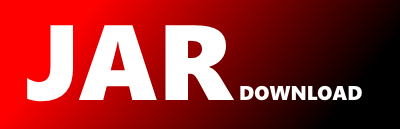
gr.iti.mklab.sfc.input.CollectionsManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mklab-stream-manager Show documentation
Show all versions of mklab-stream-manager Show documentation
Monitors a set of social streams (e.g. Twitter status updates) and collects the incoming content.
The newest version!
package gr.iti.mklab.sfc.input;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.logging.log4j.Logger;
import org.apache.logging.log4j.LogManager;
import org.mongodb.morphia.dao.BasicDAO;
import org.mongodb.morphia.query.Query;
import org.mongodb.morphia.query.QueryResults;
import gr.iti.mklab.framework.client.mongo.DAOFactory;
import gr.iti.mklab.framework.common.domain.collections.Collection;
import gr.iti.mklab.framework.common.domain.config.Configuration;
import gr.iti.mklab.framework.common.domain.feeds.Feed;
/**
* The class responsible for the creation of input feeds from mongodb storage
*
* @author manosetro - [email protected]
*/
public class CollectionsManager {
public final Logger logger = LogManager.getLogger(CollectionsManager.class);
protected static final String SINCE = "since";
protected static final String HOST = "mongo.host";
protected static final String DB = "mongo.database";
protected static final String USERNAME = "mongo.username";
protected static final String PWD = "mongo.password";
private String host = null;
private String db = null;
private String username = null;
private String password = null;
private BasicDAO collectionsDao;
public CollectionsManager(Configuration config) throws Exception {
this.host = config.getParameter(HOST);
this.db = config.getParameter(DB);
this.username = config.getParameter(USERNAME);
this.password = config.getParameter(PWD);
DAOFactory daoFactory = new DAOFactory();
if(username != null && password != null) {
collectionsDao = daoFactory.getDAO(host, db, Collection.class, username, password);
}
else {
collectionsDao = daoFactory.getDAO(host, db, Collection.class);
}
}
public Map getCollections() {
Map collections = new HashMap();
QueryResults result = collectionsDao.find();
Iterator it = result.iterator();
while(it.hasNext()) {
Collection collection = it.next();
collections.put(collection.getId(), collection);
}
return collections;
}
public Map getActiveCollections() {
Map collections = new HashMap();
Query query = collectionsDao.createQuery()
.filter("status", "running")
.order("-updateDate");
QueryResults result = collectionsDao.find(query);
Iterator it = result.iterator();
while(it.hasNext()) {
Collection collection = it.next();
collections.put(collection.getId(), collection);
}
return collections;
}
public Map> createFeedsPerSource() {
Map> feedsPerSource = new HashMap>();
Set allFeeds = createFeeds();
for(Feed feed : allFeeds) {
String source = feed.getSource();
Set feeds = feedsPerSource.get(source);
if(feeds == null) {
feeds = new HashSet();
feedsPerSource.put(source, feeds);
}
feeds.add(feed);
}
return feedsPerSource;
}
public Set createFeeds() {
Set feedsSet = new HashSet();
try {
QueryResults result = collectionsDao.find();
List collections = result.asList();
for(Collection collection : collections) {
List feeds = collection.getFeeds();
feedsSet.addAll(feeds);
}
}
catch(Exception e) {
logger.error(e);
}
return feedsSet;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy