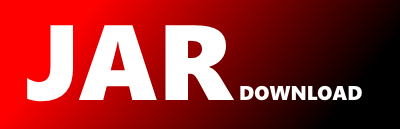
hr.fer.tel.markdown.MdToHtmlConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of markdown-to-html Show documentation
Show all versions of markdown-to-html Show documentation
Library for converting markdown with code and plantuml to local html page.
package hr.fer.tel.markdown;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.Reader;
import java.io.StringReader;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.List;
import com.vladsch.flexmark.html.HtmlRenderer;
import com.vladsch.flexmark.parser.Parser;
import com.vladsch.flexmark.util.ast.Node;
import com.vladsch.flexmark.util.data.MutableDataSet;
public class MdToHtmlConverter {
private Reader markdownReader;
public MdToHtmlConverter(Path markdownPath) throws IOException {
this(Files.newBufferedReader(markdownPath, StandardCharsets.UTF_8));
}
public MdToHtmlConverter(String markdownContent) {
this(new StringReader(markdownContent));
}
public MdToHtmlConverter(Reader markdownReader) {
this.markdownReader = markdownReader;
}
public String convert() throws IOException {
MutableDataSet options = new MutableDataSet();
options.set(Parser.EXTENSIONS, List.of(PlantUmlRendererOptions.PlantUmlExtension.create()));
Parser parser = Parser.builder(options).build();
HtmlRenderer renderer = HtmlRenderer.builder(options).build();
Node document = parser.parseReader(markdownReader);
String html = renderer.render(document);
StringBuilder sb = new StringBuilder();
sb.append(createPrefix());
sb.append(html.replaceAll("", ""));
sb.append(createSuffix());
return sb.toString();
}
private String createSuffix() {
return createJsLinks() + """