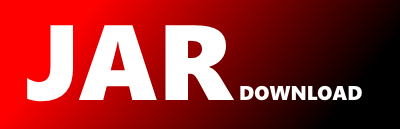
hm.binkley.util.Mixin Maven / Gradle / Ivy
/*
* This is free and unencumbered software released into the public domain.
*
* Please see https://github.com/binkley/binkley/blob/master/LICENSE.md.
*/
package hm.binkley.util;
import javax.annotation.Nonnull;
import java.util.List;
import static java.lang.reflect.Proxy.newProxyInstance;
/**
* {@code Mixin} implements concrete class mixins via JDK proxies. Proxy
* handler strategy tries: - Previously matched methods
- Exact
* static match by enclosing type
- "Duck" matches by name and parameter
* type
Mixins expose a single, composite interface of all
* supported interfaces.
*
* Mixins may optionally include {@code Mixin} as port of the composite
* interface. This exposes {@link #mixinDelegates()} providing public
* access to the mixed in object delegated to by this proxy in the same order as
* method lookup considers delegates.
*
* @author B. K. Oxley (binkley)
* @see #newMixin(Class, Object...) Create a new mixin
*/
public interface Mixin {
/**
* Creates a new mixin. If as extends {@code Mixin}, provides a
* supporting {@link #mixinDelegates()} method giving public access to
* delegates.
*
* @param as a superinterface of visible public methods implemented by
* delegates, never missing
* @param delegates the mixed-in delegates (implementations)
* @param the mixin proxy type (type of as)
*
* @return the mixed-in proxy, never missing
*/
@Nonnull
static T newMixin(@Nonnull final Class as,
final Object... delegates) {
return as.cast(newProxyInstance(as.getClassLoader(), new Class[]{as},
new MixinHandler<>(as,
new MixedDelegates(delegates).mixinDelegates())));
}
/**
* Gets an unmodifiable list of delegates implementing the mixin in the same
* order as method lookup.
*
* @return the unmodifiable list of delegates, never missing
*/
@Nonnull
List