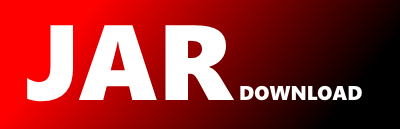
graphics.scenery.spirvcrossj.TShader Maven / Gradle / Ivy
/* ----------------------------------------------------------------------------
* This file was automatically generated by SWIG (http://www.swig.org).
* Version 3.0.9
*
* Do not make changes to this file unless you know what you are doing--modify
* the SWIG interface file instead.
* ----------------------------------------------------------------------------- */
package graphics.scenery.spirvcrossj;
public class TShader {
private transient long swigCPtr;
protected transient boolean swigCMemOwn;
protected TShader(long cPtr, boolean cMemoryOwn) {
swigCMemOwn = cMemoryOwn;
swigCPtr = cPtr;
}
protected static long getCPtr(TShader obj) {
return (obj == null) ? 0 : obj.swigCPtr;
}
protected void finalize() {
delete();
}
public synchronized void delete() {
if (swigCPtr != 0) {
if (swigCMemOwn) {
swigCMemOwn = false;
libspirvcrossjJNI.delete_TShader(swigCPtr);
}
swigCPtr = 0;
}
}
public TShader(int arg0) {
this(libspirvcrossjJNI.new_TShader(arg0), true);
}
public void setStrings(String[] s, int n) {
libspirvcrossjJNI.TShader_setStrings(swigCPtr, this, s, n);
}
public void setStringsWithLengths(String[] s, int[] l, int n) {
libspirvcrossjJNI.TShader_setStringsWithLengths(swigCPtr, this, s, l, n);
}
public void setStringsWithLengthsAndNames(String[] s, int[] l, String[] names, int n) {
libspirvcrossjJNI.TShader_setStringsWithLengthsAndNames(swigCPtr, this, s, l, names, n);
}
public void setPreamble(String s) {
libspirvcrossjJNI.TShader_setPreamble(swigCPtr, this, s);
}
public void setEntryPoint(String entryPoint) {
libspirvcrossjJNI.TShader_setEntryPoint(swigCPtr, this, entryPoint);
}
public void setSourceEntryPoint(String sourceEntryPointName) {
libspirvcrossjJNI.TShader_setSourceEntryPoint(swigCPtr, this, sourceEntryPointName);
}
public void addProcesses(StringVec arg0) {
libspirvcrossjJNI.TShader_addProcesses(swigCPtr, this, StringVec.getCPtr(arg0), arg0);
}
public void setShiftBinding(int res, long base) {
libspirvcrossjJNI.TShader_setShiftBinding(swigCPtr, this, res, base);
}
public void setShiftSamplerBinding(long base) {
libspirvcrossjJNI.TShader_setShiftSamplerBinding(swigCPtr, this, base);
}
public void setShiftTextureBinding(long base) {
libspirvcrossjJNI.TShader_setShiftTextureBinding(swigCPtr, this, base);
}
public void setShiftImageBinding(long base) {
libspirvcrossjJNI.TShader_setShiftImageBinding(swigCPtr, this, base);
}
public void setShiftUboBinding(long base) {
libspirvcrossjJNI.TShader_setShiftUboBinding(swigCPtr, this, base);
}
public void setShiftUavBinding(long base) {
libspirvcrossjJNI.TShader_setShiftUavBinding(swigCPtr, this, base);
}
public void setShiftCbufferBinding(long base) {
libspirvcrossjJNI.TShader_setShiftCbufferBinding(swigCPtr, this, base);
}
public void setShiftSsboBinding(long base) {
libspirvcrossjJNI.TShader_setShiftSsboBinding(swigCPtr, this, base);
}
public void setShiftBindingForSet(int res, long base, long set) {
libspirvcrossjJNI.TShader_setShiftBindingForSet(swigCPtr, this, res, base, set);
}
public void setResourceSetBinding(StringVec base) {
libspirvcrossjJNI.TShader_setResourceSetBinding(swigCPtr, this, StringVec.getCPtr(base), base);
}
public void setAutoMapBindings(boolean map) {
libspirvcrossjJNI.TShader_setAutoMapBindings(swigCPtr, this, map);
}
public void setAutoMapLocations(boolean map) {
libspirvcrossjJNI.TShader_setAutoMapLocations(swigCPtr, this, map);
}
public void setInvertY(boolean invert) {
libspirvcrossjJNI.TShader_setInvertY(swigCPtr, this, invert);
}
public void setHlslIoMapping(boolean hlslIoMap) {
