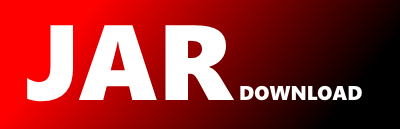
group.flyfish.rest.utils.JacksonUtil Maven / Gradle / Ivy
package group.flyfish.rest.utils;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.core.JsonGenerator;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.JavaType;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Optional;
/**
* Jackson序列化工具类
*
* @author Mr.Wang
*/
public final class JacksonUtil {
private static final ObjectMapper mapper = new ObjectMapper();
static {
// =========================================================================
// SerializationFeature for changing how JSON is written
// to enable standard indentation ("pretty-printing"):
// mapper.enable(SerializationFeature.INDENT_OUTPUT);
// to allow serialization of "empty" POJOs (no properties to serialize)
// (without this setting, an exception is thrown in those cases)
mapper.disable(SerializationFeature.FAIL_ON_EMPTY_BEANS);
// to write java.util.Date, Calendar as number (timestamp):
mapper.disable(SerializationFeature.WRITE_DATES_AS_TIMESTAMPS);
// DeserializationFeature for changing how JSON is read as POJOs:
// to prevent exception when encountering unknown property:
mapper.disable(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES);
// to allow coercion of JSON empty String ("") to null Object value:
mapper.enable(DeserializationFeature.ACCEPT_EMPTY_STRING_AS_NULL_OBJECT);
// =========================================================================
// JsonParser.Feature for configuring parsing settings:
// to allow C/C++ style comments in JSON (non-standard, disabled by default)
mapper.setDateFormat(new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"));
// (note: with Jackson 2.5, there is also `mapper.enable(feature)` / `mapper.disable(feature)`)
mapper.configure(JsonParser.Feature.ALLOW_COMMENTS, true);
// to allow (non-standard) unquoted field names in JSON:
mapper.configure(JsonParser.Feature.ALLOW_UNQUOTED_FIELD_NAMES, true);
// to allow use of apostrophes (single quotes), non standard
mapper.configure(JsonParser.Feature.ALLOW_SINGLE_QUOTES, true);
mapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
// JsonGenerator.Feature for configuring low-level JSON generation:
// to force escaping of non-ASCII characters:
mapper.configure(JsonGenerator.Feature.ESCAPE_NON_ASCII, true);
}
public static T fromJson(final String json) {
return readValue(json, new TypeReference() {
});
}
public static T fromJson(final String json, TypeReference reference) {
return readValue(json, reference);
}
public static T fromJson(final String json, Class clazz) {
if (null == json || "".equals(json)) {
return null;
}
try {
return mapper.readValue(json, clazz);
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
public static T fromJson(final String json, JavaType type) {
if (null == json || "".equals(json)) {
return null;
}
try {
return mapper.readValue(json, type);
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
// public static Map json2Map(final String json) {
// return fromJson(json, Map.class);
// }
public static Map json2Map(String json) {
return readValue(json, new TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy