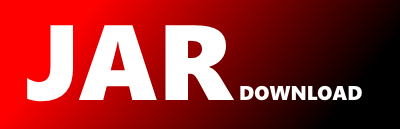
group.flyfish.rest.constants.RestConstants Maven / Gradle / Ivy
package group.flyfish.rest.constants;
import group.flyfish.rest.core.ThreadPoolManager;
import group.flyfish.rest.core.builder.TypedMapBuilder;
import group.flyfish.rest.core.resolver.*;
import group.flyfish.rest.enums.HttpMethod;
import group.flyfish.rest.enums.ResponseType;
import org.apache.http.client.config.RequestConfig;
import java.util.Map;
import java.util.concurrent.ExecutorService;
/**
* rest客户端需要的常量都在这里
*
* @author wangyu
*/
public interface RestConstants {
/**
* 请求配置
*/
RequestConfig REQUEST_CONFIG = RequestConfig.custom().setConnectTimeout(3000).build();
/**
* 请求解析器
*/
Map RESOLVER_MAP = resolverBuilder()
.with(HttpMethod.GET, new HttpGetResolver())
.with(HttpMethod.POST, new HttpPostResolver())
.with(HttpMethod.PUT, new HttpPutResolver())
.with(HttpMethod.PATCH, new HttpPatchResolver())
.with(HttpMethod.DELETE, new HttpDeleteResolver())
.build();
/**
* 线程池
*/
ExecutorService DEFAULT_EXECUTOR = ThreadPoolManager.defaultCachedThreadPool();
/**
* 使用的MIME,后缀映射
* 自定义的MIME TYPE,可以过滤
*/
Map MIME_MAP = TypedMapBuilder.stringMapBuilder()
.with("ai", "application/postscript")
.with("aif", "audio/x-aiff")
.with("aifc", "audio/x-aiff")
.with("aiff", "audio/x-aiff")
.with("asc", "text/plain")
.with("au", "audio/basic")
.with("avi", "video/x-msvideo")
.with("bcpio", "application/x-bcpio")
.with("bin", "application/octet-stream")
.with("c", "text/plain")
.with("cc", "text/plain")
.with("ccad", "application/clariscad")
.with("cdf", "application/x-netcdf")
.with("class", "application/octet-stream")
.with("cpio", "application/x-cpio")
.with("cpt", "application/mac-compactpro")
.with("csh", "application/x-csh")
.with("css", "text/css")
.with("dcr", "application/x-director")
.with("dir", "application/x-director")
.with("dms", "application/octet-stream")
.with("doc", "application/msword")
.with("drw", "application/drafting")
.with("dvi", "application/x-dvi")
.with("dwg", "application/acad")
.with("dxf", "application/dxf")
.with("dxr", "application/x-director")
.with("eps", "application/postscript")
.with("etx", "text/x-setext")
.with("exe", "application/octet-stream")
.with("ez", "application/andrew-inset")
.with("f", "text/plain")
.with("f90", "text/plain")
.with("fli", "video/x-fli")
.with("gif", "image/gif")
.with("gtar", "application/x-gtar")
.with("gz", "application/x-gzip")
.with("h", "text/plain")
.with("hdf", "application/x-hdf")
.with("hh", "text/plain")
.with("hqx", "application/mac-binhex40")
.with("htm", "text/html")
.with("html", "text/html")
.with("ice", "x-conference/x-cooltalk")
.with("ief", "image/ief")
.with("iges", "model/iges")
.with("igs", "model/iges")
.with("ips", "application/x-ipscript")
.with("ipx", "application/x-ipix")
.with("jpe", "image/jpeg")
.with("jpeg", "image/jpeg")
.with("jpg", "image/jpeg")
.with("js", "application/x-javascript")
.with("kar", "audio/midi")
.with("latex", "application/x-latex")
.with("lha", "application/octet-stream")
.with("lsp", "application/x-lisp")
.with("lzh", "application/octet-stream")
.with("m", "text/plain")
.with("man", "application/x-troff-man")
.with("me", "application/x-troff-me")
.with("mesh", "model/mesh")
.with("mid", "audio/midi")
.with("midi", "audio/midi")
.with("mif", "application/vnd.mif")
.with("mime", "www/mime")
.with("mov", "video/quicktime")
.with("movie", "video/x-sgi-movie")
.with("mp2", "audio/mpeg")
.with("mp3", "audio/mpeg")
.with("mp4", "video/mpeg")
.with("mpe", "video/mpeg")
.with("mpeg", "video/mpeg")
.with("mpg", "video/mpeg")
.with("mpga", "audio/mpeg")
.with("ms", "application/x-troff-ms")
.with("msh", "model/mesh")
.with("nc", "application/x-netcdf")
.with("oda", "application/oda")
.with("pbm", "image/x-portable-bitmap")
.with("pdb", "chemical/x-pdb")
.with("pdf", "application/pdf")
.with("pgm", "image/x-portable-graymap")
.with("pgn", "application/x-chess-pgn")
.with("png", "image/png")
.with("pnm", "image/x-portable-anymap")
.with("pot", "application/mspowerpoint")
.with("ppm", "image/x-portable-pixmap")
.with("pps", "application/mspowerpoint")
.with("ppt", "application/mspowerpoint")
.with("ppz", "application/mspowerpoint")
.with("pre", "application/x-freelance")
.with("prt", "application/pro_eng")
.with("ps", "application/postscript")
.with("qt", "video/quicktime")
.with("ra", "audio/x-realaudio")
.with("ram", "audio/x-pn-realaudio")
.with("ras", "image/cmu-raster")
.with("rgb", "image/x-rgb")
.with("rm", "audio/x-pn-realaudio")
.with("roff", "application/x-troff")
.with("rpm", "audio/x-pn-realaudio-plugin")
.with("rtf", "text/rtf")
.with("rtx", "text/richtext")
.with("scm", "application/x-lotusscreencam")
.with("set", "application/set")
.with("sgm", "text/sgml")
.with("sgml", "text/sgml")
.with("sh", "application/x-sh")
.with("shar", "application/x-shar")
.with("silo", "model/mesh")
.with("sit", "application/x-stuffit")
.with("skd", "application/x-koan")
.with("skm", "application/x-koan")
.with("skp", "application/x-koan")
.with("skt", "application/x-koan")
.with("smi", "application/smil")
.with("smil", "application/smil")
.with("snd", "audio/basic")
.with("sol", "application/solids")
.with("spl", "application/x-futuresplash")
.with("src", "application/x-wais-source")
.with("step", "application/STEP")
.with("stl", "application/SLA")
.with("stp", "application/STEP")
.with("sv4cpio", "application/x-sv4cpio")
.with("sv4crc", "application/x-sv4crc")
.with("swf", "application/x-shockwave-flash")
.with("t", "application/x-troff")
.with("tar", "application/x-tar")
.with("tcl", "application/x-tcl")
.with("tex", "application/x-tex")
.with("texi", "application/x-texinfo")
.with("texinfo", "application/x-texinfo")
.with("tif", "image/tiff")
.with("tiff", "image/tiff")
.with("tr", "application/x-troff")
.with("tsi", "audio/TSP-audio")
.with("tsp", "application/dsptype")
.with("tsv", "text/tab-separated-values")
.with("txt", "text/plain")
.with("unv", "application/i-deas")
.with("ustar", "application/x-ustar")
.with("vcd", "application/x-cdlink")
.with("vda", "application/vda")
.with("viv", "video/vnd.vivo")
.with("vivo", "video/vnd.vivo")
.with("vrml", "model/vrml")
.with("wav", "audio/x-wav")
.with("wrl", "model/vrml")
.with("xbm", "image/x-xbitmap")
.with("xlc", "application/vnd.ms-excel")
.with("xll", "application/vnd.ms-excel")
.with("xlm", "application/vnd.ms-excel")
.with("xls", "application/vnd.ms-excel")
.with("xlw", "application/vnd.ms-excel")
.with("xml", "text/xml")
.with("xpm", "image/x-xpixmap")
.with("xwd", "image/x-xwindowdump")
.with("xyz", "chemical/x-pdb")
.with("zip", "application/zip ")
.with("apk", "application/vnd.android.package-archive")
.with("*", "application/octet-stream")
.build();
static TypedMapBuilder resolverBuilder() {
return TypedMapBuilder.builder();
}
// 响应类型映射
Map RESPONSE_TYPE_MAP = TypedMapBuilder.builder()
.with(TypeConstants.STRING, ResponseType.TEXT)
.with(TypeConstants.BYTE_ARRAY, ResponseType.BINARY)
.build();
// 提示消息
String MSG_THREAD_POOL_EMPTY = "线程池未指定或为空!";
String MSG_IO_ERROR = "发起请求时出现异常!";
String MSG_UNKNOWN_HOST = "未知的请求地址!";
String MSG_REQUEST_ERROR = "请求接口{0}状态异常!代码:{1}";
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy