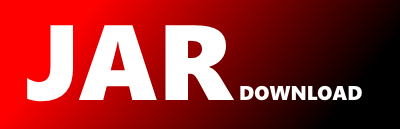
gov.sandia.cognition.learning.algorithm.clustering.initializer.RandomClusterInitializer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cognitive-foundry Show documentation
Show all versions of cognitive-foundry Show documentation
A single jar with all the Cognitive Foundry components.
/*
* File: RandomClusterInitializer.java
* Authors: Jeff Piersol
* Company: Sandia National Laboratories
* Project: Cognitive Foundry
*
* Copyright April 1, 2016, Sandia Corporation. Under the terms of Contract
* DE-AC04-94AL85000, there is a non-exclusive license for use of this work by
* or on behalf of the U.S. Government. Export of this program may require a
* license from the United States Government. See CopyrightHistory.txt for
* complete details.
*
*/
package gov.sandia.cognition.learning.algorithm.clustering.initializer;
import gov.sandia.cognition.learning.algorithm.clustering.cluster.Cluster;
import gov.sandia.cognition.learning.algorithm.clustering.cluster.ClusterCreator;
import gov.sandia.cognition.statistics.DiscreteSamplingUtil;
import gov.sandia.cognition.util.AbstractRandomized;
import gov.sandia.cognition.util.ObjectUtil;
import gov.sandia.cognition.util.Randomized;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Random;
import java.util.stream.Collectors;
/**
* Creates initial clusters by selecting random data points as singleton
* clusters.
*
* @param Type of {@link Cluster} used in this
* {@code learn()} method.
* @param The algorithm operates on a {@link Collection},
* so {@code DataType} will be something like Vector or String.
* @author Jeff Piersol
* @since 4.0.0
*/
public class RandomClusterInitializer, DataType>
extends AbstractRandomized
implements FixedClusterInitializer
{
/** The creator for new clusters. */
protected ClusterCreator creator;
/**
* Creates a new random cluster creator.
*
* @param creator the cluster creator to use
* @param random the random number generator to use
*/
public RandomClusterInitializer(
final ClusterCreator creator,
final Random random)
{
super(random);
this.creator = creator;
}
@SuppressWarnings("unchecked")
@Override
public RandomClusterInitializer clone()
{
RandomClusterInitializer clone
= (RandomClusterInitializer) super.clone();
clone.creator = ObjectUtil.cloneSmart(this.creator);
return clone;
}
@Override
public ArrayList initializeClusters(int numClusters,
Collection extends DataType> elements)
{
List extends DataType> elementsList = elements instanceof List
? (List extends DataType>) elements
: new ArrayList<>(elements);
ArrayList representativePoints
= DiscreteSamplingUtil.sampleWithReplacement(random, elementsList,
numClusters);
ArrayList clusters = representativePoints.stream()
.map(point -> creator.createCluster(Arrays.asList(point)))
.collect(Collectors.toCollection(ArrayList::new));
return clusters;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy