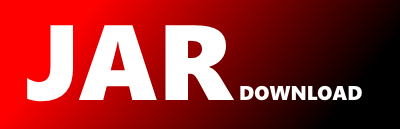
gr.james.simplegraph.BaseGraph Maven / Gradle / Ivy
Show all versions of simple-graph Show documentation
package gr.james.simplegraph;
import java.io.Serializable;
/**
* Base interface for all graph types.
*/
public interface BaseGraph extends Serializable {
/**
* Get the number of vertices in the graph.
*
* Because vertices are numbered in the range {@code [0, size)}, you can use this method in a typical for-loop to
* iterate over the vertices in the graph:
*
* for (int v = 0; v < g.size(); v++) {
* // Do something with v
* }
*
*
* Furthermore, the vertex IDs reflect the actual order at which they were inserted in the graph. So you can get the
* oldest and newest vertices like so:
*
* int oldestVertex = 0;
* int newestVertex = g.size() - 1;
*
*
* Complexity: O(1)
*
* @return how many vertices there are in the graph
*/
int size();
/**
* Returns a string representation of the graph.
*
* Complexity: O(V+E)
*
* @return a string representation of the graph
*/
@Override
String toString();
/**
* Indicates whether some other object is equal to this graph.
*
* Two graphs are equal if they are instances of the same base type ({@link Graph}, {@link DirectedGraph},
* {@link WeightedGraph}, {@link WeightedDirectedGraph}), have the same number of vertices and their edges represent
* the same mapping.
*
* Complexity: O(V+E)
*
* @param obj the reference object with which to compare
* @return {@code true} if this graph is equal to the {@code obj} argument, otherwise {@code false}
*/
@Override
boolean equals(Object obj);
/**
* Returns a hash code value for this graph.
*
* Complexity: O(V+E)
*
* @return a hash code value for this graph
*/
@Override
int hashCode();
}