}
public ArgumentlistContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_argumentlist; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitArgumentlist(this);
else return visitor.visitChildren(this);
}
}
public final ArgumentlistContext argumentlist() throws RecognitionException {
ArgumentlistContext _localctx = new ArgumentlistContext(_ctx, getState());
enterRule(_localctx, 48, RULE_argumentlist);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(216);
match(OP);
setState(225);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,20,_ctx) ) {
case 1:
{
setState(217);
argument();
setState(222);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(218);
match(COMMA);
setState(219);
argument();
}
}
setState(224);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
break;
}
setState(227);
match(CP);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PredicatelistContext extends ParserRuleContext {
public List predicate() {
return getRuleContexts(PredicateContext.class);
}
public PredicateContext predicate(int i) {
return getRuleContext(PredicateContext.class,i);
}
public PredicatelistContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_predicatelist; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitPredicatelist(this);
else return visitor.visitChildren(this);
}
}
public final PredicatelistContext predicatelist() throws RecognitionException {
PredicatelistContext _localctx = new PredicatelistContext(_ctx, getState());
enterRule(_localctx, 50, RULE_predicatelist);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(232);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==OB) {
{
{
setState(229);
predicate();
}
}
setState(234);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PredicateContext extends ParserRuleContext {
public TerminalNode OB() { return getToken(metapath10Parser.OB, 0); }
public ExprContext expr() {
return getRuleContext(ExprContext.class,0);
}
public TerminalNode CB() { return getToken(metapath10Parser.CB, 0); }
public PredicateContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_predicate; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitPredicate(this);
else return visitor.visitChildren(this);
}
}
public final PredicateContext predicate() throws RecognitionException {
PredicateContext _localctx = new PredicateContext(_ctx, getState());
enterRule(_localctx, 52, RULE_predicate);
try {
enterOuterAlt(_localctx, 1);
{
setState(235);
match(OB);
setState(236);
expr();
setState(237);
match(CB);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ArrowfunctionspecifierContext extends ParserRuleContext {
public EqnameContext eqname() {
return getRuleContext(EqnameContext.class,0);
}
public ParenthesizedexprContext parenthesizedexpr() {
return getRuleContext(ParenthesizedexprContext.class,0);
}
public ArrowfunctionspecifierContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_arrowfunctionspecifier; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitArrowfunctionspecifier(this);
else return visitor.visitChildren(this);
}
}
public final ArrowfunctionspecifierContext arrowfunctionspecifier() throws RecognitionException {
ArrowfunctionspecifierContext _localctx = new ArrowfunctionspecifierContext(_ctx, getState());
enterRule(_localctx, 54, RULE_arrowfunctionspecifier);
try {
setState(241);
_errHandler.sync(this);
switch (_input.LA(1)) {
case KW_AND:
case KW_DIV:
case KW_EMPTY_SEQUENCE:
case KW_EQ:
case KW_EXCEPT:
case KW_GE:
case KW_GT:
case KW_IDIV:
case KW_INTERSECT:
case KW_LE:
case KW_LT:
case KW_MOD:
case KW_NE:
case KW_OR:
case KW_UNION:
case LocalName:
enterOuterAlt(_localctx, 1);
{
setState(239);
eqname();
}
break;
case OP:
enterOuterAlt(_localctx, 2);
{
setState(240);
parenthesizedexpr();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PrimaryexprContext extends ParserRuleContext {
public LiteralContext literal() {
return getRuleContext(LiteralContext.class,0);
}
public ParenthesizedexprContext parenthesizedexpr() {
return getRuleContext(ParenthesizedexprContext.class,0);
}
public ContextitemexprContext contextitemexpr() {
return getRuleContext(ContextitemexprContext.class,0);
}
public FunctioncallContext functioncall() {
return getRuleContext(FunctioncallContext.class,0);
}
public PrimaryexprContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_primaryexpr; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitPrimaryexpr(this);
else return visitor.visitChildren(this);
}
}
public final PrimaryexprContext primaryexpr() throws RecognitionException {
PrimaryexprContext _localctx = new PrimaryexprContext(_ctx, getState());
enterRule(_localctx, 56, RULE_primaryexpr);
try {
setState(247);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,23,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(243);
literal();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(244);
parenthesizedexpr();
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(245);
contextitemexpr();
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(246);
functioncall();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class LiteralContext extends ParserRuleContext {
public NumericliteralContext numericliteral() {
return getRuleContext(NumericliteralContext.class,0);
}
public TerminalNode StringLiteral() { return getToken(metapath10Parser.StringLiteral, 0); }
public LiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_literal; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitLiteral(this);
else return visitor.visitChildren(this);
}
}
public final LiteralContext literal() throws RecognitionException {
LiteralContext _localctx = new LiteralContext(_ctx, getState());
enterRule(_localctx, 58, RULE_literal);
try {
setState(251);
_errHandler.sync(this);
switch (_input.LA(1)) {
case IntegerLiteral:
case DecimalLiteral:
case DoubleLiteral:
enterOuterAlt(_localctx, 1);
{
setState(249);
numericliteral();
}
break;
case StringLiteral:
enterOuterAlt(_localctx, 2);
{
setState(250);
match(StringLiteral);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class NumericliteralContext extends ParserRuleContext {
public TerminalNode IntegerLiteral() { return getToken(metapath10Parser.IntegerLiteral, 0); }
public TerminalNode DecimalLiteral() { return getToken(metapath10Parser.DecimalLiteral, 0); }
public TerminalNode DoubleLiteral() { return getToken(metapath10Parser.DoubleLiteral, 0); }
public NumericliteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_numericliteral; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitNumericliteral(this);
else return visitor.visitChildren(this);
}
}
public final NumericliteralContext numericliteral() throws RecognitionException {
NumericliteralContext _localctx = new NumericliteralContext(_ctx, getState());
enterRule(_localctx, 60, RULE_numericliteral);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(253);
_la = _input.LA(1);
if ( !((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << IntegerLiteral) | (1L << DecimalLiteral) | (1L << DoubleLiteral))) != 0)) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ParenthesizedexprContext extends ParserRuleContext {
public TerminalNode OP() { return getToken(metapath10Parser.OP, 0); }
public TerminalNode CP() { return getToken(metapath10Parser.CP, 0); }
public ExprContext expr() {
return getRuleContext(ExprContext.class,0);
}
public ParenthesizedexprContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_parenthesizedexpr; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitParenthesizedexpr(this);
else return visitor.visitChildren(this);
}
}
public final ParenthesizedexprContext parenthesizedexpr() throws RecognitionException {
ParenthesizedexprContext _localctx = new ParenthesizedexprContext(_ctx, getState());
enterRule(_localctx, 62, RULE_parenthesizedexpr);
try {
enterOuterAlt(_localctx, 1);
{
setState(255);
match(OP);
setState(257);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,25,_ctx) ) {
case 1:
{
setState(256);
expr();
}
break;
}
setState(259);
match(CP);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ContextitemexprContext extends ParserRuleContext {
public TerminalNode D() { return getToken(metapath10Parser.D, 0); }
public ContextitemexprContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_contextitemexpr; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitContextitemexpr(this);
else return visitor.visitChildren(this);
}
}
public final ContextitemexprContext contextitemexpr() throws RecognitionException {
ContextitemexprContext _localctx = new ContextitemexprContext(_ctx, getState());
enterRule(_localctx, 64, RULE_contextitemexpr);
try {
enterOuterAlt(_localctx, 1);
{
setState(261);
match(D);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class FunctioncallContext extends ParserRuleContext {
public EqnameContext eqname() {
return getRuleContext(EqnameContext.class,0);
}
public ArgumentlistContext argumentlist() {
return getRuleContext(ArgumentlistContext.class,0);
}
public FunctioncallContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_functioncall; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitFunctioncall(this);
else return visitor.visitChildren(this);
}
}
public final FunctioncallContext functioncall() throws RecognitionException {
FunctioncallContext _localctx = new FunctioncallContext(_ctx, getState());
enterRule(_localctx, 66, RULE_functioncall);
try {
enterOuterAlt(_localctx, 1);
{
setState(263);
if (!( !(
getInputStream().LA(1)==KW_EMPTY_SEQUENCE
) )) throw new FailedPredicateException(this, " !(\n getInputStream().LA(1)==KW_EMPTY_SEQUENCE\n ) ");
setState(264);
eqname();
setState(265);
argumentlist();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ArgumentContext extends ParserRuleContext {
public ExprsingleContext exprsingle() {
return getRuleContext(ExprsingleContext.class,0);
}
public ArgumentContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_argument; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitArgument(this);
else return visitor.visitChildren(this);
}
}
public final ArgumentContext argument() throws RecognitionException {
ArgumentContext _localctx = new ArgumentContext(_ctx, getState());
enterRule(_localctx, 68, RULE_argument);
try {
enterOuterAlt(_localctx, 1);
{
setState(267);
exprsingle();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class EqnameContext extends ParserRuleContext {
public TerminalNode LocalName() { return getToken(metapath10Parser.LocalName, 0); }
public TerminalNode KW_AND() { return getToken(metapath10Parser.KW_AND, 0); }
public TerminalNode KW_DIV() { return getToken(metapath10Parser.KW_DIV, 0); }
public TerminalNode KW_EMPTY_SEQUENCE() { return getToken(metapath10Parser.KW_EMPTY_SEQUENCE, 0); }
public TerminalNode KW_EQ() { return getToken(metapath10Parser.KW_EQ, 0); }
public TerminalNode KW_EXCEPT() { return getToken(metapath10Parser.KW_EXCEPT, 0); }
public TerminalNode KW_GE() { return getToken(metapath10Parser.KW_GE, 0); }
public TerminalNode KW_GT() { return getToken(metapath10Parser.KW_GT, 0); }
public TerminalNode KW_IDIV() { return getToken(metapath10Parser.KW_IDIV, 0); }
public TerminalNode KW_INTERSECT() { return getToken(metapath10Parser.KW_INTERSECT, 0); }
public TerminalNode KW_LE() { return getToken(metapath10Parser.KW_LE, 0); }
public TerminalNode KW_LT() { return getToken(metapath10Parser.KW_LT, 0); }
public TerminalNode KW_MOD() { return getToken(metapath10Parser.KW_MOD, 0); }
public TerminalNode KW_NE() { return getToken(metapath10Parser.KW_NE, 0); }
public TerminalNode KW_OR() { return getToken(metapath10Parser.KW_OR, 0); }
public TerminalNode KW_UNION() { return getToken(metapath10Parser.KW_UNION, 0); }
public EqnameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_eqname; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof metapath10Visitor ) return ((metapath10Visitor extends T>)visitor).visitEqname(this);
else return visitor.visitChildren(this);
}
}
public final EqnameContext eqname() throws RecognitionException {
EqnameContext _localctx = new EqnameContext(_ctx, getState());
enterRule(_localctx, 70, RULE_eqname);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(269);
_la = _input.LA(1);
if ( !((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << KW_AND) | (1L << KW_DIV) | (1L << KW_EMPTY_SEQUENCE) | (1L << KW_EQ) | (1L << KW_EXCEPT) | (1L << KW_GE) | (1L << KW_GT) | (1L << KW_IDIV) | (1L << KW_INTERSECT) | (1L << KW_LE) | (1L << KW_LT) | (1L << KW_MOD) | (1L << KW_NE) | (1L << KW_OR) | (1L << KW_UNION) | (1L << LocalName))) != 0)) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public boolean sempred(RuleContext _localctx, int ruleIndex, int predIndex) {
switch (ruleIndex) {
case 33:
return functioncall_sempred((FunctioncallContext)_localctx, predIndex);
}
return true;
}
private boolean functioncall_sempred(FunctioncallContext _localctx, int predIndex) {
switch (predIndex) {
case 0:
return !(
getInputStream().LA(1)==KW_EMPTY_SEQUENCE
) ;
}
return true;
}
public static final String _serializedATN =
"\3\u608b\ua72a\u8133\ub9ed\u417c\u3be7\u7786\u5964\3<\u0112\4\2\t\2\4"+
"\3\t\3\4\4\t\4\4\5\t\5\4\6\t\6\4\7\t\7\4\b\t\b\4\t\t\t\4\n\t\n\4\13\t"+
"\13\4\f\t\f\4\r\t\r\4\16\t\16\4\17\t\17\4\20\t\20\4\21\t\21\4\22\t\22"+
"\4\23\t\23\4\24\t\24\4\25\t\25\4\26\t\26\4\27\t\27\4\30\t\30\4\31\t\31"+
"\4\32\t\32\4\33\t\33\4\34\t\34\4\35\t\35\4\36\t\36\4\37\t\37\4 \t \4!"+
"\t!\4\"\t\"\4#\t#\4$\t$\4%\t%\3\2\3\2\3\2\3\3\3\3\3\3\7\3Q\n\3\f\3\16"+
"\3T\13\3\3\4\3\4\3\5\3\5\3\5\7\5[\n\5\f\5\16\5^\13\5\3\6\3\6\3\6\7\6c"+
"\n\6\f\6\16\6f\13\6\3\7\3\7\3\7\5\7k\n\7\3\7\3\7\5\7o\n\7\3\b\3\b\3\b"+
"\7\bt\n\b\f\b\16\bw\13\b\3\t\3\t\3\t\7\t|\n\t\f\t\16\t\177\13\t\3\n\3"+
"\n\3\n\7\n\u0084\n\n\f\n\16\n\u0087\13\n\3\13\3\13\3\13\7\13\u008c\n\13"+
"\f\13\16\13\u008f\13\13\3\f\3\f\3\f\7\f\u0094\n\f\f\f\16\f\u0097\13\f"+
"\3\r\3\r\3\r\3\r\3\r\7\r\u009e\n\r\f\r\16\r\u00a1\13\r\3\16\7\16\u00a4"+
"\n\16\f\16\16\16\u00a7\13\16\3\16\3\16\3\17\3\17\3\20\3\20\3\21\3\21\3"+
"\22\3\22\5\22\u00b3\n\22\3\22\3\22\3\22\5\22\u00b8\n\22\3\23\3\23\3\23"+
"\7\23\u00bd\n\23\f\23\16\23\u00c0\13\23\3\24\3\24\5\24\u00c4\n\24\3\25"+
"\3\25\3\25\3\26\5\26\u00ca\n\26\3\26\3\26\3\27\3\27\5\27\u00d0\n\27\3"+
"\30\3\30\3\31\3\31\7\31\u00d6\n\31\f\31\16\31\u00d9\13\31\3\32\3\32\3"+
"\32\3\32\7\32\u00df\n\32\f\32\16\32\u00e2\13\32\5\32\u00e4\n\32\3\32\3"+
"\32\3\33\7\33\u00e9\n\33\f\33\16\33\u00ec\13\33\3\34\3\34\3\34\3\34\3"+
"\35\3\35\5\35\u00f4\n\35\3\36\3\36\3\36\3\36\5\36\u00fa\n\36\3\37\3\37"+
"\5\37\u00fe\n\37\3 \3 \3!\3!\5!\u0104\n!\3!\3!\3\"\3\"\3#\3#\3#\3#\3$"+
"\3$\3%\3%\3%\2\2&\2\4\6\b\n\f\16\20\22\24\26\30\32\34\36 \"$&(*,.\60\62"+
"\64\668:<>@BDFH\2\13\4\2\30\30\36\36\6\2%%\'\'--\61\61\4\2\35\35\64\64"+
"\4\2**..\6\2\21\22\24\25\27\27\31\31\6\2))+,/\60\62\62\3\2#$\3\2\65\67"+
"\4\2&\64::\2\u010a\2J\3\2\2\2\4M\3\2\2\2\6U\3\2\2\2\bW\3\2\2\2\n_\3\2"+
"\2\2\fg\3\2\2\2\16p\3\2\2\2\20x\3\2\2\2\22\u0080\3\2\2\2\24\u0088\3\2"+
"\2\2\26\u0090\3\2\2\2\30\u0098\3\2\2\2\32\u00a5\3\2\2\2\34\u00aa\3\2\2"+
"\2\36\u00ac\3\2\2\2 \u00ae\3\2\2\2\"\u00b7\3\2\2\2$\u00b9\3\2\2\2&\u00c3"+
"\3\2\2\2(\u00c5\3\2\2\2*\u00c9\3\2\2\2,\u00cf\3\2\2\2.\u00d1\3\2\2\2\60"+
"\u00d3\3\2\2\2\62\u00da\3\2\2\2\64\u00ea\3\2\2\2\66\u00ed\3\2\2\28\u00f3"+
"\3\2\2\2:\u00f9\3\2\2\2<\u00fd\3\2\2\2>\u00ff\3\2\2\2@\u0101\3\2\2\2B"+
"\u0107\3\2\2\2D\u0109\3\2\2\2F\u010d\3\2\2\2H\u010f\3\2\2\2JK\5\4\3\2"+
"KL\7\2\2\3L\3\3\2\2\2MR\5\6\4\2NO\7\n\2\2OQ\5\6\4\2PN\3\2\2\2QT\3\2\2"+
"\2RP\3\2\2\2RS\3\2\2\2S\5\3\2\2\2TR\3\2\2\2UV\5\b\5\2V\7\3\2\2\2W\\\5"+
"\n\6\2XY\7\63\2\2Y[\5\n\6\2ZX\3\2\2\2[^\3\2\2\2\\Z\3\2\2\2\\]\3\2\2\2"+
"]\t\3\2\2\2^\\\3\2\2\2_d\5\f\7\2`a\7&\2\2ac\5\f\7\2b`\3\2\2\2cf\3\2\2"+
"\2db\3\2\2\2de\3\2\2\2e\13\3\2\2\2fd\3\2\2\2gn\5\16\b\2hk\5 \21\2ik\5"+
"\36\20\2jh\3\2\2\2ji\3\2\2\2kl\3\2\2\2lm\5\16\b\2mo\3\2\2\2nj\3\2\2\2"+
"no\3\2\2\2o\r\3\2\2\2pu\5\20\t\2qr\7 \2\2rt\5\20\t\2sq\3\2\2\2tw\3\2\2"+
"\2us\3\2\2\2uv\3\2\2\2v\17\3\2\2\2wu\3\2\2\2x}\5\22\n\2yz\t\2\2\2z|\5"+
"\22\n\2{y\3\2\2\2|\177\3\2\2\2}{\3\2\2\2}~\3\2\2\2~\21\3\2\2\2\177}\3"+
"\2\2\2\u0080\u0085\5\24\13\2\u0081\u0082\t\3\2\2\u0082\u0084\5\24\13\2"+
"\u0083\u0081\3\2\2\2\u0084\u0087\3\2\2\2\u0085\u0083\3\2\2\2\u0085\u0086"+
"\3\2\2\2\u0086\23\3\2\2\2\u0087\u0085\3\2\2\2\u0088\u008d\5\26\f\2\u0089"+
"\u008a\t\4\2\2\u008a\u008c\5\26\f\2\u008b\u0089\3\2\2\2\u008c\u008f\3"+
"\2\2\2\u008d\u008b\3\2\2\2\u008d\u008e\3\2\2\2\u008e\25\3\2\2\2\u008f"+
"\u008d\3\2\2\2\u0090\u0095\5\30\r\2\u0091\u0092\t\5\2\2\u0092\u0094\5"+
"\30\r\2\u0093\u0091\3\2\2\2\u0094\u0097\3\2\2\2\u0095\u0093\3\2\2\2\u0095"+
"\u0096\3\2\2\2\u0096\27\3\2\2\2\u0097\u0095\3\2\2\2\u0098\u009f\5\32\16"+
"\2\u0099\u009a\7\20\2\2\u009a\u009b\58\35\2\u009b\u009c\5\62\32\2\u009c"+
"\u009e\3\2\2\2\u009d\u0099\3\2\2\2\u009e\u00a1\3\2\2\2\u009f\u009d\3\2"+
"\2\2\u009f\u00a0\3\2\2\2\u00a0\31\3\2\2\2\u00a1\u009f\3\2\2\2\u00a2\u00a4"+
"\t\2\2\2\u00a3\u00a2\3\2\2\2\u00a4\u00a7\3\2\2\2\u00a5\u00a3\3\2\2\2\u00a5"+
"\u00a6\3\2\2\2\u00a6\u00a8\3\2\2\2\u00a7\u00a5\3\2\2\2\u00a8\u00a9\5\34"+
"\17\2\u00a9\33\3\2\2\2\u00aa\u00ab\5\"\22\2\u00ab\35\3\2\2\2\u00ac\u00ad"+
"\t\6\2\2\u00ad\37\3\2\2\2\u00ae\u00af\t\7\2\2\u00af!\3\2\2\2\u00b0\u00b2"+
"\7#\2\2\u00b1\u00b3\5$\23\2\u00b2\u00b1\3\2\2\2\u00b2\u00b3\3\2\2\2\u00b3"+
"\u00b8\3\2\2\2\u00b4\u00b5\7$\2\2\u00b5\u00b8\5$\23\2\u00b6\u00b8\5$\23"+
"\2\u00b7\u00b0\3\2\2\2\u00b7\u00b4\3\2\2\2\u00b7\u00b6\3\2\2\2\u00b8#"+
"\3\2\2\2\u00b9\u00be\5&\24\2\u00ba\u00bb\t\b\2\2\u00bb\u00bd\5&\24\2\u00bc"+
"\u00ba\3\2\2\2\u00bd\u00c0\3\2\2\2\u00be\u00bc\3\2\2\2\u00be\u00bf\3\2"+
"\2\2\u00bf%\3\2\2\2\u00c0\u00be\3\2\2\2\u00c1\u00c4\5\60\31\2\u00c2\u00c4"+
"\5(\25\2\u00c3\u00c1\3\2\2\2\u00c3\u00c2\3\2\2\2\u00c4\'\3\2\2\2\u00c5"+
"\u00c6\5*\26\2\u00c6\u00c7\5\64\33\2\u00c7)\3\2\2\2\u00c8\u00ca\7\3\2"+
"\2\u00c9\u00c8\3\2\2\2\u00c9\u00ca\3\2\2\2\u00ca\u00cb\3\2\2\2\u00cb\u00cc"+
"\5,\27\2\u00cc+\3\2\2\2\u00cd\u00d0\5H%\2\u00ce\u00d0\5.\30\2\u00cf\u00cd"+
"\3\2\2\2\u00cf\u00ce\3\2\2\2\u00d0-\3\2\2\2\u00d1\u00d2\7%\2\2\u00d2/"+
"\3\2\2\2\u00d3\u00d7\5:\36\2\u00d4\u00d6\5\66\34\2\u00d5\u00d4\3\2\2\2"+
"\u00d6\u00d9\3\2\2\2\u00d7\u00d5\3\2\2\2\u00d7\u00d8\3\2\2\2\u00d8\61"+
"\3\2\2\2\u00d9\u00d7\3\2\2\2\u00da\u00e3\7\34\2\2\u00db\u00e0\5F$\2\u00dc"+
"\u00dd\7\n\2\2\u00dd\u00df\5F$\2\u00de\u00dc\3\2\2\2\u00df\u00e2\3\2\2"+
"\2\u00e0\u00de\3\2\2\2\u00e0\u00e1\3\2\2\2\u00e1\u00e4\3\2\2\2\u00e2\u00e0"+
"\3\2\2\2\u00e3\u00db\3\2\2\2\u00e3\u00e4\3\2\2\2\u00e4\u00e5\3\2\2\2\u00e5"+
"\u00e6\7\13\2\2\u00e6\63\3\2\2\2\u00e7\u00e9\5\66\34\2\u00e8\u00e7\3\2"+
"\2\2\u00e9\u00ec\3\2\2\2\u00ea\u00e8\3\2\2\2\u00ea\u00eb\3\2\2\2\u00eb"+
"\65\3\2\2\2\u00ec\u00ea\3\2\2\2\u00ed\u00ee\7\32\2\2\u00ee\u00ef\5\4\3"+
"\2\u00ef\u00f0\7\5\2\2\u00f0\67\3\2\2\2\u00f1\u00f4\5H%\2\u00f2\u00f4"+
"\5@!\2\u00f3\u00f1\3\2\2\2\u00f3\u00f2\3\2\2\2\u00f49\3\2\2\2\u00f5\u00fa"+
"\5<\37\2\u00f6\u00fa\5@!\2\u00f7\u00fa\5B\"\2\u00f8\u00fa\5D#\2\u00f9"+
"\u00f5\3\2\2\2\u00f9\u00f6\3\2\2\2\u00f9\u00f7\3\2\2\2\u00f9\u00f8\3\2"+
"\2\2\u00fa;\3\2\2\2\u00fb\u00fe\5> \2\u00fc\u00fe\78\2\2\u00fd\u00fb\3"+
"\2\2\2\u00fd\u00fc\3\2\2\2\u00fe=\3\2\2\2\u00ff\u0100\t\t\2\2\u0100?\3"+
"\2\2\2\u0101\u0103\7\34\2\2\u0102\u0104\5\4\3\2\u0103\u0102\3\2\2\2\u0103"+
"\u0104\3\2\2\2\u0104\u0105\3\2\2\2\u0105\u0106\7\13\2\2\u0106A\3\2\2\2"+
"\u0107\u0108\7\r\2\2\u0108C\3\2\2\2\u0109\u010a\6#\2\2\u010a\u010b\5H"+
"%\2\u010b\u010c\5\62\32\2\u010cE\3\2\2\2\u010d\u010e\5\6\4\2\u010eG\3"+
"\2\2\2\u010f\u0110\t\n\2\2\u0110I\3\2\2\2\34R\\djnu}\u0085\u008d\u0095"+
"\u009f\u00a5\u00b2\u00b7\u00be\u00c3\u00c9\u00cf\u00d7\u00e0\u00e3\u00ea"+
"\u00f3\u00f9\u00fd\u0103";
public static final ATN _ATN =
new ATNDeserializer().deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
for (int i = 0; i < _ATN.getNumberOfDecisions(); i++) {
_decisionToDFA[i] = new DFA(_ATN.getDecisionState(i), i);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy