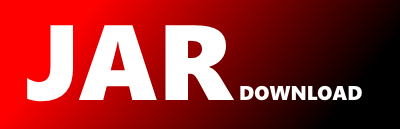
hm.binkley.util.logging.osi.SupportLoggers Maven / Gradle / Ivy
/*
* This is free and unencumbered software released into the public domain.
*
* Please see https://github.com/binkley/binkley/blob/master/LICENSE.md.
*/
package hm.binkley.util.logging.osi;
import ch.qos.logback.classic.Level;
import hm.binkley.util.logging.MarkedLogger;
import hm.binkley.util.logging.MinimumLogger;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.slf4j.ext.XLogger;
import org.slf4j.ext.XLoggerFactory;
import javax.annotation.Nonnull;
import static ch.qos.logback.classic.Level.ALL;
import static ch.qos.logback.classic.Level.INFO;
import static ch.qos.logback.classic.Level.WARN;
import static org.slf4j.MarkerFactory.getMarker;
/**
* {@code SupportLoggers} are custom {@link MarkedLogger}s for specialized use. Methods create new
* marked loggers with the enum names as markers. There is one factory method variant for each
* style of logger creation:
- {@link #getLogger(Class)}
- Creates a new underlying
* logger from a class
- {@link #getLogger(String)}
- Creates a new underlying logger
* from a logger name
- {@link #getLogger(Logger)}
- Reuses an existing underlying
* logger
Applications should configure logback or other logging system by marker:
*
- {@link #ALERT}
- Send alerts for system issues
- {@link #APPLICATION}
* - Logs normally following logback configuration
- {@link #AUDIT}
- Records an
* audit trail, typically to a database
- {@link #TRACE}
- A tracing logger for method
* entry and exit
*
* @author B. K. Oxley (binkley)
*/
public enum SupportLoggers {
/**
* Marks "ALERT" loggers for system issues. Rejects logging at less than {@code WARN} level
* (throws {@code IllegalStateException}).
*/
ALERT(WARN),
/** Unmarked loggers for normal logging. */
APPLICATION(ALL) {
@Nonnull
@Override
public Logger getLogger(@Nonnull final Class> logger) {
return LoggerFactory.getLogger(logger);
}
@Nonnull
@Override
public Logger getLogger(@Nonnull final String logger) {
return LoggerFactory.getLogger(logger);
}
@Nonnull
@Override
public Logger getLogger(@Nonnull final Logger logger) {
return logger;
}
},
/**
* Marks "AUDIT" loggers to record an audit trail, typically to a database. Rejects logging
* less than {@code INFO} level (throws {@code IllegalStateException}).
*/
AUDIT(INFO),
/** Trace loggers ({@link XLogger} for debugging. */
TRACE(ALL) {
@Nonnull
@Override
public XLogger getLogger(@Nonnull final Class> logger) {
return XLoggerFactory.getXLogger(logger);
}
@Nonnull
@Override
public XLogger getLogger(@Nonnull final String logger) {
return XLoggerFactory.getXLogger(logger);
}
@Nonnull
@Override
public XLogger getLogger(@Nonnull final Logger logger) {
return (XLogger) logger;
}
};
@Nonnull
private final Level minimum;
SupportLoggers(@Nonnull final Level minimum) {
this.minimum = minimum;
}
/**
* Redundant method for {@link #TRACE} returning {@code XLogger}.
*
* @param logger the logger class, never missing
*
* @return the XLogger, never missing
*
* @todo Java needs anonymous instance covariant returns to avoid casting
*/
@Nonnull
public static XLogger trace(@Nonnull final Class> logger) {
return trace(logger.getName());
}
/**
* Redundant method for {@link #TRACE} returning {@code XLogger}.
*
* @param logger the logger name, never missing
*
* @return the XLogger, never missing
*
* @todo Java needs anonymous instance covariant returns to avoid casting
*/
@Nonnull
public static XLogger trace(@Nonnull final String logger) {
return (XLogger) TRACE.getLogger(logger);
}
/**
* Creates a new marked logger for the given logger.
*
* @param logger the underlying class, never missing
*
* @return the wrapping marked logger, never missing
*/
@Nonnull
public Logger getLogger(@Nonnull final Class> logger) {
return getLogger(LoggerFactory.getLogger(logger));
}
/**
* Creates a new marked logger for the given logger.
*
* @param logger the underlying logger name, never missing
*
* @return the wrapping marked logger, never missing
*/
@Nonnull
public Logger getLogger(@Nonnull final String logger) {
return getLogger(LoggerFactory.getLogger(logger));
}
/**
* Creates a new marked logger for the given logger.
*
* @param logger the underlying SLF4j logger, never missing
*
* @return the wrapping marked logger, never missing
*/
@Nonnull
public Logger getLogger(@Nonnull final Logger logger) {
return ALL == minimum ? new MarkedLogger(logger, getMarker(name())) : new MinimumLogger(
new MarkedLogger(logger, MinimumLogger.class.getName(), getMarker(name())),
minimum);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy