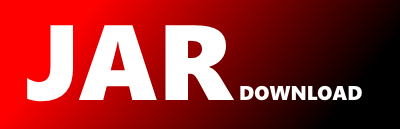
gr.james.simplegraph.BipartiteGraph Maven / Gradle / Ivy
Show all versions of simple-graph Show documentation
package gr.james.simplegraph;
import java.util.Set;
/**
* Represents an unweighted and undirected bipartite graph.
*
* A bipartite graph comprises of two independent sets of vertices, namely set {@code A} and set {@code B}. An edge can
* only connect one vertex in set {@code A} to one vertex in set {@code B}. Therefore, the graph cannot contain self
* loops.
*
* Furthermore, the graph cannot contain parallel edges. More formally, any unordered pair of endpoints may correspond
* to at most one edge.
*
* An unordered pair {@code {a, b}} is a pair of objects with no particular relation between them; the order in which
* the objects appear in the pair is not significant.
*
* Memory Complexity: O(V+E)
*/
public interface BipartiteGraph extends Graph {
/**
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
int size();
/**
* Returns a {@link Set} of all vertices in set {@code A}.
*
* Complexity: O(1)
*
* @return a {@link Set} of all vertices in set {@code A}
* @see #setB()
*/
Set setA();
/**
* Returns a {@link Set} of all vertices in set {@code B}.
*
* Complexity: O(1)
*
* @return a {@link Set} of all vertices in set {@code B}
* @see #setA()
*/
Set setB();
/**
* {@inheritDoc}
*
* @param v {@inheritDoc}
* @return {@inheritDoc}
* @throws IndexOutOfBoundsException {@inheritDoc}
*/
@Override
Set adjacent(int v);
/**
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
Iterable edges();
/**
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
DirectedGraph asDirected();
/**
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
WeightedGraph asWeighted();
/**
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
WeightedDirectedGraph asWeightedDirected();
/**
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
String toString();
/**
* {@inheritDoc}
*
* @param obj {@inheritDoc}
* @return {@inheritDoc}
*/
@Override
boolean equals(Object obj);
/**
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
int hashCode();
}