libspirvcrossjJNI.TShader_setHlslIoMapping(swigCPtr, this, hlslIoMap);
}
public void setFlattenUniformArrays(boolean flatten) {
libspirvcrossjJNI.TShader_setFlattenUniformArrays(swigCPtr, this, flatten);
}
public void setNoStorageFormat(boolean useUnknownFormat) {
libspirvcrossjJNI.TShader_setNoStorageFormat(swigCPtr, this, useUnknownFormat);
}
public void setTextureSamplerTransformMode(int mode) {
libspirvcrossjJNI.TShader_setTextureSamplerTransformMode(swigCPtr, this, mode);
}
public void setEnvInput(int lang, int envStage, int client, int version) {
libspirvcrossjJNI.TShader_setEnvInput(swigCPtr, this, lang, envStage, client, version);
}
public void setEnvClient(int client, int version) {
libspirvcrossjJNI.TShader_setEnvClient(swigCPtr, this, client, version);
}
public void setEnvTarget(int lang, int version) {
libspirvcrossjJNI.TShader_setEnvTarget(swigCPtr, this, lang, version);
}
public void setEnvTargetHlslFunctionality1() {
libspirvcrossjJNI.TShader_setEnvTargetHlslFunctionality1(swigCPtr, this);
}
public boolean getEnvTargetHlslFunctionality1() {
return libspirvcrossjJNI.TShader_getEnvTargetHlslFunctionality1(swigCPtr, this);
}
static public class Includer {
private transient long swigCPtr;
protected transient boolean swigCMemOwn;
protected Includer(long cPtr, boolean cMemoryOwn) {
swigCMemOwn = cMemoryOwn;
swigCPtr = cPtr;
}
protected static long getCPtr(Includer obj) {
return (obj == null) ? 0 : obj.swigCPtr;
}
protected void finalize() {
delete();
}
public synchronized void delete() {
if (swigCPtr != 0) {
if (swigCMemOwn) {
swigCMemOwn = false;
libspirvcrossjJNI.delete_TShader_Includer(swigCPtr);
}
swigCPtr = 0;
}
}
static public class IncludeResult {
private transient long swigCPtr;
protected transient boolean swigCMemOwn;
protected IncludeResult(long cPtr, boolean cMemoryOwn) {
swigCMemOwn = cMemoryOwn;
swigCPtr = cPtr;
}
protected static long getCPtr(IncludeResult obj) {
return (obj == null) ? 0 : obj.swigCPtr;
}
protected void finalize() {
delete();
}
public synchronized void delete() {
if (swigCPtr != 0) {
if (swigCMemOwn) {
swigCMemOwn = false;
libspirvcrossjJNI.delete_TShader_Includer_IncludeResult(swigCPtr);
}
swigCPtr = 0;
}
}
public IncludeResult(String headerName, String headerData, long headerLength, SWIGTYPE_p_void userData) {
this(libspirvcrossjJNI.new_TShader_Includer_IncludeResult(headerName, headerData, headerLength, SWIGTYPE_p_void.getCPtr(userData)), true);
}
public String getHeaderName() {
return libspirvcrossjJNI.TShader_Includer_IncludeResult_headerName_get(swigCPtr, this);
}
public String getHeaderData() {
return libspirvcrossjJNI.TShader_Includer_IncludeResult_headerData_get(swigCPtr, this);
}
public long getHeaderLength() {
return libspirvcrossjJNI.TShader_Includer_IncludeResult_headerLength_get(swigCPtr, this);
}
public void setUserData(SWIGTYPE_p_void value) {
libspirvcrossjJNI.TShader_Includer_IncludeResult_userData_set(swigCPtr, this, SWIGTYPE_p_void.getCPtr(value));
}
public SWIGTYPE_p_void getUserData() {
long cPtr = libspirvcrossjJNI.TShader_Includer_IncludeResult_userData_get(swigCPtr, this);
return (cPtr == 0) ? null : new SWIGTYPE_p_void(cPtr, false);
}
}
public TShader.Includer.IncludeResult includeSystem(String arg0, String arg1, long arg2) {
long cPtr = libspirvcrossjJNI.TShader_Includer_includeSystem(swigCPtr, this, arg0, arg1, arg2);
return (cPtr == 0) ? null : new TShader.Includer.IncludeResult(cPtr, false);
}
public TShader.Includer.IncludeResult includeLocal(String arg0, String arg1, long arg2) {
long cPtr = libspirvcrossjJNI.TShader_Includer_includeLocal(swigCPtr, this, arg0, arg1, arg2);
return (cPtr == 0) ? null : new TShader.Includer.IncludeResult(cPtr, false);
}
public void releaseInclude(TShader.Includer.IncludeResult arg0) {
libspirvcrossjJNI.TShader_Includer_releaseInclude(swigCPtr, this, TShader.Includer.IncludeResult.getCPtr(arg0), arg0);
}
}
static public class ForbidIncluder extends TShader.Includer {
private transient long swigCPtr;
protected ForbidIncluder(long cPtr, boolean cMemoryOwn) {
super(libspirvcrossjJNI.TShader_ForbidIncluder_SWIGUpcast(cPtr), cMemoryOwn);
swigCPtr = cPtr;
}
protected static long getCPtr(ForbidIncluder obj) {
return (obj == null) ? 0 : obj.swigCPtr;
}
protected void finalize() {
delete();
}
public synchronized void delete() {
if (swigCPtr != 0) {
if (swigCMemOwn) {
swigCMemOwn = false;
libspirvcrossjJNI.delete_TShader_ForbidIncluder(swigCPtr);
}
swigCPtr = 0;
}
super.delete();
}
public void releaseInclude(TShader.Includer.IncludeResult arg0) {
libspirvcrossjJNI.TShader_ForbidIncluder_releaseInclude(swigCPtr, this, TShader.Includer.IncludeResult.getCPtr(arg0), arg0);
}
public ForbidIncluder() {
this(libspirvcrossjJNI.new_TShader_ForbidIncluder(), true);
}
}
public boolean parse(SWIGTYPE_p_TBuiltInResource arg0, int defaultVersion, int defaultProfile, boolean forceDefaultVersionAndProfile, boolean forwardCompatible, int arg5, TShader.Includer arg6) {
return libspirvcrossjJNI.TShader_parse__SWIG_0(swigCPtr, this, SWIGTYPE_p_TBuiltInResource.getCPtr(arg0), defaultVersion, defaultProfile, forceDefaultVersionAndProfile, forwardCompatible, arg5, TShader.Includer.getCPtr(arg6), arg6);
}
public boolean parse(SWIGTYPE_p_TBuiltInResource res, int defaultVersion, int defaultProfile, boolean forceDefaultVersionAndProfile, boolean forwardCompatible, int messages) {
return libspirvcrossjJNI.TShader_parse__SWIG_1(swigCPtr, this, SWIGTYPE_p_TBuiltInResource.getCPtr(res), defaultVersion, defaultProfile, forceDefaultVersionAndProfile, forwardCompatible, messages);
}
public boolean parse(SWIGTYPE_p_TBuiltInResource builtInResources, int defaultVersion, boolean forwardCompatible, int messages) {
return libspirvcrossjJNI.TShader_parse__SWIG_2(swigCPtr, this, SWIGTYPE_p_TBuiltInResource.getCPtr(builtInResources), defaultVersion, forwardCompatible, messages);
}
public boolean parse(SWIGTYPE_p_TBuiltInResource builtInResources, int defaultVersion, boolean forwardCompatible, int messages, TShader.Includer includer) {
return libspirvcrossjJNI.TShader_parse__SWIG_3(swigCPtr, this, SWIGTYPE_p_TBuiltInResource.getCPtr(builtInResources), defaultVersion, forwardCompatible, messages, TShader.Includer.getCPtr(includer), includer);
}
public boolean preprocess(SWIGTYPE_p_TBuiltInResource builtInResources, int defaultVersion, int defaultProfile, boolean forceDefaultVersionAndProfile, boolean forwardCompatible, int message, java.lang.String[] outputString, TShader.Includer includer) {
return libspirvcrossjJNI.TShader_preprocess(swigCPtr, this, SWIGTYPE_p_TBuiltInResource.getCPtr(builtInResources), defaultVersion, defaultProfile, forceDefaultVersionAndProfile, forwardCompatible, message, outputString, TShader.Includer.getCPtr(includer), includer);
}
public String getInfoLog() {
return libspirvcrossjJNI.TShader_getInfoLog(swigCPtr, this);
}
public String getInfoDebugLog() {
return libspirvcrossjJNI.TShader_getInfoDebugLog(swigCPtr, this);
}
public int getStage() {
return libspirvcrossjJNI.TShader_getStage(swigCPtr, this);
}
public SWIGTYPE_p_glslang__TIntermediate getIntermediate() {
long cPtr = libspirvcrossjJNI.TShader_getIntermediate(swigCPtr, this);
return (cPtr == 0) ? null : new SWIGTYPE_p_glslang__TIntermediate(cPtr, false);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy