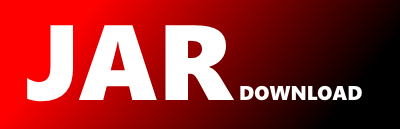
guru.mocker.java.api.MockerBase Maven / Gradle / Ivy
The newest version!
package guru.mocker.java.api;
import guru.mocker.java.api.annotation.UseCase;
import guru.mocker.java.internal.CallableMethod;
import guru.mocker.java.internal.api.DefaultMockerFactory;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Arrays;
import java.util.Optional;
import java.util.concurrent.Callable;
import static guru.mocker.java.api.util.TestFrameworkUtil.unWrapArguments;
public class MockerBase implements GivenMocker
{
private final MockerContext mockerContext;
public MockerBase()
{
this(new DefaultMockerFactory());
}
protected MockerBase(MockerFactory mockerFactory)
{
mockerContext = mockerFactory.createMockerContext();
}
public void addAssert(Runnable... runnables)
{
mockerContext.asserts().addAll(Arrays.asList(runnables));
}
public void addMethods(Runnable mockRunnable, Runnable realRunnable)
{
addMethods(convertRunnableToCallable(mockRunnable), convertRunnableToCallable(realRunnable));
}
public void addMethods(Callable
© 2015 - 2024 Weber Informatics LLC | Privacy Policy