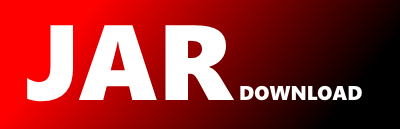
hm.binkley.corba.CORBAHelper Maven / Gradle / Ivy
The newest version!
package hm.binkley.corba;
import org.omg.CORBA.ORB;
import org.omg.CORBA.ORBPackage.InvalidName;
import org.omg.CosNaming.NamingContextExt;
import org.omg.CosNaming.NamingContextExtHelper;
import org.omg.CosNaming.NamingContextPackage.CannotProceed;
import org.omg.CosNaming.NamingContextPackage.NotFound;
import org.omg.PortableServer.POA;
import org.omg.PortableServer.POAHelper;
import org.omg.PortableServer.POAManagerPackage.AdapterInactive;
import org.omg.PortableServer.POAPackage.ServantNotActive;
import org.omg.PortableServer.POAPackage.WrongPolicy;
import org.omg.PortableServer.Servant;
import javax.annotation.Nonnull;
import java.util.Properties;
/**
* {@code Narrow} simplifies ORB object narrowing.
*
* @author B. K. Oxley (binkley)
*/
public final class CORBAHelper
implements Runnable {
private static final Properties useJacorb = new Properties();
static {
useJacorb.setProperty("org.omg.CORBA.ORBClass", "org.jacorb.orb.ORB");
useJacorb.setProperty("org.omg.CORBA.ORBSingletonClass", "org.jacorb.orb.ORBSingleton");
}
private final ORB orb;
private final POA root;
private final NamingContextExt nameService;
public interface Narrower {
/**
* Narrows the given CORBA object reference.
*
* @param reference the object reference, never missing
*
* @return the narrowed object
*/
T narrow(@Nonnull final org.omg.CORBA.Object reference);
}
public static ORB jacorb(final String... args) {
return ORB.init(args, useJacorb);
}
/**
* Constructs a new {@code CORBAHelper} for the given orb.
*
* @param orb the CORBA ORB, never missing
*/
public CORBAHelper(@Nonnull final ORB orb) {
try {
this.orb = orb;
root = initial(orb, "RootPOA", POAHelper::narrow);
root.the_POAManager().activate();
nameService = initial(orb, "NameService", NamingContextExtHelper::narrow);
} catch (final AdapterInactive | InvalidName e) {
throw new Error("Bad CORBA", e);
}
}
/**
* Gets the orb.
*
* @return the ORB, never missing
*/
@Nonnull
public ORB orb() {
return orb;
}
/**
* Runs the ORB, blocking until it shuts down.
*
* @see ORB#run()
*/
@Override
public void run() {
orb.run();
}
/**
* Narrows an initial reference from the ORB by name.
*
* @param the object type
* @param orb the ORB, never missing
* @param name the object name, never missing
* @param narrower the narrowing function, never missing
*
* @return the narrowed object
*
* @throws InvalidName if nae is not found by the ORB
*/
public static T initial(@Nonnull final ORB orb, @Nonnull final String name,
@Nonnull final Narrower narrower)
throws InvalidName {
return narrower.narrow(orb.resolve_initial_references(name));
}
/**
* Narrows a servant reference from the POA with the given implementation.
*
* @param the object type
* @param the servant type
* @param implementation the object implementation, never missing
* @param narrower the narrowing function, never missing
*
* @return the narrowed object
*
* @throws ServantNotActive if the servant is inactive
* @throws WrongPolicy TODO what is WrongPolicy
*/
public T servant(@Nonnull final S implementation,
@Nonnull final Narrower narrower)
throws ServantNotActive, WrongPolicy {
return narrower.narrow(root.servant_to_reference(implementation));
}
/**
* Rebinds the given implementation object to name.
*
* @param the object type
* @param the servant type
* @param name the rebound name, never missing
* @param implementation the object implementaiton, never missing
* @param narrower the narrowing function, never missing
*
* @throws org.omg.CosNaming.NamingContextPackage.InvalidName if name is invalid
* @throws NotFound TODO what is not NotFound
* @throws CannotProceed TODO what is CannotProceed
* @throws ServantNotActive if the servant is inactive
* @throws WrongPolicy TODO what is WrongPolicy
*/
public void rebind(
@Nonnull final String name, @Nonnull final S implementation,
@Nonnull final Narrower narrower)
throws org.omg.CosNaming.NamingContextPackage.InvalidName, NotFound, CannotProceed,
ServantNotActive, WrongPolicy {
nameService.rebind(nameService.to_name(name), servant(implementation, narrower));
}
/**
* Resolves the given name to an object.
*
* @param the object type
* @param name the object name, never missing
* @param narrower the narrowing function, never missing
*
* @return the resolved object
*
* @throws org.omg.CosNaming.NamingContextPackage.InvalidName if name is invalid
* @throws CannotProceed TODO what is CannotProceed
* @throws NotFound if name is not bound
*/
public T resolve(@Nonnull final String name, @Nonnull final Narrower narrower)
throws CannotProceed, org.omg.CosNaming.NamingContextPackage.InvalidName, NotFound {
return narrower.narrow(nameService.resolve_str(name));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy