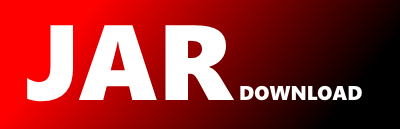
gr.james.simplegraph.examples.DepthFirstSearch Maven / Gradle / Ivy
Show all versions of simple-graph Show documentation
package gr.james.simplegraph.examples;
import gr.james.simplegraph.DirectedGraph;
import java.util.Deque;
import java.util.HashSet;
import java.util.LinkedList;
import java.util.Set;
/**
* Demonstration of depth first search (DFS) using a stack.
*/
public final class DepthFirstSearch {
private DepthFirstSearch() {
}
/**
* Performs DFS on a directed graph.
*
* Complexity: O(V+E)
*
* @param g the graph
* @param source the source vertex
* @return the number of vertices that are reachable from {@code source} (self included)
* @throws NullPointerException if {@code g} is {@code null}
* @throws IndexOutOfBoundsException if {@code source} is not in the graph
*/
public static int depthFirstSearch(DirectedGraph g, int source) {
final Deque stack = new LinkedList();
final Set visited = new HashSet();
stack.push(source);
while (!stack.isEmpty()) {
final int next = stack.pop();
if (visited.add(next)) {
for (int adj : g.adjacentOut(next)) {
stack.push(adj);
}
}
}
return visited.size();
}
}